Question
For this assignment you will write a program that does a frequency analysis on a file. A key is created based on that frequency analysis.
"For this assignment you will write a program that does a frequency analysis on a file. A key is created based on that frequency analysis. The file is then displayed using the initial key. The user of the program is then asked if they want to make a change to the key. They will be asked what character in the decrypted version of the text they want to change and what that character should decrypt to instead.
sample log of a program run you must display the frequency of all the printable ASCII characters. (Ignore ASCII chars 0 - 31 and 127). See this section of the Wikipedia article for more on the printable ASCII characters.
Use the other method from DecryptUtilities. The DecryptUtilities.getDecryptionKey(int[]) method takes a parameter that is an array of ints. The method expects that the array of ints has a length of 128 and that it represents the frequency table for the encrypted text. The method then creates and returns an array of chars that is the initial decryption key. The array shall have a length of 128. Recall, the key is used to decrypt the message. The array of chars that is returned relies on mapping. Thus the index of the array indicates the ASCII code of the character in the encrypted text and the actual element is character than the encrypted character is changed into. Here is an example. Assume we obtain the array of chars from the DecryptUtilities.getDecryptionKey(int[]) method. Assume this is a portion of the array. (I don't show all of it.)
index | 65 | 66 | 67 | 68 | 69 | 70 | 71 | 72 | 73 | 74 |
element | 'c' | ' ' | '.' | 'a' | '!' | 's' | 't' | 'r' | 'e' | 'B' |
Again, that is only a portion of the array. Index 65 maps to ASCII character 65. ASCII code 65 is 'A'. Thus an 'A' in the encrypted message will decrypt to a 'c' based on the current key. ASCII code 66 is 'B'. Thus a 'B' in the encrypted message will decrypt to a ' ' (a space) based on the current key. And so forth. So if we had this encrypted message: JIBDBFGDHEE
and used the key shown above to decrypt it we would get Be a star!! J in the first character in the encrypted message. J has an ASCII code of 74. Going to index 64 in the array that represents the key we have a B. So J becomes B. I has an ASCII code of 73 so I becomes e. And so forth. The array returned from the method DecryptUtilities.getDecryptionKey(int[]) is used to transform the encrypted message to a decrypted message.
After obtaining the initial key display it (as in the sample log) and then display the decrypted version of the text. Create a new String that is the decrypted version of the text. Don't overwrite the String that is the encrypted version. You'll need it again later.
The trouble with this approach is that with short messages there will be differences between the expected frequency of letters and the actual frequencies. The decrypted text probably won't be perfect unless it is several thousand characters long. Even then there could be mistakes due to differences between the standard frequencies of characters and the actual frequency of characters in the original text. So now the user becomes a detective. Ask them if they want to make a change to the key. If they answer 'Y' or 'y', ask what decrypted character they want to change. Then ask what that decrypted character should decrypt to instead. For example if we display the decrypted text and we saw tde over and over again we would be fairly certain that whatever character is decrypting to d should decrypt to h instead since you would expect most texts to have a lot of instances of the word the. Search the key for the element that contains 'd' and change it to 'h'. If we left it at that we there would be a problem. There would now be two encrypted characters that decrypt to 'h'. That can make the process harder. So we will change whatever was decrypting to 'h' to decrypt to 'd' instead. (we swap the characters in the array after we find them both.) This isn't always a perfect change but it makes it easier to eventually get the correct key..
After a change in the key display the new version of the decrypted file. Keep asking the user if they want to make a change, what they want to change, and display the new version until they want to stop.
After the user doesn't want any more changes display the final version of the key and the decrypted text.
Things to remember:
We are mapping the ASCII values of chars to indices in the array. Both when you generate the initial frequency array and in the key itself.
You can convert from a char to an int without casting. int x = ch; // if ch is a char, x now holds the ASCII code for that char
Converting from an int to a char requires casting char ch = (char) x; // if x is an int, ch now holds the ASCII character associated with the code x
Break the problem up into methods. Test methods before going on. This will require writing some testing code that you delete before turning in your program.. Simple println statements are VERY useful when testing and debugging.
As always you must use good program hygiene. (Constants, meaningful variable names, well structured code, removal of redundancy, correct spacing and tabbing and alignment of braces.)"
Step by Step Solution
There are 3 Steps involved in it
Step: 1
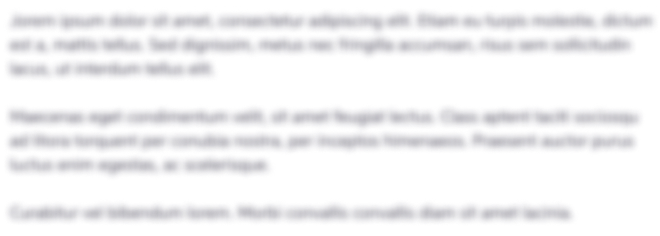
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started