Question
For this assignment we will begin working on the Library Inventory System. It is a piece of software that keeps tracks of books in a
For this assignment we will begin working on the "Library Inventory System." It is a piece of software that keeps tracks of books in a library's inventory. Including the ability to check-in/check-out books as well as add and remove books from the system. We will be building on this system throughout the semester.
Your software needs to do the following:
1.Load the inventory from and save the inventory to a file.
2.Keep track of the author and title of each book, as well as an ID number for each book.
3.Be able to check in and out books in the inventory based on the book's ID number.
4.Be able to add and remove books to/from the inventory based on the book's ID number.
You will have some freedom in how you implement the code, but it must meet those requirements.
I have provided the source.cpp and library.h files from my solution. Your job is to write the definitions in library.cpp for the function in library.h. A couple of the functions are overloaded. You can choose to use the overload or not.
Quitting should save the inventory to the same file it was loaded from.
Bonus 50XP: cin can't deal with spaces very easily, so it is best to separate words with underscores for the title and author. Learn how to use getline and use that instead!
Bonus 140XP: Keep the array sorted by ID number. Create a binary search function and use that to find books!
Please focus on Bonus.
here is the source.cpp file given to me
/* | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
Bonus: Keep array sorted and use binary search! | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
Bonus: getline | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
*/ | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
#include | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
#include | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
#include "library.h" | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
using namespace std; | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
int displayMainMenu(); | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
int main() | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
{ | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
Book inventory[MAX_BOOKS]; | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
int currID = 0; | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
int numBooks = 0; | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
string fileName = "libraryInventory.txt"; | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
if (!loadBooks(fileName, inventory, numBooks, currID)) | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
{ | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
cout << "No current inventory saved! Using empty inventory! "; | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
system("PAUSE"); | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
} | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
enum class MainMenu { add = 1, remove, checkOut, checkIn, save, quit, NA }; | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
MainMenu choice = MainMenu::NA; | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
while (choice != MainMenu::quit) | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
{ | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
system("CLS"); | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
choice = (MainMenu)displayMainMenu(); | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
system("CLS"); | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
switch (choice) | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
{ | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
case MainMenu::add: | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
{ | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
addNewBook(currID, inventory, numBooks); | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
break; | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
} | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
case MainMenu::remove: | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
removeBook(inventory, numBooks); | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
break; | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
case MainMenu::checkOut: | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
checkOut(inventory, numBooks); | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
break; | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
case MainMenu::checkIn: | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
checkIn(inventory, numBooks); | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
break; | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
case MainMenu::quit: | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
cout << "Thank you for using the EIT Library System "; | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
case MainMenu::save: | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
if (saveBooks(fileName, inventory, numBooks, currID)) | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
{ | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
cout << "Inventory successfully saved to disk! "; | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
} | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
else | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
{ | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
cout << "Error! Inventory could not be saved! All new data may be lost! "; | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
} | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
system("PAUSE"); | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
break; | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
default: | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
cout << "Please enter a valid option! "; | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
system("PAUSE"); | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
break; | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
} | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
} | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
return 0; | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
} | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
int displayMainMenu() | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
{ | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
int choice = 0; | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
while (choice < 1 || choice > 6) | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
{ | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
cout << "Welcome to the EIT Library System do you want to: "; | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
cout << " 1. Add a book to inventory "; | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
cout << " 2. Remove a book from inventory "; | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
cout << " 3. Check out a book "; | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
cout << " 4. Check in a book "; | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
cout << " 5. Save Inenvtory "; | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
cout << " 6. Save Inenvtory & Quit "; | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
cout << "Please make a selection (1-6): "; | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
cin >> choice; | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
} | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
return (choice); | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
} And here is the library.h file
| |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
|
Step by Step Solution
There are 3 Steps involved in it
Step: 1
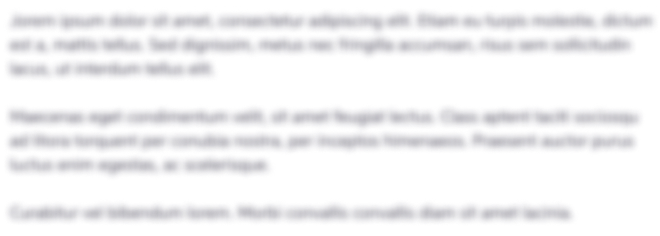
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started