Question
For my program below that is in C++ I have the different parts below that I have to make sure my code has completed or
For my program below that is in C++ I have the different parts below that I have to make sure my code has completed or needs to include. Also I need to implement in my code that has the capability of measuring the actual sorting time (https://stackoverflow.com/questions/5248915/execution-time-of-c-program) heres a reference link I was given. I also attached a screenshot of the input files that I am suppose to measure the running time but im just only including for you to see what I am suppose to measure.
a) my program is well commented
b) The program reads input from a data file specified by user and write the sorted output into another file;
c) Design your program in the way that it can accept users options such as:
*. Which sort algorithm to use;
*. Which sort order to use, that is, non-decreasing order or non-increasing order?
*. Whether should the output remove duplications?
d) Also I need to implement in my code that has the capability of measuring the actual sorting time (https://stackoverflow.com/questions/5248915/execution-time-of-c-program) heres a reference link I was given.
#include
#include
#include
#include
#include
using namespace std;
void insertionsort(int array[],int n){
int i, key, j;
for (i= 1; i
key= array[i];
j=i-1;
while (j >= 0 && array[j]>key){
array[j+1] = array[j];
j = j-1;
}
array[j+1] = key;
}
ofstream out("inputFile.txt");
for(i=0;i out cout out.close(); } void merge(int arr[], int l, int m, int r){ int i, j, k; int n1 = m - l + 1; int n2 = r - m; int L[n1], R[n2]; for (i = 0; i L[i] = arr[l + i]; } for (j = 0; j R[j] = arr[m + 1+ j]; i = 0; j = 0; k = l; } while (i if (L[i] arr[k] = L[i]; i++; } else{ arr[k] = R[j]; j++; } k++; } while (i arr[k] = L[i]; i++; k++; } while (j arr[k] = R[j]; j++; k++; } } void mergeSort(int arr[], int l, int r){ if (l int m = l+(r-l)/2; mergeSort(arr, l, m); mergeSort(arr, m+1, r); merge(arr, l, m, r); } } void swap(int *a, int *b){ int x = *a; *a = *b; *b = x; } int partition (int arr[], int low, int high){ int pivot = arr[high]; int i = (low - 1); for (int j = low; j if (arr[j] i++; swap(&arr[i], &arr[j]); } } swap(&arr[i + 1], &arr[high]); return (i + 1); } void quickSort(int arr[], int low, int high){ if (low int z = partition(arr, low, high); quickSort(arr, low, z - 1); quickSort(arr, z + 1, high); } } int removeDuplicates(int array[], int n){ if (n==0 || n==1) return n; int j = 0; for (int i=0; i if (array[i] != array[i+1]) array[j++] = array[i]; array[j++] = array[n-1]; return j; } //Sorting the array void countingSort(int *input, size_t num_elements) { size_t max = *std::max_element(input, input+num_elements); std::vector for (int i=0; i ++counts[input[i]]; int temp = input[0]; input[0] = input[num_elements-1]; int ntemp = input[num_elements-2]; input[num_elements-2] = temp; input[num_elements-1] = ntemp; } int main(int argc,char *argv[]){ int array[],i=0,j,count=0,k; ifstream input; input.open(argv[1]); if(input.fail()) { cout } else{ while(input.eof()==0){ input>>array[i]; i=i+1; count=count+1; } string s; cout cin >> s; if(s==std::string("insert")){ insertionsort(array,count); ofstream out("inputFile.txt"); for(i=0;i out } cout out.close(); } else if(s==std::string("quick")){ quickSort(array,0,count-1); ofstream out("inputFile.txt"); for(i=0;i out } cout out.close(); } else if(s==std::string("merge")){ mergeSort(array,0,count); ofstream out("inputFile.txt"); for(i=0;i out cout out.close(); } else if(s==std::string("count")){ countingSort(array, count); ofstream out("inputFile.txt"); for(i=0;i out cout } cout out.close(); } else{ cout }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
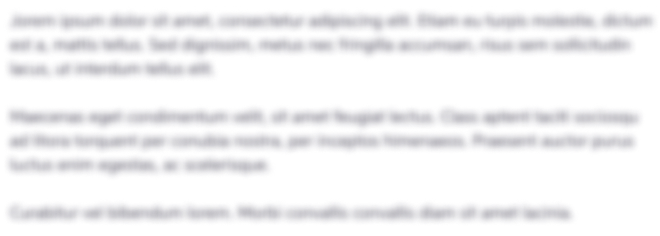
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started