Question
For this lab you will write a Java program that manipulates numbers. The program will ask the user to enter a binary number (or an
For this lab you will write a Java program that manipulates numbers. The program will ask the user to enter a binary number (or an empty line to quit) and then convert that number from base 2 into base 10 (decimal) and display the result. The program will loop until the user enters an empty line to end the program. See Project 08 for more discussion on binary numbers and their relationship to decimal numbers. For this assignment you must start with the following "skeleton" of Java code. Import this into your Eclipse workspace and fill in the methods as directed. Feel free to add any methods you find useful, but make sure that you add comments indicating what they do following the form of the rest of the comments in the code. Project09.java
Converting from Binary to Decimal
To convert from a binary to a decimal representation, your code will need to take into account the place of each digit in the binary string. One algorithm for performing this conversion is:
Start with a value of 0 for your decimal number
Assign a value n to be the power of the leftmost digit in your representation (HINT: this will be the length of the string - 1)
For each digit in your binary representation, working from left to right
Multiply the digit by your 2n
Add that result to your decimal total
Subtract 1 from n
Repeat until all digits have been processed
Here's an example of the number 1011 converted to a decimal number by applying the algorithm above
Start with result = 0, n = 3
At index 3
Multiply 1 by 23 = 8
result = 0 + 8 = 8
n = 2
At index 2
Multiply 0 by 22 = 0
result = 8 + 0 = 8
n = 1
At index 1
Multiply 1 by 21 = 2
result = 8 + 2 = 10
n = 0
At index 0
Multiply 1 by 20 = 1
result = 10 + 1 = 11
n = -1
Our final result is 11
Verify this by using your program from the previous project (or computing it by hand, using the algorithm given in the previous project).
Project 09 Sample Output
This is a sample transcript of what your program should do. Items in bold are user input and should not be put on the screen by your program. Converting from Binary to Decimal Enter a binary value (empty line to quit): 111011 The binary value 111011 is 59 in decimal (base 10). Enter a binary value (empty line to quit): 1101 The binary value 1101 is 13 in decimal (base 10). Enter a binary value (empty line to quit): 1011 The binary value 1011 is 11 in decimal (base 10). Enter a binary value (empty line to quit): 1d231 ERROR - invalid binary value Enter a binary value (empty line to quit): 10 The binary value 10 is 2 in decimal (base 10). Enter a binary value (empty line to quit): Goodbye Note that your output depends on the choices made by the user. You are required to check that the user has input a valid binary value (i.e. a string consisting only of 1s and 0s) or an empty line. See the project skeleton for more details. NOTE: For this assignment you will need to use the Character.getNumericValue() method. This method takes a character value such as '2' and converts it to the appropriate integer value (in this case the number 2). char input='2'; int inputInt = Character.getNumericValue(input); // inputInt now has a value of 2
skeleton code:
/** * Project09.java * * A program that converts binary numbers into decimal numbers. * Used to practice breaking code up into methods. * * @author YOUR NAME HERE */ package osu.cse1223; import java.util.Scanner; public class Project09 { public static void main(String[] args) { //TODO: Fill in body } /** * Given a Scanner as input, prompt the user to enter a binary value. Use * the function checkForBinaryValue below to make sure that the value * entered is actually a binary value and not junk. Then return the value * entered by the user as an String to the calling method. * * @param input * A scanner to take user input from * @return a String representing a binary value read from the user */ public static String promptForBinary(Scanner input) { // TODO: Fill in body } /** * Given a String as input, return true if the String represents a valid * binary value (i.e. contains only the digits 1 and 0). Returns false if * the String does not represent a binary value. * * @param value * A String value that may contain a binary value * @return true if the String value contains a binary value, false otherwise */ public static boolean checkForBinaryValue(String value) { // TODO: Fill in body } /** * Given a binary value, return an int value that is the base 10 * representation of that value. Your implementation must use the algorithm * described in the Project 9 write-up. Other algorithms will receive no * credit. * * @param value * A String containing a binary value to convert to integer * @return The base 10 integer value of that binary in the String */ public static int binaryToDecimal(String value) { // TODO: Fill in body } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
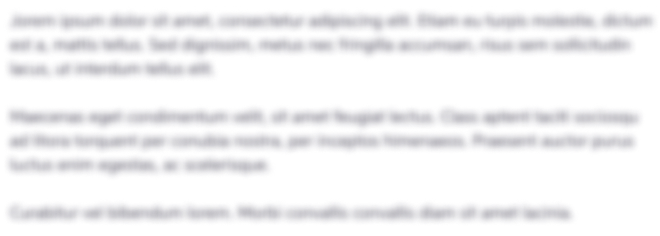
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started