Question
For this assignment, you must work individually. For each of the programming questions, create the code requested. Much of the code requires that you create
For this assignment, you must work individually. For each of the programming questions, create the code requested. Much of the code requires that you create a procedure that performs a specific task. In addition to creating the procedures, you will need to create code to test all the procedures you have written. For this assignment, you will create a class named Statistics that will calculate some basic statistics using an array passed as an argument. This class is similar to the MyMath class discussed in the lectures. To put the data in the array, you will generate psuedo random numbers. Finally, you will calculate some descriptive statistics and display the results to the user in the Console window.
Program Specifics
Create a new Java project in Eclipse.
Declare (create) a class named Statistics similar to the examples that I have presented in class. Create a second (main) class such that you have a static main method as shown in the demos.
In the main procedure, you will need to generate random numbers and store those values in an array of type double. Using the example presented class, create an instance of the Random class. The following statements create an instance of the Random class and sets the seed value: Random rnd = new Random(); long seed = 0; rnd.setSeed(seed); Note that you will need to import the Random class.
Using the same technique that you used before, display a prompt and read a value from the user. Convert that value to a double, if possible, or return an error value if the input cannot be converted. The input value must also be greater than zero. To perform the input validation, you must use theValidateInput class you created in the lab and demonstrated in class.
Declare an array such that it has the same number of elements as the number of elements specified by the user in the previous step. Suppose that the variable named elements contains an integer value. The following code segment shows how to declare an array named elementList: double[] elementList = new double[elements];
Write a loop to populate the array with random numbers. The following loop contains a template which will enumerate through the array elements. Note that elementList.length contains the number of elements in the array. All arrays have a length property containing the element count. int count; for (count = 0; count < elementList.length; count++ ) { // Statement(s) to get a random number rnd.nextInt() and store the value in the array. }
Create a static procedure named Minimum in the Statistics class. The function should return a double value. Your function should accept one argument - an array of type double. The function should return a double containing the minimum array value. The following statement shows the signature of the Minimum method (function). Your function must have exactly the same signature to get full credit. public static double Minimum(double[] inputList) And the following statement shows how to call the Minimum function using the examples already provided: double result; result = Minimum(elementList);
Create a static procedure named Maximum. The function should return a double value. Your function should accept ' one argument - an array of typedouble. The function should return a double containing the maximum array value.
Create a static procedure named Average. The function should return a double value. Return the average (mean) of all values in the array. Again, the function should accept an array as its argument.
Create a static procedure named StdDev. The function should return a double value. Return the standard deviation (population) of all the array elements. Again, the function should accept an array as its argument.
In main procedure, write the code to call the above procedures and display the results to the Console window.
Your program should produce the following output. First display the list of random numbers. Then display the min, max, average, and StdDev output values with an associated prompt.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
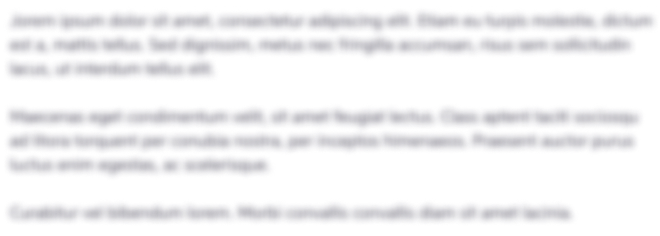
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started