Question
For this assignment, you will be writing a class called FreightTrain that represents a train containing multiple cargo-carrying boxcars. The boxcars in the train are
For this assignment, you will be writing a class called FreightTrain that represents a train containing multiple cargo-carrying boxcars. The boxcars in the train are instances of the Boxcar class that you created in Assignment 2: Part 1
(public class Boxcar{ private String cargo; private int numUnits; private boolean repair; public Boxcar(){ cargo = "gizmos"; numUnits = 5; repair = false; } public Boxcar(String c, int u, boolean r){ if (c.equalsIgnoreCase("gizmos") || c.equalsIgnoreCase("gadgets") || c.equalsIgnoreCase("widgets") || c.equalsIgnoreCase("wadgets")){ cargo = c.toLowerCase(); } else { cargo = "gizmos"; } if (u >= 0 && u <=10) numUnits = u; else numUnits = 0; repair = r; if (repair) numUnits = 0; } public String toString(){ String str = ""; if (repair) str = "in repair"; else str = "in service"; return Integer.toString(numUnits) + " " + cargo + "\t" + str; } public void loadCargo(){ if (repair){ numUnits = 0; System.out.println("can't load.Its in repair"); return; } if (!isFull()) numUnits++; else System.out.println("can't load.Its fully loaded"); } public String getCargo(){ return cargo; } public void setCargo(String c){ if (c.equalsIgnoreCase("gizmos") || c.equalsIgnoreCase("gadgets") || c.equalsIgnoreCase("widgets") || c.equalsIgnoreCase("wadgets")){ cargo = c.toLowerCase(); } else { cargo = "gizmos"; } } public boolean isFull(){ return numUnits == 10; } public void callForRepair(){ repair = true; numUnits = 0; } } public class Demo49{ public static void main(String[] args){ Boxcar b1 = new Boxcar(); System.out.println(b1); Boxcar b2 = new Boxcar("widgets",7,false); System.out.println(b2); Boxcar b3 = new Boxcar("WaDGets",7,true); System.out.println(b3); Boxcar b4 = new Boxcar("OtherStuff",7,true); System.out.println(b4); b4.callForRepair(); System.out.println(b4); Boxcar b5 = new Boxcar("gadgets",8,false); System.out.println(b5); System.out.println(b5.isFull()); Boxcar b6 = new Boxcar("gadgets",10,false); System.out.println(b6); System.out.println(b6.isFull()); b6.loadCargo(); b6.callForRepair(); System.out.println(b6); b2.setCargo("gadgets"); System.out.println(b2); } })- Boxcar. To get started, download the template file FreightTrain.java ( https://s3.amazonaws.com/term.2/week04/FreightTrain.java ). into the same folder that holds your Boxcar.java code from Assignment 2: Part 1 - Boxcar. The FreightTrain class needs the Boxcar class to work properly.
As in Part 1 of this assignment, your job is to add code to FreightTrain.java so that your implementation meets the requirements specified below. To complete the assignment, replace all the /* missing code */ comments in the file with your own code. You do not need to change any other code in the file.
In previous assignments, we had a requirement that your class be named Main. In this assignment, the class is required to be named FreightTrain.
When you are done writing your FreightTrain.java code, you can test it by downloading the Student_Runner_FreightTrain.java (https://s3.amazonaws.com/term.2/week04/Solution/Student_Runner_FreightTrain.java) file into the same directory and running the file. Check to see that your implementation output matches the sample run listed below. You may find that you wish to change some of the test cases in the runner file to more closely test the functionality of your code. We will test your code using our own runner file that is different from the one provided.
When you are done coding and testing, copy and paste your entire FreightTrain class into the Code Runner and press "Submit" in order for your assignment to count as turned in. Do not resubmit your Boxcar class. We will test your FreightTrain class with our own version of the Boxcar class.
Variables
ArrayList
Methods
FreightTrain() - Default constructor that sets train to an ArrayList holding one boxcar containing 5 gizmos, that is not in repair.
FreightTrain(int n) - A constructor that sets train to an ArrayList of size n, holding n boxcars each containing 5 gizmos and not in repair. If n 0, then the train should be set to size one, with a single boxcar holding 5 gizmos and not in repair.
String toString() - This method returns a String representation of the Boxcar objects in the train, one per line. For example:
3 gadgets in service 2 wadgets in service 0 gizmos in repair 7 gadgets in service 0 gadgets in repair
void setCargo(String c) - This method sets the the cargo type of all the boxcars in the entire train. The cargo variable is set to c only if c is "gizmos", "gadgets", widgets, or wadgets. It should ignore the case of the value in c. If c holds any value other than "gizmos", "gadgets", widgets, or wadgets, cargo will be set to "gizmos".
void setMultiCargo() - This method sets the boxcars to the alternating pattern "gizmos", "gadgets", "widgets", "wadgets", "gizmos", "gadgets", "widgets", "wadgets", ... until the end of the train.
void fillTrain() - This method fills all boxcars in the train to the maximum capacity of 10.
void callForRepair(int i) - This method sets the repair variable of the Boxcar at location i to true.
Sample run
1. Test the default constructor FreightTrain() Printing FreightTrain(): 5 gizmos in service 2. Test the constructor FreightTrain(n) Printing emptyTrain (n=0): 5 gizmos in service Printing negativeTrain (n=-7): 5 gizmos in service Printing trainWithFiveCars (n=5): 5 gizmos in service 5 gizmos in service 5 gizmos in service 5 gizmos in service 5 gizmos in service 3. Test setCargo(String) Printing trainWithFiveCars with cargo now gadgets: 5 gadgets in service 5 gadgets in service 5 gadgets in service 5 gadgets in service 5 gadgets in service Printing trainWithFiveCars with cargo of onions (back to gizmos): 5 gizmos in service 5 gizmos in service 5 gizmos in service 5 gizmos in service 5 gizmos in service 4. Test fillTrain() Printing trainWithFiveCars with all Boxcars full: 10 gizmos in service 10 gizmos in service 10 gizmos in service 10 gizmos in service 10 gizmos in service 5. Test callForRepair(n) Printing trainWithFiveCars with second car in repair: 10 gizmos in service 0 gizmos in repair 10 gizmos in service 10 gizmos in service 10 gizmos in service 6. Test setMultiCargo() Printing multi Cargo 8-car train: 5 gizmos in service 5 gadgets in service 5 widgets in service 5 wadgets in service 5 gizmos in service 5 gadgets in service 5 widgets in service 5 wadgets in service
Step by Step Solution
There are 3 Steps involved in it
Step: 1
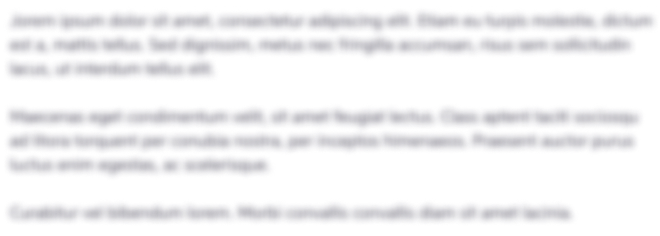
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started