Question
For this lab you will implement a phone book using a linked list to keep people's names and phone numbers organized in alphabetical order. The
For this lab you will implement a phone book using a linked list to keep people's names and phone numbers organized in alphabetical order. The structure of the list is already provided. Your job is to implement all methods defined in the provided Contact.h file.
You will need a method to insert a node at the right place in order to keep the list alphabetically sorted, a method to remove a contact from the list, a method to traverse the list to print all contacts, and also constructor and destructor. You can find implementations for those operations in the book and slides.
You can disregard duplicate names.
Methods insertContact() and deleteContact() should not ask for the contact to be inserted or deleted. This should be done in main, prior to calling the method that inserts or removes it.
Use the PhoneBook.cpp to test your class.
Only turn in contact.cpp. Make sure you don't change the Contact.h. Your Contact.cpp will need to compile with the Contact.h that I am providing.
EXTRA CREDIT OPTION (10 points, individual work only, no groups):
Add a member function to search for a specific contact given the name and return the phone number.
You will need to implement your own version of the main function in order to test the new function that you will add to the class. This change will involve asking the user for a contact's name and searching the list to retrieve the contact's phone number.
If you implement this extra credit option you will need to turn in the Contact.h and your updated version of the PhoneBook.cpp
/
#ifndef CONTACT_H_ #define CONTACT_H_
#include
class Contact { private: struct ContactNode { std::string name; std::string phoneNumber; ContactNode *next; }; ContactNode *head; public: Contact() { head = nullptr; } //ContactList(ContactList &obj) { } virtual ~Contact(); // destroys the list one node at a time void insertContact(std::string name, std::string phoneNumber); // do not allow duplicate names void deleteContact(std::string name); // deletes the corresponding node from the list void printContacts() const; // prints all contacts on the screen };
#endif /* CONTACT_H_ */
//============================================================================ // Name : PhoneBook.cpp // Author : // Version : // Copyright : Your copyright notice // Description : Program that tests the Contact class //============================================================================
#include
int main() { cout << "Phonebook application " << endl; Contact Phonebook; Phonebook.insertContact("Hellen", "222-333-4444"); cout << "************ Contacts after inserting Hellen *************" << endl; Phonebook.printContacts(); Phonebook.insertContact("Dani", "333-444-5555"); cout << "************ Contacts after inserting Dani *************" << endl; Phonebook.printContacts(); Phonebook.insertContact("Beth", "444-555-6666"); cout << "************ Contacts after inserting Beth *************" << endl; Phonebook.printContacts(); cout << "************ Contacts after inserting Zach *************" << endl; Phonebook.insertContact("Zach", "555-666-7777"); Phonebook.printContacts(); cout << "********************************************************" << endl; Phonebook.deleteContact("Dani"); cout << "************ Contacts after deleting Dani *************" << endl; Phonebook.printContacts(); Phonebook.deleteContact("Zach"); cout << "************ Contacts after deleting Zach *************" << endl; Phonebook.printContacts(); Phonebook.deleteContact("Beth"); cout << "************ Contacts after deleting Beth *************" << endl; Phonebook.printContacts(); Phonebook.deleteContact("Hellen"); cout << "************ Contacts after deleting Hellen *************" << endl; Phonebook.printContacts();
return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
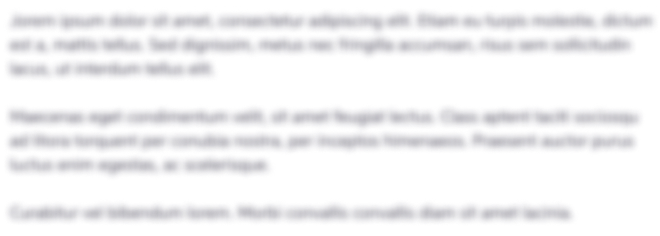
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started