Question
For this assignment you will write a method that handles an exception and a method that throws an exception. For this assignment we will work
For this assignment you will write a method that handles an exception and a method that throws an exception.
For this assignment we will work with just one type exception InputMismatchException. This will allow us to experiment with exception handling without bringing in too much complexity.
You will begin by writing a class that stores a single integer as its instance variable. Before advancing to exception handling, write a simple class with the following:
- One instance variable of type int.
- A full constructor:
- Constructor receives an integer as a parameter from the calling method and stores it in the objects instance variable.
- A .get() method that returns to the calling method the value currently stored in the objects instance variable.
- A .set() method that receives an integer as a parameter from the calling method and stores it in the objects instance variable.
- A .toString() method that returns a string containing a copy of the current value stored in the objects instance variable.
Before going further, write a test program that allows the user to input an integer. The test program will set up an object of your class; you should also check your classs .get()/.set() and .toString() methods to be sure your class works before adding the required methods.
- This testing will assure you that your class works as designed so far. Its important to make this sort of test so that you arent trying to add exception handling to a class that does not work otherwise as designed.
You will now add two methods to your class. Both methods will accept no parameters from the calling method; both will also be void methods. Each of them will allow the user to input an int value from the keyboard and will then print the product of this value and the current value of the objects instance variable. For example, if the user inputs 5 and the objects instance variable contains 4, the methods output might look like the following: 5 * 4 = 20
Since the value input from the keyboard is to be read as an int, theres always the possibility that the user will enter an improper value; for example, they might mistakenly type 4.2. Without exception handling, this InputMismatchException would cause the program to crash.
Your task is to prevent a crash.
- One of your methods will handle an InputMismatchException internally.
- The other method will throw an InputMismatchException that will be handled with a trycatch construction in the calling method (in this simple case main()).
With both the trycatch constructions include a finally clause that prints a message stating that control is inside the finally clause. This will allow us to see that the finally clause is executed whether or not an exception occurs.
These two methods will be called from main(). After the calls to these methods in main(), have a statement that displays a message stating that we are exiting our test program. This will allow the user to see that the exceptions are handled and the program terminates cleanly.
A run of the program in which no exceptions occur might look like the following:
Type an integer: 5
Integer input: 5
myClass contains 5
Call method that throws InputMismatchException.
Type multiplier for multiplyThrows(): 4
5 * 4 = 20
Inside finally clause associated with multiplyThrows.
Call method that handles InputMismatchException.
Type multiplier for multiplyHandles(): 6
5 * 6 = 30
Inside multiplyHandles finally clause.
Exiting TwoEntryExceptions.
It is possible that calls to both methods result in exceptions; in that case the programs output might look like the following:
Type an integer: 5
Integer input: 5
myClass contains 5
Call method that throws InputMismatchException.
Type multiplier for multiplyThrows(): 4.2
InputMismatchException thrown by multiplyThrows().
Inside finally clause associated with multiplyThrows.
Call method that handles InputMismatchException.
Type multiplier for multiplyHandles(): 7.3
InputMismatchException caught in multipliesHandle().
Inside multiplyHandles finally clause.
Exiting TwoEntryExceptions.
It is also possible that the data entry may be correct in one of the method calls and incorrect in the other. In this case the output might look like the following:
Type an integer: 5
Integer input: 5
myClass contains 5
Call method that throws InputMismatchException.
Type multiplier for multiplyThrows(): 4.2
InputMismatchException thrown by multiplyThrows().
Inside finally clause associated with multiplyThrows.
Call method that handles InputMismatchException.
Type multiplier for multiplyHandles(): 6
5 * 6 = 30
Inside multiplyHandles finally clause.
Exiting TwoEntryExceptions.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
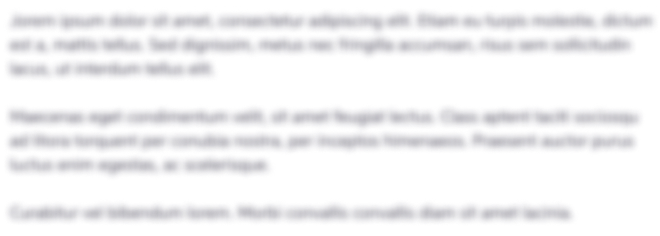
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started