Question
For this assignment you will be updating the Dashboard.java class from Assignment 1. Feel free to use your solution for Dashboard.java from Assignment 1 as
For this assignment you will be updating the Dashboard.java class from Assignment 1. Feel free to use your solution for Dashboard.java from Assignment 1 as a starting point for this assignment. Be sure to make a copy of your version of Dashboard.java so you dont lose your original dashboard file.
Now we want to be able to compare Dashboard Objects. We will do this by implementing the Comparable Interface. Youll need to implement the interface, which includes adding this method:
int compareTo(Object other) - A Dashboard object should be considered less than another if it has lower total mileage, or lower speed, or does not have a check engine light on, in that order of priority. This method should return -1 if the dashboard being checked is less than the other, 0 if they are the same, and 1 if the one being checked is greater than the other.
Additionally, well define a few other methods:
String race(Dashboard other, int acc1, int acc2) - This method should simulate a race between this dashboard and another dashboard called "other". Each dashboard will accelerate in increments of 1 mph n1 and n2 times, respectively (i.e. dashboard 1 will accelerate() acc1 times, and dashboard 2 ("other") will accelerate() acc2 times). If the first ("this") dashboard stalls out (exceeds 100 mph at any point), the method should return First car stalled out!. If the second ("other") dashboard stalls out at any point, the method should return Second car stalled out!. Then, the method should return the status of both dashboards and the result of the race. If one dashboard stalls, the one that did not should be declared the winner. If neither dashboard stalls, you can assume the winner is the dashboard with the higher final speed. If both dashboards stalled or neither stalls but they have the same speed, the result should be "Its a tie!" See the sample run of the student runner file for the proper return formatting.
String difference(Dashboard other) - This method should calculate how long the dashboard with fewer miles would need to drive at its current speed to have the same number of miles on its odometer as the dashboard with more miles. For the output, the dashboard that this method is being called on should be labeled as "first car", and the "other" dashboard should be labeled "second car". The method should return a string formatted as follows:
Second car will need to drive for 60 minutes to catch first car.
Or:
First car will need to drive for 60 minutes to catch second car.
Final Notes
See the sample run of the student runner file below for examples of the expected output format.
Remember, all variables should have an access level of private and all required methods should have an access level of public.
Please download the runner class, Student_Runner_Dashboard.java (Links to an external site.)Links to an external site. into the same directory as your Dashboard.java file, run the file in DrJava, and verify that the class output matches the Sample Run that follows. We will use a different runner to grade the program. Remember to change the runner to test different values to make sure your program fits the requirements.
When you are ready, paste your entire Dashboard.java class in the box below, click run to test the output, and click submit when you are satisfied with your results.
Sample run of Student_Runner_Dashboard.java:
Printing Dashboard: Speedometer: 50 MPH Odometer: 01250 Check Engine: Off Comparing two dashboards: -1 1 0 -1 Testing race: Race 1: Car 1 stalls First car stalled out! Speedometer: 0 MPH Odometer: 01250 Check Engine: On Speedometer: 80 MPH Odometer: 01250 Check Engine: Off Car 2 has won the race! Race 2: Both cars stall First car stalled out! Second car stalled out! Speedometer: 0 MPH Odometer: 01250 Check Engine: On Speedometer: 0 MPH Odometer: 01250 Check Engine: On It's a tie! Race 3: Neither car stalls Speedometer: 80 MPH Odometer: 01250 Check Engine: Off Speedometer: 70 MPH Odometer: 01250 Check Engine: Off Car 1 has won the race! Race 4: Neither car stalls, both have same speed Speedometer: 70 MPH Odometer: 01250 Check Engine: Off Speedometer: 70 MPH Odometer: 01250 Check Engine: Off It's a tie! Testing difference: Second car will need to drive for 300 minutes to catch first car. First car will need to drive for 60 minutes to catch second car.
THIS IS THE ORIGIANL DASHBOARD CODE
public class Dashboard { private int speedometer; private int odometer; private String checkEngineLight; // default constructor
public Dashboard() { speedometer = 0; odometer = 0; checkEngineLight = "off"; }
// parametrized constructor
public Dashboard(int milesTravelled, int speed) { boolean isValid = true; if(milesTravelled >= 0 && milesTravelled <= 99999) { odometer = milesTravelled; }
else { isValid = false; milesTravelled = 0; }
if(speed >= 0 && speed <= 100) { speedometer = speed; }
else { isValid = false; speedometer = 0; }
if(isValid) checkEngineLight = "Off"; else checkEngineLight = "On"; }
public void accelerate() { this.speedometer += 1; if(speedometer > 100) { speedometer = 0; checkEngineLight = "On"; } } public void drive(int numMinutes) { odometer = odometer + (speedometer * numMinutes / 60); if(odometer > 99999) { odometer = 0; checkEngineLight = "On"; } }
public String toString() { return "Speedometer: " + speedometer + " MPH" + " " + "Odometer: " + String.format("%05d", odometer) + " " + "Check Engine: " + checkEngineLight ; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
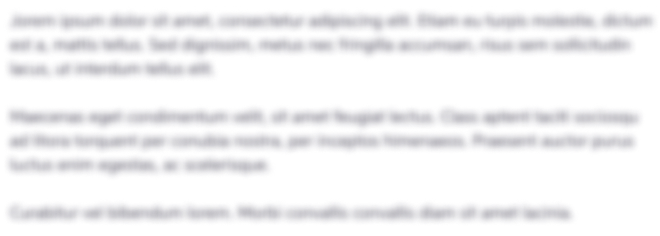
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started