Question
For this exercise, you will be writing a java application in support of a Dessert Shoppe which sells candy by the pound, cookies by the
For this exercise, you will be writing a java application in support of a Dessert Shoppe which sells candy by the pound, cookies by the dozen, ice cream, and sundaes (ice cream with a topping). Your program will be used for the checkout system. To do this, you will implement an inheritance hierarchy of classes derived from a DessertItem abstract superclass. The Candy, Cookie, and IceCream classes will be derived from the DessertItem class. The Sundae class will be derived from the IceCream class. You will also write a Checkout class which maintains a list (Vector) of DessertItem's. The DessertItem Class
The DessertItem class is an abstract superclass from which specific types of DessertItems can be derived. It contains only one data member, a name. It also defines a few methods. All of the DessertItem class methods except the getCost() method are defined in a generic way in the file, DessertItem.java, provided for you along with the other homework specific files in the directory. The getCost() method is an abstract method that is not defined in the DessertItem class because the method of determining the costs varies based on the type of item. Tax amounts should be rounded to the nearest cent. For example, the calculating the tax on a food item with a cost of 199 cents with a tax rate of 2.0% should be 4 cents. DO NOT CHANGE THE DessertItem.java file! Your code must work with this class as it is provided.
DessertItem.java
public abstract class DessertItem { protected String name; /** * Null constructor for DessertItem class */ public DessertItem() { this(""); } /** * Initializes DessertItem data */ public DessertItem(String name) { if (name.length() <= DessertShoppe.MAX_ITEM_NAME_SIZE) this.name = name; else this.name = name.substring(0,DessertShoppe.MAX_ITEM_NAME_SIZE); } /** * Returns name of DessertItem * @return name of DessertItem */ public final String getName() { return name; } /** * Returns cost of DessertItem * @return cost of DessertItem */ public abstract int getCost(); /** * Returns the name of the dessert item and it's cost as formatted String. * @return name and cost of the dessert item. */ @Override public String toString(){ return String.format("%-"+DessertShoppe.MAX_ITEM_NAME_SIZE+"s"+"%"+DessertShoppe.COST_WIDTH+"s",getName(), DessertShoppe.cents2dollarsAndCents(getCost())); }
}
The DessertShoppe Class The DessertShoppe class is also provided for you in the file, DessertShoppe.java. It contains constants such as the tax rate as well the name of the store, the maximum size of an item name and the width used to display the costs of the items on the receipt. Your code should use these constants wherever necessary! The DessertShoppe class also contains the cents2dollarsAndCentsmethod which takes an integer number of cents and returns it as a String formatted in dollars and cents. For example, 105 cents would be returned as "1.05". DO NOT CHANGE THE DessertShoppe.java file! Your code must work with this class as it is provided.
DessertShoppe.java
public class DessertShoppe {
public final static double TAX_RATE = 6.5; // 6.5% public final static String STORE_NAME = "Kamloops Dessert Shoppe"; public final static int MAX_ITEM_NAME_SIZE = 25; public final static int COST_WIDTH = 6; public final static int MAX_CHECKOUT_ITMES = 100; /** * A static method, which takes an integer number of cents and returns it as * a String formatted in dollars and cents. * <\br> For example, 105 cents would be returned as "1.05". * @param cents number of cents. * @return */ public static String cents2dollarsAndCents(int cents) { String s = ""; if (cents < 0) { s += "-"; cents *= -1; } int dollars = cents/100; cents = cents % 100; if (dollars > 0) s += dollars; s +="."; if (cents < 10) s += "0"; s += cents; return s; } }
The Derived Classes All the classes which are derived from the DessertItem class must define a constructor. Please see the provided TestCheckout class, TestCheckout.java (see a sample test run of this test class at the end of this document), to determine the parameters for the various constructors. Each derived class should be implemented by creating a file with the correct name, eg., Candy.java. The Candy class should be derived from the DessertItem class. A Candy item has a weight and a price per pound which are used to determine its cost. For example, 2.30 lbs.of fudge @ .89 /lb. = 205 cents. The cost should be rounded to the nearest cent. The Cookie class should be derived from the DessertItem class. A Cookie item has a number and a price per dozen which are used to determine its cost. For example, 4 cookies @ 399 cents /dz. = 133 cents. The cost should be rounded to the nearest cent. The IceCream class should be derived from the DessertItem class. An IceCream item simply has a cost. The Sundae class should be derived from the IceCream class. The cost of a Sundae is the cost of the IceCream plus the cost of the topping. You must implement all derived classes with appropriate behavior.
The Checkout Class The Checkout class, provides methods to enter dessert items into the cash register, clear the cash register, get the number of items, get the total cost of the items (before tax), get the total tax for the items, and get a String representing a receipt for the dessert items. The Checkout class must use an array to store the DessertItem's (max size of the array should be set from constant taken from the DessertShoppe class). The total tax should be rounded to the nearest cent. The complete specifications for the Checkout class are provided for you in JavaDoc format (provided in javadoc folder)
Checkout.java
class Checkout { private DessertItem [] items; private int noi;
/** * Creates a Checkout instance with an empty list of DessertItem's. */ public Checkout() { items = new DessertItem[DessertShoppe.MAX_CHECKOUT_ITMES]; noi = 0; } /** * Clears the Checkout to begin checking out a new set of items. */ public void clear(){ noi = 0; } /** * A DessertItem is added to the end of the list of items. * @param item DessertItem to add to list of items. */ public void enterItem(DessertItem item) { items[noi++] = item; } /** * Returns the number of DessertItem's in the list. * @return number of DessertItem's in the list. */ public int numberOfItems(){ return noi; } /** * Returns total cost of items in cents (without tax). * @return total cost of items in cents (without tax). */ public int totalCost(){ int tc = 0; for(int i=0; i } Testing A simple test driver, TestCheckout.java along with its expected output (see below), are provided for you to test your class implementations. You must add additional tests to the driver to more thoroughly test your code. Sample Test Run Output from TestCheckout.java file: Number of items: 4 Total cost: 1331 Total tax: 87 Cost + Tax: 1418 Kamloops Dessert Shoppe -------------------- 2.25 lbs. @ 3.99/lb. Peanut Butter Fudge 8.98 Vanilla Ice Cream 1.05 Hot Fudge Sundae with Choc. Chip Ice Cream 1.95 4 @ 3.99/dz. Oatmeal Raisin Cookies 1.33 Tax .87 Total Cost 14.18 Number of items: 6 Total cost: 1192 Total tax: 77 Cost + Tax: 1269 Kamloops Dessert Shoppe -------------------- Strawberry Ice Cream 1.45 Caramel Sundae with Vanilla Ice Cream 1.55 1.33 lbs. @ .89/lb. Gummy Worms 1.18 4 @ 3.99/dz. Chocolate Chip Cookies 1.33 1.5 lbs. @ 2.09/lb. Salt Water Taffy 3.14 3.0 lbs. @ 1.09/lb. Candy Corn 3.27 Tax .77 Total Cost 12.69
Step by Step Solution
There are 3 Steps involved in it
Step: 1
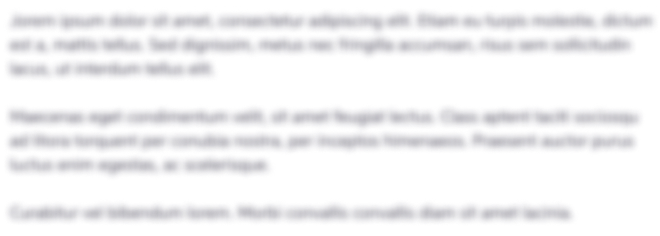
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started