Question
For this assignment, you will create a class, Gameboard, that will contain methods to create a game board and manage certain parts of the game
For this assignment, you will create a class, Gameboard, that will contain methods to create a game board and manage certain parts of the game of Checkers. The board will consist of a 2D array, with each individual space designated by an array of integers representing the row and column indexes. The upper-left square on the board will be at coordinate [0,0]. In the picture above, the white piece in the lower-left corner would be at [7,0], and the upper-right red piece would be at [0,7]. class Gameboard Variables: public char[][] board - a public variable containing the 8x8 game board of characters. Methods: public Gameboard(boolean setup) - Constructor that creates a game board as an 8x8 2D array of characters. If the parameter setup is false, the board will be created empty. If setup is true, the board will be populated with pieces as per the standard game setup in the picture above. The value of each location in the 2D array will be a char with the following characteristics: - for an empty space w for a space occupied by a white checker (note the lowercase w) r for a space occupied by a red piece (note the lowercase r) For the sake of this assignment, white pieces will start along the bottom 3 rows and have a piece occupying the lower left corner, and red pieces will occupy the top 3 rows and will have a piece occupying the upper right corner. boolean move(int x, int y, boolean left) - This method will take a board space as a coordinate pair as a parameter, as well as a boolean left that signifies if the piece should move left (true) or right (false) in the event that a piece can move in both directions. The method will then check for the following conditions, in order of priority Can the piece jump another piece, either forward-left or forward-right? If so, it will do so, in that order of priority. This will involve a) moving this piece to the proper square on the other side of the opponents piece, and then b) removing the opponents piece from the board. Write the helper method jump below to use for this. If left is true, can the piece move forward and to the players left? Note that forward and left are relative to the player; in looking at the gameboard above, forward and to the left for the white player means one spot up and one spot left, but for the red player that means moving one spot down and one spot to the right. If the forward-left spot is available, move the piece to that spot. If the piece cannot move left, or a move to the right is signified with the left parameter being false, can the piece move forward and to the right? If so, move the piece to that spot. The method will return true if a move was successfully executed, or false if none of the conditions above are possible and a move does not occur. Hint: watch out for moves that take a piece off the edge of the board! boolean jump(int x, int y) - This method takes a board location as a parameter and, if a piece exists in that spot, will determine if the piece can capture an opponent piece by jumping forward-left and will do so if it can. If not, it will determine if the piece can jump an opponent piece by jumping forward-right and will do so if it can. Remember, when a piece gets jumped, it is captured and removed from the board. The method will return true if either condition occurs, or false if no jump occurs. Upon landing, this method should immediately check again to see if it can execute a further jump, and execute it if it can. This should continue until no more jumps are possible. Hint: use recursion! You can also make other helper methods to simplify your code. boolean kingMe(int x, int y) - This method should take in a board location as a parameter and king the piece in it by turning the lowercase letter for the piece (w or r) into the uppercase version of the same letter (W or R). This should return true if a piece was successfully kinged, or false if the spot is empty. NOTE: You do not need to worry about handling moves for kings in this assignment. String toString() - Renders the board using the characters -, r, w, R, and W as applicable, in the boards correct shape (8 rows of 8 symbols). In order to make the board easier to read, insert a space between each spaces character. Final Notes For this assignment, both the board variable and all methods should have an access level of public. To test your code, download the student runner file (Links to an external site.)Links to an external site. into the same folder as your Gameboard.java file, run it in DrJava, and check your output against the sample run of the file below. We will use a different runner to grade the program. Remember to change the runner to test different values to make sure your program fits the requirements. When you are ready, paste your entire Gameboard.java class from start to finish in the box below, click run to test the output, and click submit when you are satisfied with your results. Sample run of Student_Runner_Gameboard.java (Links to an external site.)Links to an external site.: Printing blank gameboard: - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - Printing game-ready gameboard: - r - r - r - r r - r - r - r - - r - r - r - r - - - - - - - - - - - - - - - - w - w - w - w - - w - w - w - w w - w - w - w - Testing move method for both colors: Testing move left: - r - r - r - r r - r - r - r - - r - r - - - r - - - - - - r - - w - - - - - - w - - - w - w - - w - w - w - w w - w - w - w - Testing move right: - r - r - r - r r - r - r - - - - r - r - r - r - - - - - - r - - w - - - - - - w - w - w - w - - - - w - w - w w - w - w - w - Testing can't move: false false Testing jump: - - - - - - - - - - - - - - - - - - - - - r - - - - - - - - - - - - - - - - - - - - w - - - - - - - - - - - - - - - - - - - - - Testing multiple jumps: - - - - - - - - - - w - - - - - - - - - - - - - - - - - - - - - - - - - - - - r - - - - - - - - - - - - - - - - - - - - - - - - Testing kingMe: - - - - - K - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - R - - - - -
Step by Step Solution
There are 3 Steps involved in it
Step: 1
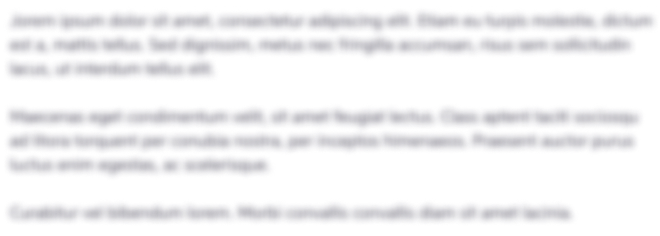
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started