Question
For this portion of the lab, you will reuse the program you wrote in Lab 5A Part 2 A. Redesign this solution in the following
For this portion of the lab, you will reuse the program you wrote in Lab 5A Part 2 A. Redesign this solution in the following manner:
Create a menu and ask the user which of the following conversions they wish to perform::
Miles to kilometer
Gallons to liters
Pounds to kilograms
Inches to centimeters
Fahrenheit to Celsius.
Your program must raise an exception if the user chooses any item not on the menu presented. Along with raising an exception, write the code to handle the exception.
Once the user has chosen a menu item he program should:
Ask the user for a value to convert. Refer to the input validations in Lab 4. Your program must raise an exception, and handle the exception, if an input error occurs.
Perform the conversion and write the original value, the original unit, the converted value, and the converted unit to an output file named conversions.txt.
Repeat steps a and b 10 times (in a loop).
####################################
LAB5APART2A.py
from conversions import *
def main(): """ Main function """ # Reading miles miles = float(input('William, how many miles do you want to convert to kilometers? ')) # Calling function kilometers = MilesToKm(miles) # If a valid value is provided, prints the result if kilometers != -1: print("The distance1 in kilometers is: ", kilometers) # Reading Fahrenheit value fahrenheit = float(input('William, what degrees fahrenheit do you want to convert to celsius? ')) # Calling function celsius = FahToCel(fahrenheit) # If a valid value is provided, prints the result if celsius != -1: print("The degrees in celsius is: ", celsius) # Reading gallons gallons = float(input('William, how many gallons do you want to convert to liters? ')) # Calling function liters = GalToLit(gallons) # If a valid value is provided, prints the result if liters != -1: print("The amount in liters is: ", liters) # Reading pounds pounds = float(input('William, how many pounds do you want to convert to kilograms? ')) # Calling function kilograms = PoundsToKg(pounds)
# If a valid value is provided, prints the result if kilograms != -1: print("The weight in kilograms is: ", kilograms) # Reading inches inches = float(input('William, how many inches do you want to convert to centimeters? ')) # Calling function centimeters = InchesToCm(inches) # If a valid value is provided, prints the result if centimeters != -1: print("The distance in centimeters is: ", centimeters) # Calling main main()
conversions.py (this is the code the main program is importing)
def MilesToKm(miles): counter = 0 while miles < 0: print("'ERROR - Negative Value [ENDING PROGRAM]") miles = float(input("Enter the correct value for miles ")) counter+=1
if counter > 2: break
if counter <= 2: kilometers= miles * 1.6 return kilometers else: print("Exceeded error count") return -1
def FahToCel(fahrenheit): counter1 = 0 while fahrenheit < 0: print("'ERROR - Negative Value [ENDING PROGRAM]") fahrenheit = float(input("Enter the correct value for fahrenheit ")) counter1+=1
if counter1 > 2: break
if counter1 <= 2: celsius = (fahrenheit - 32) * 5/9 return celsius
else: print("Exceeded error count") return -1
def GalToLit(gallons): counter2 = 0 while gallons < 0: print("'ERROR - Negative Value [ENDING PROGRAM]") gallons = float(input("Enter the correct value for gallons ")) counter2+=1
if counter2 > 2: break
if counter2 <= 2: liters = gallons * 3.9 return liters else: print("Exceeded error count") return -1 def PoundsToKg(pounds): counter3 = 0 while pounds < 0: print("'ERROR - Negative Value [ENDING PROGRAM]") pounds = float(input("Enter the correct value for pounds ")) counter3+=1
if counter3 > 2: break
if counter3 <= 2: kilograms = pounds * 0.45 return kilograms else: print("Exceeded error count")
return -1 def InchesToCm(inches): counter4 = 0 while inches < 0: print("'ERROR - Negative Value [ENDING PROGRAM]") inches = float(input("Enter the correct value for miles ")) counter4+=1
if counter4 > 2: break
if counter4 <= 2: centimeters = inches * 2.54 return centimeters else: print("Exceeded error count") return -1
#####################################
Python program, please show proper indentation. Thamls!!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
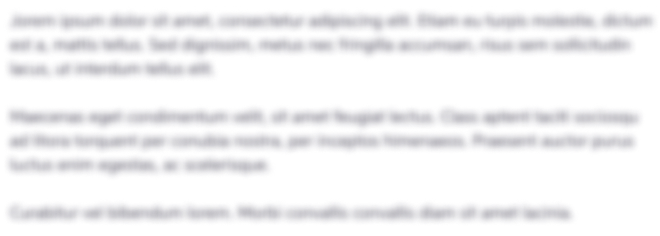
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started