Question
For this assignment, you will write a C program that calculates and displays the prime factorization of any integer greater than 1. Requirements: Your program
For this assignment, you will write a C program that calculates and displays the prime factorization of any integer greater than 1.
Requirements:
- Your program must compile and run correctly using the gcc compiler on ale.
- Your program must prompt the user for an integer greater than 1, and then display its prime factorization in the format used in the Sample Runs below.
- Your program must check that the number entered is, in fact, a positive integer. If it is not, your program should re-prompt until a positive integer is entered. The program need not check, however, whether the user entered an integer in the first place.
- Your program must show the prime factorization as only the product of the prime factors whose factor power is greater than 0.
- Your program must include both the prototype and definition of a function named factor_power(), which takes two integer parameters, n and d, and returns the unique non-negative integer p, such that d raised to the p power divides n but d raised to the (p+1) power does not divide n.
Sample Runs:
% ./factor
Enter an integer (> 1): -1
Enter an integer (> 1): 1
Enter an integer (> 1): 1200
1200 = 2^4 * 3^1 * 5^2
% ./factor
Enter an integer (> 1): 17
17 = 17^1
% ./factor
Enter an integer (> 1): 123456789
123456789 = 3^2 * 3607^1 * 3803^1
Notes on mathematics:
- A factor of a positive integer n is an integer which evenly divides n; that is, one which leaves no remainder when n is divided by it. Thus 2, 3, 4, and 6 are all factors of 12, but 5 is not, as 12 divided by 5 has a remainder of 2. We can test whether one number is a factor of another using the modulus (%) operator, which returns the remainder from integer division. Thus 12%5 is 2, while 12%6 is 0. Every number is a factor of itself, and 1 is a factor of every number.
- A prime number is a positive integer which is greater than 1 whose only factors are 1 and itself. Thus 17 is a prime number, whereas 12 is not.
- The prime factorization of a positive integer n is the unique list of prime numbers which when multiplied together result in n. Thus the prime factorization of 12 is 2^2 * 3^1, while that of 625 is 5^4.
Note: we write 2^2 instead of 2 * 2, and 3^1 instead of 3, and 5^4 instead of 5 * 5 * 5 * 5.
Please solve the above assignment using C programming language. Please solve it as soon as possible and solve it correctly and in a clear way.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
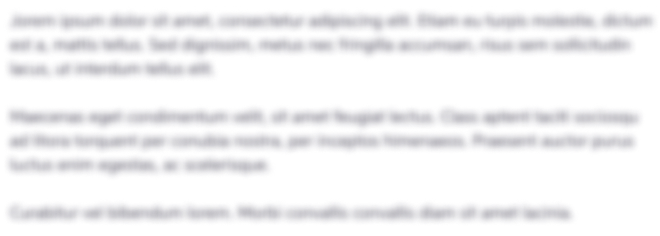
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started