Question
For this assignment, you are asked to use your GasTank, Engine, and Car classes from assignment 2 and create a GUI which presents the user
For this assignment, you are asked to use your GasTank, Engine, and Car classes from assignment 2 and create a GUI which presents the user with dialog windows for inputting information about a car and driving trip. Once all of the information about your car has been entered, your program must display a window which contains a description of the car. When that window is closed, your program must gather the data about the trip and then display a graphics panel on which is drawn a set of lines showing each leg of the trip, and text showing the end coordinates of each. You will need to read and learn about various GUI (awt and swing) classes, such as the Graphics class provided by Java, and this is intended to give you experience in learning API's.
GasTank.java
- Make a new project, with a copy of each of the classes from Assignment 2.
import java.lang.Math;
class GasTank
{
private int capacity;
private double curr_level;
GasTank(int cap)
{
if(cap<0)
{
this.capacity=0;
}
else
{
this.capacity=cap;
}
}
public int getCapacity()
{
return this.capacity;
}
public double getLevel()
{
return this.curr_level;
}
public void setLevel(double level)
{
if(level<0)
{
this.curr_level=0;
}
else if(level > capacity){
this.curr_level = capacity;
}
else
{
this.curr_level=level;
}
}
}
Engine.java
class Engine
{
private String description;
private int mpg;
private int max_speed;
Engine(String des, int mpg, int speed)
{
if(des.length()==0)
{
this.description="Generic Engine";
}
else
{
this.description=des;
}
if(mpg<0)
{
this.mpg=0;
}
else
{
this.mpg=mpg;
}
if(speed<0)
{
this.max_speed=0;
}
else
{
this.max_speed=speed;
}
}
public String getDescription()
{
return this.description + "(MPG: "+ this.mpg+ ", "+ "Max Speed: "+ this.max_speed+")";
}
public int get_mpg()
{
return this.mpg;
}
public int getMaxSpeed()
{
return this.max_speed;
}
}
Car.java
public class Car
{
private String description;
private int x;
private int y;
private GasTank tank;
private Engine eng;
Car(String desc, int cap, Engine e)
{
if( desc.length()<=0)
{
this.description="Generic car";
}
else
{
this.description=desc;
}
this.tank=new GasTank(cap);
if(e==null)
{
this.eng=new Engine("",0,0);
}
else
{
this.eng=e;
}
}
public String getDescription()
{
String out="";
out=out+this.description;
out=out+" (engine: ";
out=out+eng.getDescription();
out=out+"), ";
out=out+" fuel: ";
out=out+this.tank.getLevel()+"//"+this.tank.getCapacity();
out=out+", location: ("+this.x+", "+ this.y+")";
return out;
}
public int get_x()
{
return this.x;
}
public int get_y()
{
return this.y;
}
public double get_fuel_level()
{
return this.tank.getLevel();
}
public int get_mpg()
{
return this.eng.get_mpg();
}
public void fillUp()
{
double fill=this.tank.getCapacity();
this.tank.setLevel(fill);
}
public int get_max_speed()
{
return this.eng.getMaxSpeed();
}
public double drive(int distance, double xr, double yr)
{
double cos=xr/Math.pow((xr*xr+yr*yr),0.5);
double sin=yr/Math.pow((xr*xr+yr*yr),0.5);
double x_dist;
double y_dist;
double dist_possible=this.tank.getLevel()*this.eng.get_mpg();
if (dist_possible>=distance)
{
dist_possible=distance;
x_dist=dist_possible*cos;
y_dist=dist_possible*sin;
this.x=this.x+(int)x_dist;
this.y=this.y+(int)y_dist;
this.tank.setLevel(this.tank.getLevel() -distance/this.eng.get_mpg());
}
else
{
x_dist=dist_possible*cos;
y_dist=dist_possible*sin;
this.x=this.x+(int)x_dist;
this.y=this.y+(int)y_dist;
this.tank.setLevel(0);
System.out.println("Ran out after driving "+ dist_possible+" miles");
}
return dist_possible;
}
}
- Create a new class named Assignment3, which will first use a series of dialogs to prompt the user for all of the necessary information for a car (in the following order):
- Car description
- Fuel tank capacity
- Engine description
- Miles per gallon
- Max speed
- (Now a dialog displays all of the car's info, before the next series of input dialogs)
- The number of legs in the trip (you can assume that this number will never be greater than 10)
- (For each leg of the trip, the following three input dialogs appear, in this order, one leg at a time, and each dialog should show the number of the leg that it is collecting info for)
- The distance of the leg
- The x ratio for the direction of the leg
- The y ratio for the direction of the leg
- When reading in the values which are integers, you should use Integer.parseInt (google it for documentation about how it works) to get the integer value of the value entered by the user. Note that Integer.parseInt throws an exception if given something other than a valid number. If such an exception occurs, you must catch the exception and display a dialog saying "Invalid data entered. Exiting." and then the program should immediately exit. When reading in values for doubles, you should find the necessary function which is analogous to Integer.parseInt but used for doubles, and non-numerical input should be handled the same way.
- When any number other than the x ratio or y ratio are requested, if a negative number or zero is entered, your program should loop and keep redisplaying the same input dialog until a positive number is entered.
- A Car object should be created (along with the necessary GasTank and Engine objects) and all of the information about the car and legs of the trip should be used to calculate the location of the car and fuel level after each (just as in assignment 2). Before beginning the first leg of the trip, the car's tank should be filled, but it should not be filled again for subsequent legs. All aspects of the calculations which were required for the previous assignment are required for this assignment, so make sure your code works and correct any bugs that existed in your last assignment. You should not need to modify any of the classes from Assignment 2 as long as they were working correctly.
- Finally, a JFrame object should be created which contains an instance of a panel class that you create (which should be in DrivePanel.java and named DrivePanel and will extend JPanel similar to the example DrawPanel class in section 4.15 of the book). On the panel should be drawn line segments corresponding to each leg of the trip, with the assumption that the first leg begins at point (0,0) and that point is located at the bottom left corner. Keep in mind that graphics windows treat y=0 as the top of the window, with y-coordinates increasing downward, but we will assume (and draw) the opposite to be in line with traditional coordinate systems. Additionally, the location of the end of each leg should be written in text at the location 10 pixels to the right. (See the example-output1.jpg below for the output when a correctly working version is given the following series of input: TestCar, 50, V8, 50, 100, 3, 200, 2, 3, 300, -1, 4, 500, 2, -0.5. That is, a trip of the three legs (200, 2, 3), (300, -1, 4), (500, 2, -0.5). Additionally, see Assignment3-Example.pdf for pictures of the full set of windows in the correct sequence.)
- Your JFrame window must be created at size 600 x 600. To get full points for this assignment, your JFrame window should correctly show the lines and end coordinates in text even after being resized.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
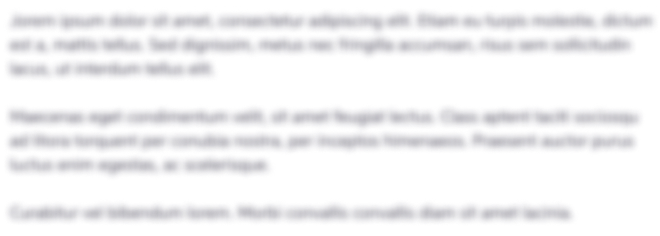
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started