Question
For this assignment, you will write an assembly language subroutine that prints out a 32-bit unsigned number to standard output. The 32-bit value will be
For this assignment, you will write an assembly language subroutine that prints out a 32-bit unsigned number to standard output. The 32-bit value will be passed to your subroutine through the EAX register. Your subroutine must preserve all registers (i.e., any registers that you use must be saved on the stack and restored before you return). Your subroutine should do nothing else (not even print a linefeed).
To determine the values of the base 10 digits in the decimal representation of the number, you will use the division method (don't use repeated subtraction). The DIV instruction will divide the 64-bit value stored in EDX:EAX by the single 32-bit operand given. After the DIV instruction is executed, the quotient is stored in the EAX register and the remainder is stored in the EDX register. (See further discussion of the DIV instruction in Implementation Notes below.)
Implement your subroutine in a separate file. The entry point of your subroutine must be called prt_dec. The "main program" will reside in a separate file.
NOTES:
In the division method for converting a number to base 10, you will get the one's place first (the least significant digit), then the 10's place, then the 100's place, ... This is in the opposite order that the decimal number will be printed. The easiest way to handle this situation is to prepare a string starting at a higher numbered address and loop backwards storing each character at a lower numbered address.
The number of characters that you will print will depend on the value passed in the EAX register. You will need to determine or keep count of the length of the decimal number to be printed.
If the value in the EAX register is 0, then your subroutine should print out a single digit 0.
In order for code in other files to call your subroutine, you must declare the prt_dec label to be global. (If the label is not global, then only code from the same file can use that label.)
Thus, you must include the following declaration in your prt_dec.asm file:
global prt_dec
The global declarations are typically made just after the SECTION .text declaration.
Since you are converting a 32-bit value into decimal, the dividend always fits in the lower 32 bits. Thus, you must zero out the EDX register before each DIV instruction.
We are always dividing by 10 in this program. So, we know that the remainder in EDX will always be less than 10. This value fits in 8 bits, which is quite rather convenient.
Remember that the bytes that you are printing to the screen will be interpreted as ASCII values.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
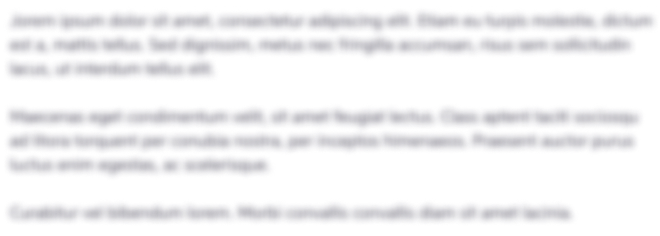
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started