Question
For this assignment you will be implementing the classic cellular automata of Conway's Game of Life. Rules A new cell is born on an empty
For this assignment you will be implementing the classic cellular automata of Conway's Game of Life. Rules
A new cell is born on an empty square if is surrounded by exactly three neighboring cells
A cell dies of overcrowding if it has four or more neighbors
A cell dies of loneliness if it has zero or one neighbor
Any live cell with two or three live neighbors lives, unchanged, to the next generation.
Implementation details:
The initial state of the game is held in a text file named the driver code and attached to this assignment (you must use this driver program). The format will be sequences of ones and zeros. A one means a live cell and zero a dead cell. You can determine the width of the grid by reading first line character by character until the end of the line terminator is encountered. You can determine the height of the grid by counting the lines of data.
Example:
00000000100000001010 00000000010000001001 11100000010100000010 10100100101010101010 00101010010010101000
The grader will use a file with arbitrary grade size (there is no limitation in terms of how large the grid would be) to grade the project. You algorithm should be able to handle this.
Example: Jeremy Allen's Glider Gun fight grid (set the iterations to 500).
Note: When printing out the status of the grid, print one character per cell in the grid to console. Use 0 to represent dead cell and one to represent live cell. The driver program will clear the screen between generations.
In this project you will have three files: 1) "life.h" stores the prototypes for all functions that are employed in the implementation of Game of Life 2) "life.cpp" stores the definitions of the functions that declared in "life.h" 3) "life_driver.cpp" the driver file that tests the functionality of your game of life.
Compilation and Running:
To compile the project in Linux type g++ life_driver.cpp life.cpp life.h o life in your terminal. Executable file life will be created as a result. You can run it by typing ./life
Please note that your project will be graded on eros.cs.txstate.edu and if your project does not compile with g++ compiler in that environment your project will get a grade of 0!
Provided files as they are should not produce any compilation errors.
Please note that you CANNOT modify file life_driver.cpp. You can only modify life.h and life.cpp file. It means that submitted life.h and life.cpp should work with default life_driver.cpp. file. Add additional functions, i.e., prototypes to life.h and corresponding function definitions to life.cpp, to make your code more readable. In this project you can also add global variables to allow passage of data structures between the functions in the life_driver.cpp. Those variables can be two dimensional pointers to store dynamic arrays or variables representing grid size etc. You can also create a temporary file that will store the state of your grid between the generations, i.e., between the calls to the iterateGeneration() function. You can use any reading/writing file operations you know.
Be sure to follow the style standards for the course.
Read grading criteria file for this project for more information.
Grading criteria for CS 2308
Grading criteria for CS 2308 Department of Computer Science, Texas State University-San Marcos |
Code compiles: 10 pts the code should be solving the problem related to the project, otherwise a grade of 0 will be assigned. The code will be compiled and graded with g++ compiler on eros.cs.txstate.edu If the code does not compile in that environment a grade of 0 will be assigned.
Following functionality is tested
ShowWorld() function works correctly: 5pts.
iterateGeneration() function works correctly, i.e., correct cells become alive and correct cells die: 30pts.
the program can handle input of an arbitrary size by using two dimensional dynamic arrays: 35pts.
Comments are present in the code: 5 pts.
Code styling margins (lines of the code are not too long), readability (code is not cluttered and follows style standards for the class ): 7 pts
Code efficiency: code is concise and no unnecessary conditional, interactive and sequential statements are employed considering the implementation requirements and constraints outlined by the project: 8 pts
Penalty points:
Non-default life_driver.cpp has to be employed for the program to work -30pts.
Dynamic arrays should grow and shrink with each generation as new live cells appear and disappear. Only single boundary of dead cells around the live cells is allowed when the new generation is displayed or written to a temporary file. In case of the glider-like configuration the displayed grid will remain visually stationary, i.e., in case of the static grid the glider would look like it is traveling somewhere, however in case of the dynamic grid because only single boundary of dead cells are allowed around the live cells the glider will seem as if it is stationary. If dynamic grid functionality is not implemented -10pts.
If string objects are used (i.e.,
If initially the implementation can read only grid up to a certain size, e.g., not more than 256 characters in length -30 pts
Extra grade:
1) Best project award +10pts.
2) First correct submission award, i.e., first submitted project that receives 100pts. will get an additional +10pts.
glider_gun_fight.txt
0000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000010000000000000000000000000000000000000000000 0000000000000000000000000000001010000000000000000000000000000000000000000000 0000000000000000000011000000110000000000001100000000000000000000000000000000 0000000000000000000100010000110000000000001100000000000000000000000110000000 0000000011000000001000001000110000000000000000000000000000000000000110000000 0000000011000000001000101100001010000000000000000000000000000000000000000000 0000000000000000001000001000000010000000000000000000000000000000000000000000 0000000000000000000100010000000000000000000000000000000000000000000000000000 0000000000000000000011000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000001110000000 0000000000000000000000000000000000000000000000000000000000000000010001000000 0000000000000000000000000000000000000000000000000000000000000000100000100000 0000000000000000000000000000000000000000000000000000000000000000100000100000 0000000000000000000000000000000000000000000000000000000000000000000100000000 0000000000000000000000000000000000000000000000000000000000000000010001000000 0000000000000000000000000000000000000000000000000000000000000000001110000000 0000011000000000000000111000000000000000000000000000000000000000000100000000 0000011000000000000000101000000000000000000000000000000000000000000000000000 0000000000000000000000110000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000011100000 0000000000000000000000000000000000000000000000000000000000000000000011100000 0000000000000000000000000000000000000000000000000000000000000000000100010000 0000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000001100011000 0000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000 0001100011000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000 0000100010000000000000000000000000000000000000000000000000000000000000000000 0000011100000000000000000000000000000000000000000000000000000000000000000000 0000011100000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000110000000000000000000000 0000000000000000000000000000000000000000000000000001010000000000000001100000 0000000010000000000000000000000000000000000000000001110000000000000001100000 0000000111000000000000000000000000000000000000000000000000000000000000000000 0000001000100000000000000000000000000000000000000000000000000000000000000000 0000000010000000000000000000000000000000000000000000000000000000000000000000 0000010000010000000000000000000000000000000000000000000000000000000000000000 0000010000010000000000000000000000000000000000000000000000000000000000000000 0000001000100000000000000000000000000000000000000000000000000000000000000000 0000000111000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000001100000000000000000000 0000000000000000000000000000000000000000000000000000100010000000000000000000 0000000000000000000000000000000000000000000100000001000001000000001100000000 0000000000000000000000000000000000000000000101000011010001000000001100000000 0000000110000000000000000000000011000000000000110001000001000000000000000000 0000000110000000000000000000000011000000000000110000100010000000000000000000 0000000000000000000000000000000000000000000000110000001100000000000000000000 0000000000000000000000000000000000000000000101000000000000000000000000000000 0000000000000000000000000000000000000000000100000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000
life.cpp
/**************************************************** Name: Date: Problem Number: CS2308 Instructor: Komogortsev, TSU *****************************************************/
//This file provides the implementation of the life.h header file.
#include
#include "life.h"
using namespace std;
//GLOBAL VARIABLES
int ROWS; //stores the number of rows in the grid int COLUMNS; //stores the number of rows in the grid
//This function reads input file for subsequent prosessing (add high level //description of your implementation logic) void populateWorld (const char * file) {
}
//This function outputs the grid for current generation (add high level //description of your implementation logic) void showWorld () {
}
//This function creats new geneneration grid from the old generation grid //(add high level description of your implementation logic) void iterateGeneration () {
}
life.h
/**************************************************** Name: Date: Problem Number: CS2308 Instructor: Komogortsev, TSU *****************************************************/
//This header file provides the prototypes of the function definitions //for the project.
#ifndef life_h #define life_h
#include
using namespace std;
void populateWorld(const char * file); void showWorld(); void iterateGeneration();
#endif
life_driver.cpp
/**************************************************** Name: Date: Problem Number: CS2308 Instructor: Komogortsev, TSU *****************************************************/
// This is driver's code
#ifdef linux #define LINUX true #define WINDOWS false #endif
#ifdef _WIN32 #define LINUX false #define WINDOWS true #endif
#include
#include "life.h"
const char FILE_NAME[] = "glider_gun_fight.txt";
using namespace std;
const int NUM_GENERATIONS = 10; //set to a smaller number for debugging
int main() { populateWorld(FILE_NAME);
showWorld();
for (int iteration = 0; iteration < NUM_GENERATIONS; iteration++) {
if (WINDOWS) system("cmd.exe /c cls"); //Windows only else system("clear"); //Linux only iterateGeneration(); showWorld(); } if (WINDOWS) system("cmd.exe /c PAUSE"); return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
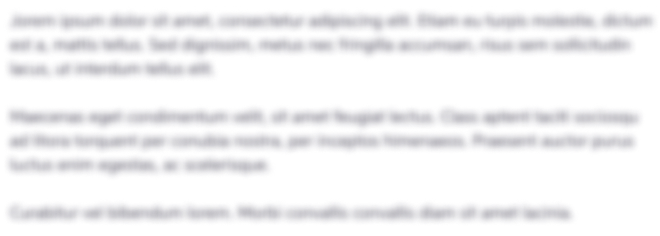
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started