Question
For question 1 I'm not sure what to put in the header.h file and the main.c file. Please copy-and-paste the following files (0 Points): insertionSort.c
For question 1 I'm not sure what to put in the header.h file and the main.c file.
Please copy-and-paste the following files (0 Points):
insertionSort.c
/*-------------------------------------------------------------------------* *--- ---* *--- insertionSort.c ---* *--- ---* *--- This file defines a function that implements the insertion- ---* *--- sort algorithm. ---* *--- ---* *--- ---- ---- ---- ---- ---- ---- ---- ---- ---* *--- ---* *--- Version 1a 2018 April 2 Joseph Phillips ---* *--- ---* *-------------------------------------------------------------------------*/ #include "header.h" // PURPOSE: To sort the 'arrayLen' strings in array 'array' with the // insertion-sort algorithm. No return value. void insertionSort (char** array, int arrayLen ) { int i; int j; for (i = 0; i < arrayLen-1; i++) for (j = i+1; j < arrayLen; j++) if ( strncmp(array[i],array[j],strLen) > 0 ) swap(array,i,j); }
quickSort.c
/*-------------------------------------------------------------------------* *--- ---* *--- quickSort.c ---* *--- ---* *--- This file defines a function that implements the quick-sort ---* *--- algorithm. ---* *--- ---* *--- ---- ---- ---- ---- ---- ---- ---- ---- ---* *--- ---* *--- Version 1b 2018 April 2 Joseph Phillips ---* *--- ---* *-------------------------------------------------------------------------*/ #include "header.h" int partition (char** array, char* pivot, int lo, int hi ) { lo--; hi++; while (1) { do { lo++; } while (strncmp(array[lo],pivot,strLen) < 0); do { hi--; } while (strncmp(array[hi],pivot,strLen) > 0); if (lo >= hi) break; swap(array,lo,hi); } return(hi); } int pivot (char** array, int lo, int hi ) { char* atLo = array[lo]; char* atMid = array[(lo+hi)/2]; char* atHi = array[hi]; if ( ((strncmp(atLo,atMid,strLen)<=0) && (strncmp(atMid,atHi,strLen)<=0)) || ((strncmp(atLo,atMid,strLen)>=0) && (strncmp(atMid,atHi,strLen)>=0)) ) return((lo+hi)/2); if ( ((strncmp(atMid,atLo,strLen)<=0) && (strncmp(atLo,atHi,strLen)<=0)) || ((strncmp(atMid,atLo,strLen)>=0) && (strncmp(atLo,atHi,strLen)>=0)) ) return(lo); return(hi); } void quickSort_ (char** array, int lo, int hi ) { if (lo < hi) { int left; int right; int p = pivot(array,lo,hi); p = partition(array,array[p],lo,hi); quickSort_(array,lo,p); quickSort_(array,p+1,hi); } } // PURPOSE: To sort the 'arrayLen' strings in array 'array' with the // quick-sort algorithm. No return value. void quickSort (char** array, int arrayLen ) { quickSort_(array,0,arrayLen-1); }
1. C programming (20 Points):
These two files need a main() to run their functions insertionSort() and quickSort(). Then all three C files need a header file to inform them of what the others have that they need. Please finish both the main() and header.h.
Please note! Not everything needs to be shared.
main() needs insertionSort() and quickSort()
Both insertionSort() and quickSort() need swap() and strLen.
Otherwise, it is best not to share too much, kind of like keeping methods and members private in C++ and Java.
header.h
/*-------------------------------------------------------------------------* *--- ---* *--- header.h ---* *--- ---* *--- ---- ---- ---- ---- ---- ---- ---- ---- ---* *--- ---* *--- Version 1a 2018 April 2 Joseph Phillips ---* *--- ---* *-------------------------------------------------------------------------*/ #include #include #include // YOUR CODE HERE
main.c
/*-------------------------------------------------------------------------* *--- ---* *--- main.c ---* *--- ---* *--- This file defines the variable strLen and function swap() ---* *--- needed for the program of assignment 1. ---* *--- ---* *--- ---- ---- ---- ---- ---- ---- ---- ---- ---* *--- ---* *--- Version 1a 2018 April 2 Joseph Phillips ---* *--- ---* *-------------------------------------------------------------------------*/ #include "header.h" #define TEXT_LEN 256 // PURPOSE: To tell the length of the strings to sort. int strLen; // PURPOSE: To swap the strings in 'array[]' at indices 'index0' and 'index1'. // No return value. void swap (char** array, int index0, int index1 ) { // YOUR CODE HERE } // PURPOSE: To repeatedly ask the user the text "Please enter ", followed // by the text in 'descriptionCPtr', followed by the numbers 'min' and // 'max', and to get an entered integer from the user. If this entered // integer is either less than 'min', or is greater than 'max', then // the user is asked for another number. After the user finally enters // a legal number, this function returns that number. int obtainIntInRange(const char* descriptionCPtr, int min, int max ) { int entry; char text[TEXT_LEN]; // YOUR CODE HERE return(entry); } // PURPOSE: To generate the array of strings. char** generateStringArray (int numStrings ) { char** array = (char**)calloc(numStrings,sizeof(char*)); int i; int j; for (i = 0; i < numStrings; i++) { array[i] = (char*)malloc(strLen); for (j = 0; j < strLen; j++) { array[i][j] = (rand() % 16) + 'A'; } } return(array); } void print (char** array, int arrayLen ) { int i; int j; for (i = 0; i < arrayLen; i++) { for (j = 0; j < strLen; j++) { putchar(array[i][j]); } putchar(' '); } } void releaseMem (char** array, int arrayLen ) { int i; for (i = 0; i < arrayLen; i++) { free(array[i]); } free(array); } int main () { int arrayLen; char** array; int choice; arrayLen = obtainIntInRange("number of strings",1,65536*16); strLen = obtainIntInRange("length of each string",1,16); choice = obtainIntInRange("1 for insertion sort or 2 for quicksort",1,2); array = generateStringArray(arrayLen); switch (choice) { case 1 : insertionSort(array,arrayLen); break; case 2 : quickSort(array,arrayLen); break; } print(array,arrayLen); releaseMem(array,arrayLen); return(EXIT_SUCCESS); }
Sample Initial Output:
$ ./assign1 Please enter number of strings (1-1048576): 0 Please enter number of strings (1-1048576): 200000000 Please enter number of strings (1-1048576): 32 Please enter length of each string (1-16): 3 Please enter 1 for insertion sort or 2 for quicksort (1-2): 1 BID BMH BNM CEB CLD CNH DBP DDP EBO EIL FHI GKO GLE GMC HAJ HGJ HKM IHN ILM IMK JKG JOE KFN KMJ LCN LCP LGP MAL MEM NKL OBB OBO
Step by Step Solution
There are 3 Steps involved in it
Step: 1
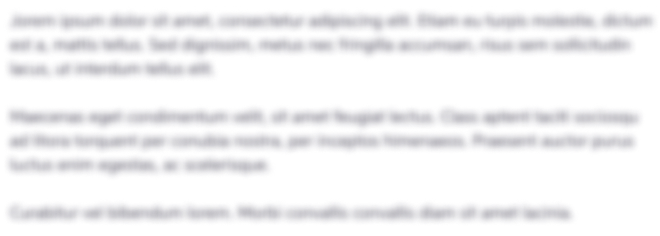
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started