Answered step by step
Verified Expert Solution
Question
1 Approved Answer
For this exercise, you will need to make an application that has a Graphical User Interface that represents a timer. Template files: Controller.java *
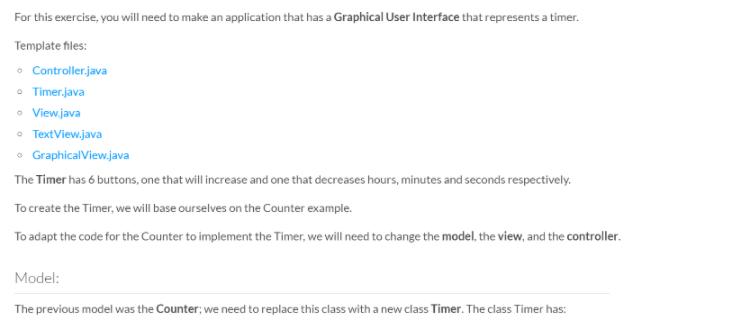
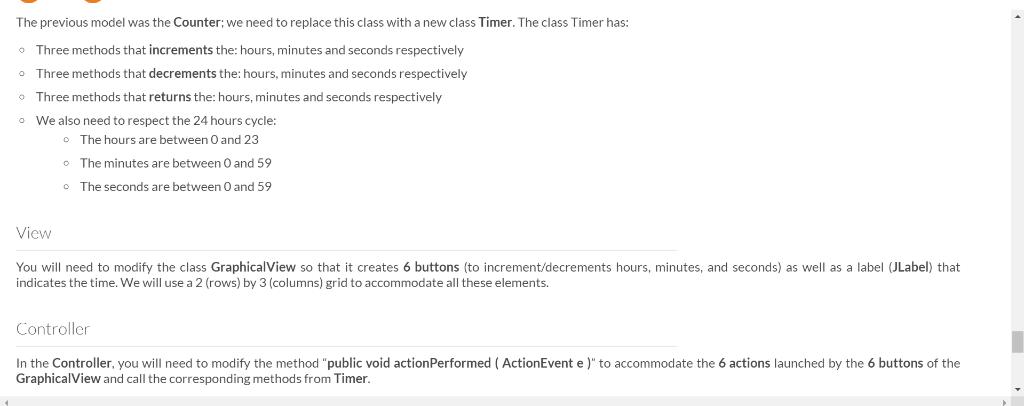
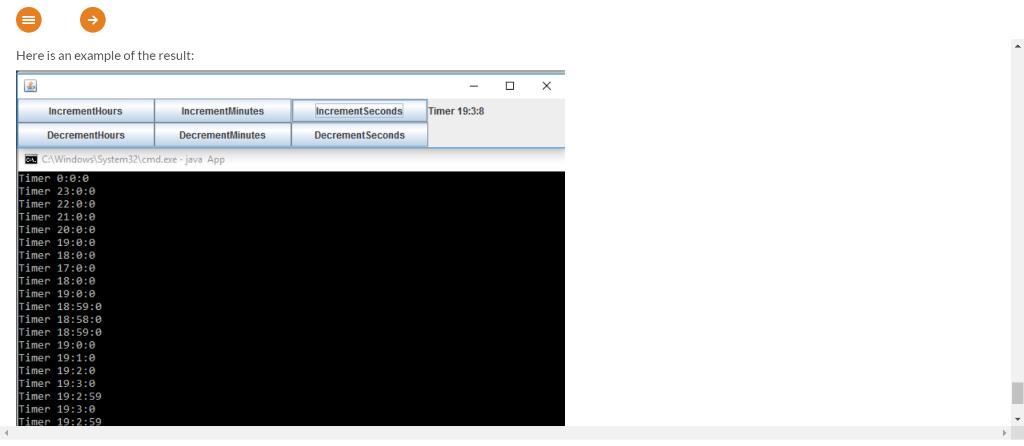
![import java.awt.event.*;public class Controller implements ActionListenerprivate Timer model;private View] views;private](https://dsd5zvtm8ll6.cloudfront.net/si.experts.images/questions/2022/03/623457f6b112f_1647597556505.jpg)
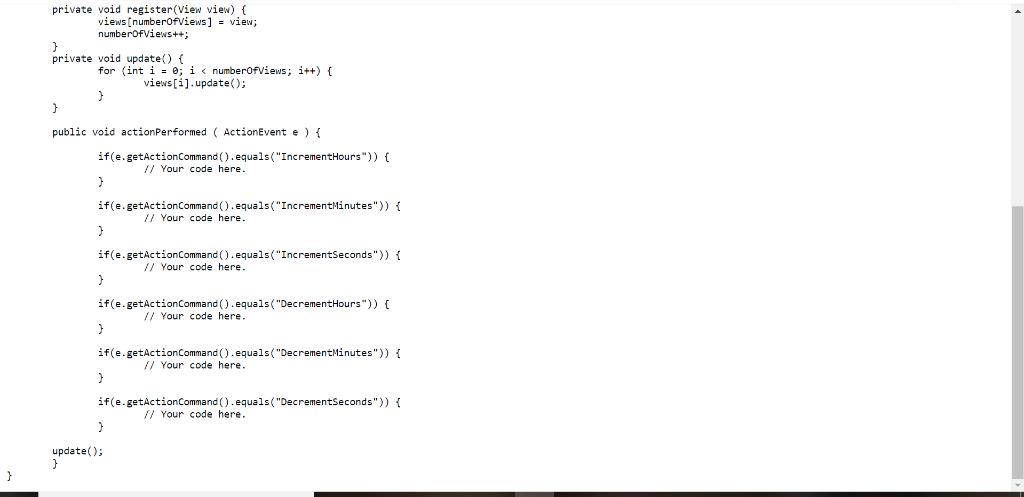
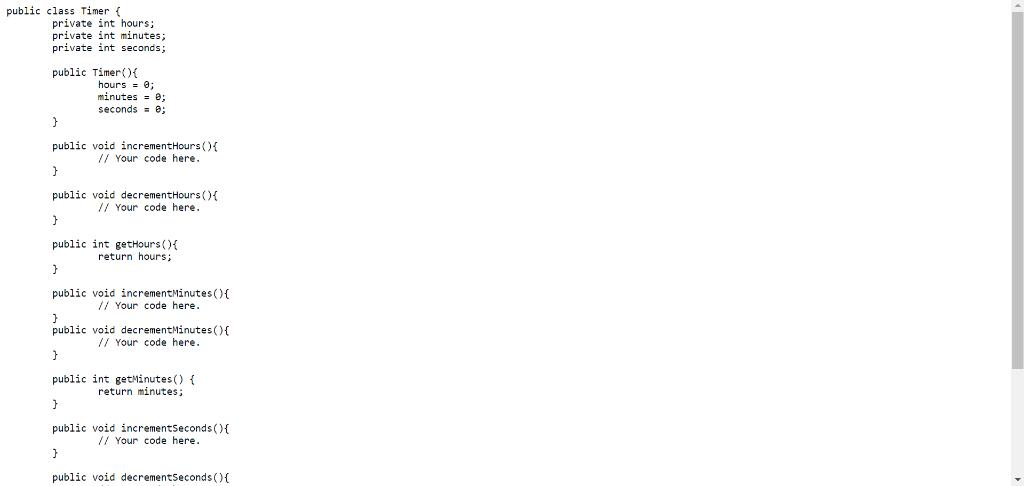
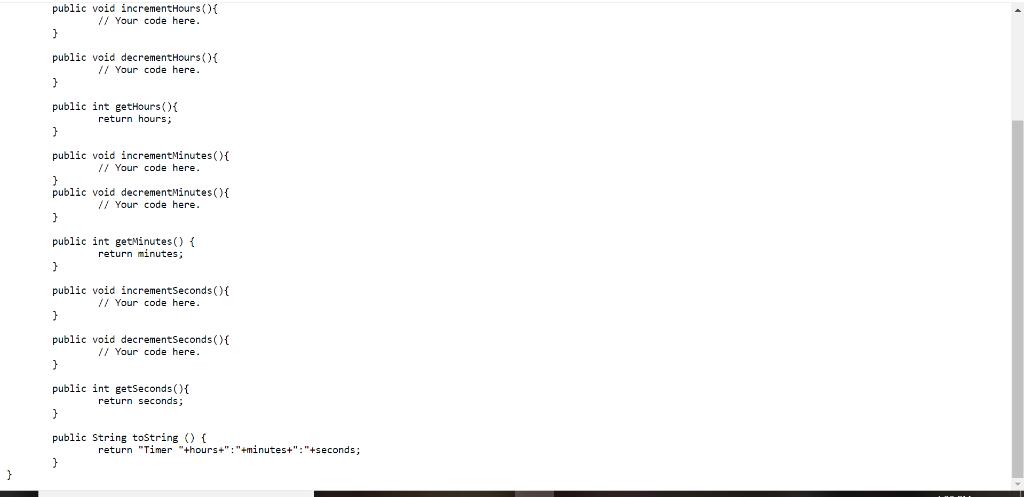
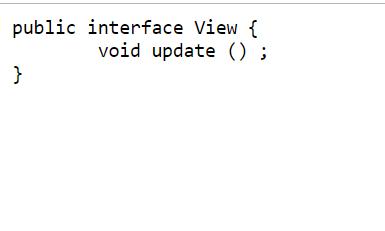
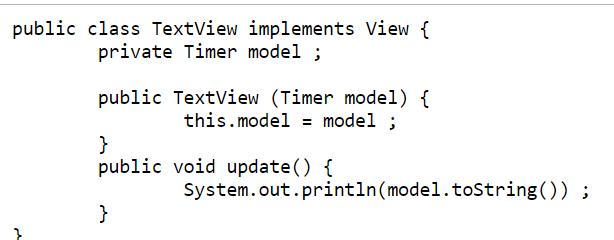
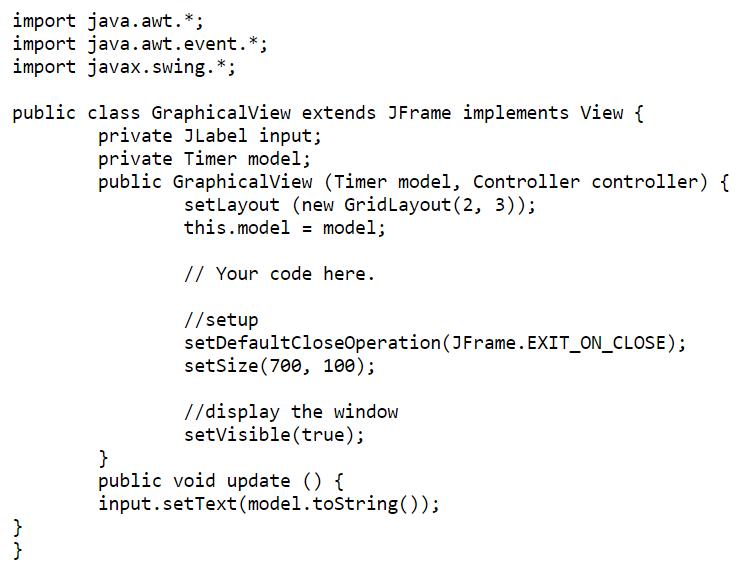
For this exercise, you will need to make an application that has a Graphical User Interface that represents a timer. Template files: Controller.java * Timer.java View.java TextView.java GraphicalView.java The Timer has 6 buttons, one that will increase and one that decreases hours, minutes and seconds respectively. To create the Timer, we will base ourselves on the Counter example. To adapt the code for the Counter to implement the Timer, we will need to change the model, the view, and the controller. Model: The previous model was the Counter; we need to replace this class with a new class Timer. The class Timer has: The previous model was the Counter; we need to replace this class with a new class Timer. The class Timer has: Three methods that increments the: hours, minutes and seconds respectively Three methods that decrements the: hours, minutes and seconds respectively Three methods that returns the: hours, minutes and seconds respectively o We also need to respect the 24 hours cycle: O The hours are between 0 and 23 o The minutes are between 0 and 59 The seconds are between O and 59 View You will need to modify the class GraphicalView so that it creates 6 buttons (to increment/decrements hours, minutes, and seconds) as well as a label (JLabel) that indicates the time. We will use a 2 (rows) by 3 (columns) grid to accommodate all these elements. Controller In the Controller, you will need to modify the method "public void actionPerformed ( ActionEvent e )" to accommodate the 6 actions launched by the 6 buttons of the GraphicalView and call the corresponding methods from Timer. Here is an example of the result: IncrementHours IncrementMinutes IncrementSeconds Timer 19:3:8 DecrementHours DecrementMinutes DecrementSeconds CAWindowsSystem32\cmd.exe-java App Timer e:0:e Timer 23:0:0 Timer 22:0:0 Timer 21:0:0 Timer 20:0:e Timer 19:0:e Timer 18:0:0 Timer 17:0:0 Timer 18:0:0 Timer 19:0:e Timer 18:59:0 Timer 18:58:0 Timer 18:59:0 Timer 19:0:0 Timer 19:1:0 Timer 19:2:0 Timer 19:3:0 Timer 19:2:59 Timer 19:3:0 Timer 19:2:59 import java.awt.event.*; public class Controller implements ActionListener { private Timer model; private View[) views; private int numberofviews; public Controller(){ views = new View[2]; numberofviews = 0; model = new Timer(); register(new GraphicalView(model, this)); register(new TextView(model)); update(); public Timer getModel () { return model; public View[] getviews () { return views; private void register(View view) { views[numberofviews] = view; numberofviews++; private void update() ( for (int i = 0; i numberofViews; i++) { views[i].update(); public void actionPerformed (ActionEvent e ) { private void register(View view) { views(numberofviews] - view; numberofviews++; } private void update() ( for (int i = 0; i numberofViews; i++) { views[i].update(); public void actionPerformed ( ActionEvent e ) { if(e.getActionCommand ().equals("IncrementHours")) { // Your code here. if(e.getActionCommand ().equals("IncrementMinutes")) { // Your code here. if(e.getActionCommand ().equals ("IncrementSeconds")) { // Your code here. if(e.getActionCommand ().equals ("DecrementHours")) { // Your code here. if(e.getActionCommand ().equals("DecrementMinutes")) { // Your code here. if(e.getActionCommand ().equals ("DecrementSeconds")) { // Your code here. update(); public class Timer { private int hours; private int minutes; private int seconds; public Timer(){ hours = 0; minutes = 0; seconds = e; public void incrementHours (){ // Your code here. public void decrementHours(){ // Your code here. public int getHours (){ return hours; public void incrementMinutes (O{ // Your code here. } public void decrementMinutes(){ // Your code here. public int getMinutes () { return minutes; public void incrementSeconds (){ // Your code here. public void decrementSeconds (){ public void incrementHours (( // Your code here. public void decrementHours ({ // Your code here. public int getHours (){ return hours; public void incrementMinutes(){ // Your code here. public void decrementMinutes (){ // Your code here. public int getMinutes () { return minutes; public void incrementSeconds (O // Your code here. public void decrementSeconds (){ // Your code here. public int getSeconds (){ return seconds; public String tostring () { return "Timer "+hours+":"+minutes+":"+seconds; } public interface View { void update () ; } public class TextView implements View { private Timer model ; public TextView (Timer model) { this.model = model ; } public void update() { System.out.println(model.toString()) ; } import java.awt.*; import java. awt.event.*; import javax.swing.*; public class GraphicalView extends JFrame implements View { private JLabel input; private Timer model; public GraphicalView (Timer model, Controller controller) { setlayout (new GridLayout (2, 3)); this.model = model; // Your code here. //setup setDefaultcloseOperation(JFrame.EXIT_ON_CLOSE); setSize(700, 100); //display the window setVisible(true); } public void update () { input.setText(model.toString()); } } For this exercise, you will need to make an application that has a Graphical User Interface that represents a timer. Template files: Controller.java * Timer.java View.java TextView.java GraphicalView.java The Timer has 6 buttons, one that will increase and one that decreases hours, minutes and seconds respectively. To create the Timer, we will base ourselves on the Counter example. To adapt the code for the Counter to implement the Timer, we will need to change the model, the view, and the controller. Model: The previous model was the Counter; we need to replace this class with a new class Timer. The class Timer has: The previous model was the Counter; we need to replace this class with a new class Timer. The class Timer has: Three methods that increments the: hours, minutes and seconds respectively Three methods that decrements the: hours, minutes and seconds respectively Three methods that returns the: hours, minutes and seconds respectively o We also need to respect the 24 hours cycle: O The hours are between 0 and 23 o The minutes are between 0 and 59 The seconds are between O and 59 View You will need to modify the class GraphicalView so that it creates 6 buttons (to increment/decrements hours, minutes, and seconds) as well as a label (JLabel) that indicates the time. We will use a 2 (rows) by 3 (columns) grid to accommodate all these elements. Controller In the Controller, you will need to modify the method "public void actionPerformed ( ActionEvent e )" to accommodate the 6 actions launched by the 6 buttons of the GraphicalView and call the corresponding methods from Timer. Here is an example of the result: IncrementHours IncrementMinutes IncrementSeconds Timer 19:3:8 DecrementHours DecrementMinutes DecrementSeconds CAWindowsSystem32\cmd.exe-java App Timer e:0:e Timer 23:0:0 Timer 22:0:0 Timer 21:0:0 Timer 20:0:e Timer 19:0:e Timer 18:0:0 Timer 17:0:0 Timer 18:0:0 Timer 19:0:e Timer 18:59:0 Timer 18:58:0 Timer 18:59:0 Timer 19:0:0 Timer 19:1:0 Timer 19:2:0 Timer 19:3:0 Timer 19:2:59 Timer 19:3:0 Timer 19:2:59 import java.awt.event.*; public class Controller implements ActionListener { private Timer model; private View[) views; private int numberofviews; public Controller(){ views = new View[2]; numberofviews = 0; model = new Timer(); register(new GraphicalView(model, this)); register(new TextView(model)); update(); public Timer getModel () { return model; public View[] getviews () { return views; private void register(View view) { views[numberofviews] = view; numberofviews++; private void update() ( for (int i = 0; i numberofViews; i++) { views[i].update(); public void actionPerformed (ActionEvent e ) { private void register(View view) { views(numberofviews] - view; numberofviews++; } private void update() ( for (int i = 0; i numberofViews; i++) { views[i].update(); public void actionPerformed ( ActionEvent e ) { if(e.getActionCommand ().equals("IncrementHours")) { // Your code here. if(e.getActionCommand ().equals("IncrementMinutes")) { // Your code here. if(e.getActionCommand ().equals ("IncrementSeconds")) { // Your code here. if(e.getActionCommand ().equals ("DecrementHours")) { // Your code here. if(e.getActionCommand ().equals("DecrementMinutes")) { // Your code here. if(e.getActionCommand ().equals ("DecrementSeconds")) { // Your code here. update(); public class Timer { private int hours; private int minutes; private int seconds; public Timer(){ hours = 0; minutes = 0; seconds = e; public void incrementHours (){ // Your code here. public void decrementHours(){ // Your code here. public int getHours (){ return hours; public void incrementMinutes (O{ // Your code here. } public void decrementMinutes(){ // Your code here. public int getMinutes () { return minutes; public void incrementSeconds (){ // Your code here. public void decrementSeconds (){ public void incrementHours (( // Your code here. public void decrementHours ({ // Your code here. public int getHours (){ return hours; public void incrementMinutes(){ // Your code here. public void decrementMinutes (){ // Your code here. public int getMinutes () { return minutes; public void incrementSeconds (O // Your code here. public void decrementSeconds (){ // Your code here. public int getSeconds (){ return seconds; public String tostring () { return "Timer "+hours+":"+minutes+":"+seconds; } public interface View { void update () ; } public class TextView implements View { private Timer model ; public TextView (Timer model) { this.model = model ; } public void update() { System.out.println(model.toString()) ; } import java.awt.*; import java. awt.event.*; import javax.swing.*; public class GraphicalView extends JFrame implements View { private JLabel input; private Timer model; public GraphicalView (Timer model, Controller controller) { setlayout (new GridLayout (2, 3)); this.model = model; // Your code here. //setup setDefaultcloseOperation(JFrame.EXIT_ON_CLOSE); setSize(700, 100); //display the window setVisible(true); } public void update () { input.setText(model.toString()); } } For this exercise, you will need to make an application that has a Graphical User Interface that represents a timer. Template files: Controller.java * Timer.java View.java TextView.java GraphicalView.java The Timer has 6 buttons, one that will increase and one that decreases hours, minutes and seconds respectively. To create the Timer, we will base ourselves on the Counter example. To adapt the code for the Counter to implement the Timer, we will need to change the model, the view, and the controller. Model: The previous model was the Counter; we need to replace this class with a new class Timer. The class Timer has: The previous model was the Counter; we need to replace this class with a new class Timer. The class Timer has: Three methods that increments the: hours, minutes and seconds respectively Three methods that decrements the: hours, minutes and seconds respectively Three methods that returns the: hours, minutes and seconds respectively o We also need to respect the 24 hours cycle: O The hours are between 0 and 23 o The minutes are between 0 and 59 The seconds are between O and 59 View You will need to modify the class GraphicalView so that it creates 6 buttons (to increment/decrements hours, minutes, and seconds) as well as a label (JLabel) that indicates the time. We will use a 2 (rows) by 3 (columns) grid to accommodate all these elements. Controller In the Controller, you will need to modify the method "public void actionPerformed ( ActionEvent e )" to accommodate the 6 actions launched by the 6 buttons of the GraphicalView and call the corresponding methods from Timer. Here is an example of the result: IncrementHours IncrementMinutes IncrementSeconds Timer 19:3:8 DecrementHours DecrementMinutes DecrementSeconds CAWindowsSystem32\cmd.exe-java App Timer e:0:e Timer 23:0:0 Timer 22:0:0 Timer 21:0:0 Timer 20:0:e Timer 19:0:e Timer 18:0:0 Timer 17:0:0 Timer 18:0:0 Timer 19:0:e Timer 18:59:0 Timer 18:58:0 Timer 18:59:0 Timer 19:0:0 Timer 19:1:0 Timer 19:2:0 Timer 19:3:0 Timer 19:2:59 Timer 19:3:0 Timer 19:2:59 import java.awt.event.*; public class Controller implements ActionListener { private Timer model; private View[) views; private int numberofviews; public Controller(){ views = new View[2]; numberofviews = 0; model = new Timer(); register(new GraphicalView(model, this)); register(new TextView(model)); update(); public Timer getModel () { return model; public View[] getviews () { return views; private void register(View view) { views[numberofviews] = view; numberofviews++; private void update() ( for (int i = 0; i numberofViews; i++) { views[i].update(); public void actionPerformed (ActionEvent e ) { private void register(View view) { views(numberofviews] - view; numberofviews++; } private void update() ( for (int i = 0; i numberofViews; i++) { views[i].update(); public void actionPerformed ( ActionEvent e ) { if(e.getActionCommand ().equals("IncrementHours")) { // Your code here. if(e.getActionCommand ().equals("IncrementMinutes")) { // Your code here. if(e.getActionCommand ().equals ("IncrementSeconds")) { // Your code here. if(e.getActionCommand ().equals ("DecrementHours")) { // Your code here. if(e.getActionCommand ().equals("DecrementMinutes")) { // Your code here. if(e.getActionCommand ().equals ("DecrementSeconds")) { // Your code here. update(); public class Timer { private int hours; private int minutes; private int seconds; public Timer(){ hours = 0; minutes = 0; seconds = e; public void incrementHours (){ // Your code here. public void decrementHours(){ // Your code here. public int getHours (){ return hours; public void incrementMinutes (O{ // Your code here. } public void decrementMinutes(){ // Your code here. public int getMinutes () { return minutes; public void incrementSeconds (){ // Your code here. public void decrementSeconds (){ public void incrementHours (( // Your code here. public void decrementHours ({ // Your code here. public int getHours (){ return hours; public void incrementMinutes(){ // Your code here. public void decrementMinutes (){ // Your code here. public int getMinutes () { return minutes; public void incrementSeconds (O // Your code here. public void decrementSeconds (){ // Your code here. public int getSeconds (){ return seconds; public String tostring () { return "Timer "+hours+":"+minutes+":"+seconds; } public interface View { void update () ; } public class TextView implements View { private Timer model ; public TextView (Timer model) { this.model = model ; } public void update() { System.out.println(model.toString()) ; } import java.awt.*; import java. awt.event.*; import javax.swing.*; public class GraphicalView extends JFrame implements View { private JLabel input; private Timer model; public GraphicalView (Timer model, Controller controller) { setlayout (new GridLayout (2, 3)); this.model = model; // Your code here. //setup setDefaultcloseOperation(JFrame.EXIT_ON_CLOSE); setSize(700, 100); //display the window setVisible(true); } public void update () { input.setText(model.toString()); } } For this exercise, you will need to make an application that has a Graphical User Interface that represents a timer. Template files: Controller.java * Timer.java View.java TextView.java GraphicalView.java The Timer has 6 buttons, one that will increase and one that decreases hours, minutes and seconds respectively. To create the Timer, we will base ourselves on the Counter example. To adapt the code for the Counter to implement the Timer, we will need to change the model, the view, and the controller. Model: The previous model was the Counter; we need to replace this class with a new class Timer. The class Timer has: The previous model was the Counter; we need to replace this class with a new class Timer. The class Timer has: Three methods that increments the: hours, minutes and seconds respectively Three methods that decrements the: hours, minutes and seconds respectively Three methods that returns the: hours, minutes and seconds respectively o We also need to respect the 24 hours cycle: O The hours are between 0 and 23 o The minutes are between 0 and 59 The seconds are between O and 59 View You will need to modify the class GraphicalView so that it creates 6 buttons (to increment/decrements hours, minutes, and seconds) as well as a label (JLabel) that indicates the time. We will use a 2 (rows) by 3 (columns) grid to accommodate all these elements. Controller In the Controller, you will need to modify the method "public void actionPerformed ( ActionEvent e )" to accommodate the 6 actions launched by the 6 buttons of the GraphicalView and call the corresponding methods from Timer. Here is an example of the result: IncrementHours IncrementMinutes IncrementSeconds Timer 19:3:8 DecrementHours DecrementMinutes DecrementSeconds CAWindowsSystem32\cmd.exe-java App Timer e:0:e Timer 23:0:0 Timer 22:0:0 Timer 21:0:0 Timer 20:0:e Timer 19:0:e Timer 18:0:0 Timer 17:0:0 Timer 18:0:0 Timer 19:0:e Timer 18:59:0 Timer 18:58:0 Timer 18:59:0 Timer 19:0:0 Timer 19:1:0 Timer 19:2:0 Timer 19:3:0 Timer 19:2:59 Timer 19:3:0 Timer 19:2:59 import java.awt.event.*; public class Controller implements ActionListener { private Timer model; private View[) views; private int numberofviews; public Controller(){ views = new View[2]; numberofviews = 0; model = new Timer(); register(new GraphicalView(model, this)); register(new TextView(model)); update(); public Timer getModel () { return model; public View[] getviews () { return views; private void register(View view) { views[numberofviews] = view; numberofviews++; private void update() ( for (int i = 0; i numberofViews; i++) { views[i].update(); public void actionPerformed (ActionEvent e ) { private void register(View view) { views(numberofviews] - view; numberofviews++; } private void update() ( for (int i = 0; i numberofViews; i++) { views[i].update(); public void actionPerformed ( ActionEvent e ) { if(e.getActionCommand ().equals("IncrementHours")) { // Your code here. if(e.getActionCommand ().equals("IncrementMinutes")) { // Your code here. if(e.getActionCommand ().equals ("IncrementSeconds")) { // Your code here. if(e.getActionCommand ().equals ("DecrementHours")) { // Your code here. if(e.getActionCommand ().equals("DecrementMinutes")) { // Your code here. if(e.getActionCommand ().equals ("DecrementSeconds")) { // Your code here. update(); public class Timer { private int hours; private int minutes; private int seconds; public Timer(){ hours = 0; minutes = 0; seconds = e; public void incrementHours (){ // Your code here. public void decrementHours(){ // Your code here. public int getHours (){ return hours; public void incrementMinutes (O{ // Your code here. } public void decrementMinutes(){ // Your code here. public int getMinutes () { return minutes; public void incrementSeconds (){ // Your code here. public void decrementSeconds (){ public void incrementHours (( // Your code here. public void decrementHours ({ // Your code here. public int getHours (){ return hours; public void incrementMinutes(){ // Your code here. public void decrementMinutes (){ // Your code here. public int getMinutes () { return minutes; public void incrementSeconds (O // Your code here. public void decrementSeconds (){ // Your code here. public int getSeconds (){ return seconds; public String tostring () { return "Timer "+hours+":"+minutes+":"+seconds; } public interface View { void update () ; } public class TextView implements View { private Timer model ; public TextView (Timer model) { this.model = model ; } public void update() { System.out.println(model.toString()) ; } import java.awt.*; import java. awt.event.*; import javax.swing.*; public class GraphicalView extends JFrame implements View { private JLabel input; private Timer model; public GraphicalView (Timer model, Controller controller) { setlayout (new GridLayout (2, 3)); this.model = model; // Your code here. //setup setDefaultcloseOperation(JFrame.EXIT_ON_CLOSE); setSize(700, 100); //display the window setVisible(true); } public void update () { input.setText(model.toString()); } }
Step by Step Solution
★★★★★
3.51 Rating (158 Votes )
There are 3 Steps involved in it
Step: 1
TestGraphicalViewjava public class TestGraphicalView public static void mainString args Controller controller new Controller Controllerjava import javaawtevent public class Controller implements Actio...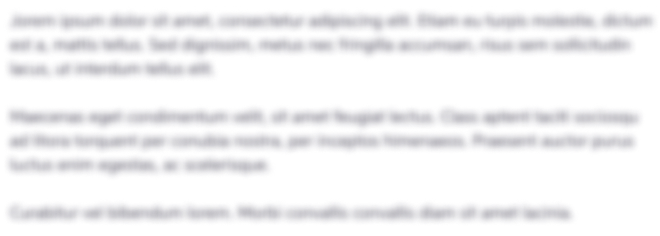
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started