Question
For this assignment, one complete program and one partial program are being provided to you. The PlayingCard program is good as it is. The CardDeal
For this assignment, one complete program and one partial program are being provided to you. The PlayingCard program is good as it is. The CardDeal program is like a tester program and you will need make modifications to it.
CardDeal methods:
populateCardDeck() - this method populates an ArrayList with 52 PlayingCard objects (one for each suit/value pair) you find in a deck of playing cards.
outputCardDeck() - the method call is there and the method heading shell is there. Modify this method to output all the PlayingCards which are in the deck ArrayList.
dealHand() - the method call is there and the method heading shell is there. if you look at the hand array in the program, you will see it's set up to accomodate 5 PlayingCard objects. This method is to populate that hand array with 5 distinct PlayingCard objects. It also needs to manage the deck ArrayList of PlayingCard to ensure the same card isn't dealt more than once. Meaning as a PlayingCard is dealt to the hand array it is removed from the deck ArrayList.
showHand() - the method call is there and the method heading shell is there. Modify this method to output the hand array content of 5 PlayingCards.
showRemainingDeck() - the method call is there and the method heading shell is there. Modify this method to output all the remaining PlayingCards which are in the deck ArrayList. This should be one line of code that simply calls one of the other methods.
***** I do not know how to attach a file to the question so I am pasting below
PlayingCard.java
/** This program is for representing one Card from a fifty-two card deck of playing cards. */ public class PlayingCard { private String suit; private String value; public PlayingCard() { //default no argument constructor for creating a new instance }
public PlayingCard(String pSuit, String pValue) { //overloaded constructor to initialize with passes values suit = pSuit; value = pValue; } public String getSuit() { return suit; } public void setSuit(String pSuit) { suit = pSuit; } public String getValue() { return value; } public void setValue(String pValue) { value = pValue; } public String toString() { StringBuffer buf = new StringBuffer(); buf.append("Card: " + getValue() + " of " + getSuit()); return buf.toString(); } public boolean equals(Object obj) { PlayingCard oth; boolean result = false; if (obj instanceof PlayingCard) { oth = (PlayingCard) obj; if (this.getSuit().equals(oth.getSuit()) && this.getValue().equals(oth.getValue())) { result = true; //if all the preceding are true this PlayingCard and oth are equivalent } } return result; } }
CardDeal.java
import java.util.ArrayList;
public class CardDeal { static String[] values = {"Ace", "2", "3", "4", "5", "6", "7", "8", "9", "10", "Jack", "Queen", "King"}; static String[] suits = {"Hearts", "Diamonds", "Spades", "Clubs"}; static ArrayList
static PlayingCard[] hand = new PlayingCard[5]; public static void main(String[] args) { populateCardDeck(); outputCardDeck(); dealHand(); showHand(); showRemainingDeck(); } public static void populateCardDeck() { //populate the deck with PlayingCards for(int s = 0; s < suits.length; s++) { for(int v = 0; v < values.length; v++) { PlayingCard card = new PlayingCard(suits[s], values[v]); deck.add(card); } } }
public static void outputCardDeck() { }
public static void dealHand() { } public static void showHand() { }
public static void showRemainingDeck() { } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
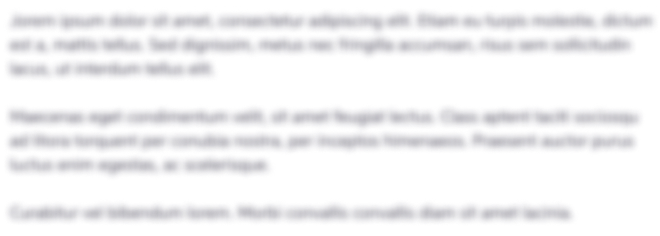
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started