Question
For the fourth assignment, you will modify the Tip Calculator app in Section 12.5 to allow the user to enter the number of people in
For the fourth assignment, you will modify the Tip Calculator app in Section 12.5 to allow the user to enter the number of people in the party. Calculate and display the amount owed by each person if the bill were to be split evenly among the party members.
Provided code
tip.java ***********************************
import javafx.application.Application; import javafx.fxml.FXMLLoader; import javafx.scene.Parent; import javafx.scene.Scene; import javafx.stage.Stage;
public class tip extends Application {
@Override
public void start(Stage stage) throws Exception {
Parent root = FXMLLoader.load(getClass().getResource("Tipcalculator.fxml")); Scene scene = new Scene(root); stage.setScene(scene); stage.setTitle("Tip Calculator"); // Displayed in window's title bar stage.show(); // Display the stage }
public static void main(String[] args) { launch(args); } }
Tipcalc.java *******************************
import java.math.BigDecimal; import java.math.RoundingMode; import java.text.NumberFormat; import javafx.beans.value.ChangeListener; import javafx.beans.value.ObservableValue; import javafx.event.ActionEvent; import javafx.fxml.FXML; import javafx.scene.control.Label; import javafx.scene.control.Slider; import javafx.scene.control.TextField;
public class Tipcalcc {
private static final NumberFormat currency = NumberFormat.getCurrencyInstance();
private static final NumberFormat percent = NumberFormat.getPercentInstance();
private BigDecimal tipPercentage = new BigDecimal(0.15); // 15% default
@FXML private TextField amountTextField;
@FXML private Label tipPercentageLabel;
@FXML private Slider tipPercentageSlider;
@FXML private TextField tipTextField;
@FXML private TextField totalTextField;
@FXML private void calculateButtonPressed(ActionEvent event) {
try {
BigDecimal amount = new BigDecimal(amountTextField.getText()); BigDecimal tip = amount.multiply(tipPercentage); BigDecimal total = amount.add(tip); tipTextField.setText(currency.format(tip)); totalTextField.setText(currency.format(total));
} catch (NumberFormatException ex) {
amountTextField.setText("Enter amount"); amountTextField.selectAll(); amountTextField.requestFocus();
} }
public void initialize() {
currency.setRoundingMode(RoundingMode.HALF_UP); tipPercentageSlider.valueProperty().addListener(new ChangeListener
}
});
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
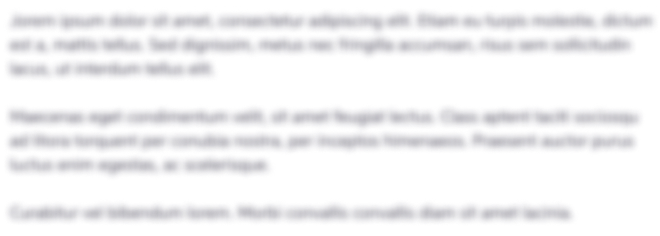
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started