Question
For this problem a 2d array of ints represents the value of each block in a city. Each element in the array is a city
For this problem a 2d array of ints represents the value of each block in a city. Each element in the array is a city block. The value of a block could be negative indicating the block is a liability to own. Create a method that finds the value of the most valuable contiguous sub rectangle in the city represented by the 2d array. The sub rectangle must be at least 1 by 1. (If all the values are negative "the most valuable" rectangle would be the negative value closest to 0.) Note, for this method you may assume the given 2d array of ints will not cause your method to overflow or underflow the Java int data type.
Consider the following example. The 2d array of ints has 6 rows and 5 columns per row, representing an area of the city. The cells with the white background and underlined represent the most valuable contiguous sub rectangle in the given array. (Value of 15.)
Here is another example with the almost same 2D array with a single change. The value of the block at row 4, column 2 has been changed from 1 to 6. Given that configuration the most valuable contiguous sub rectangle in the given array has a value of 17 and is the cells with the white background and underlined.
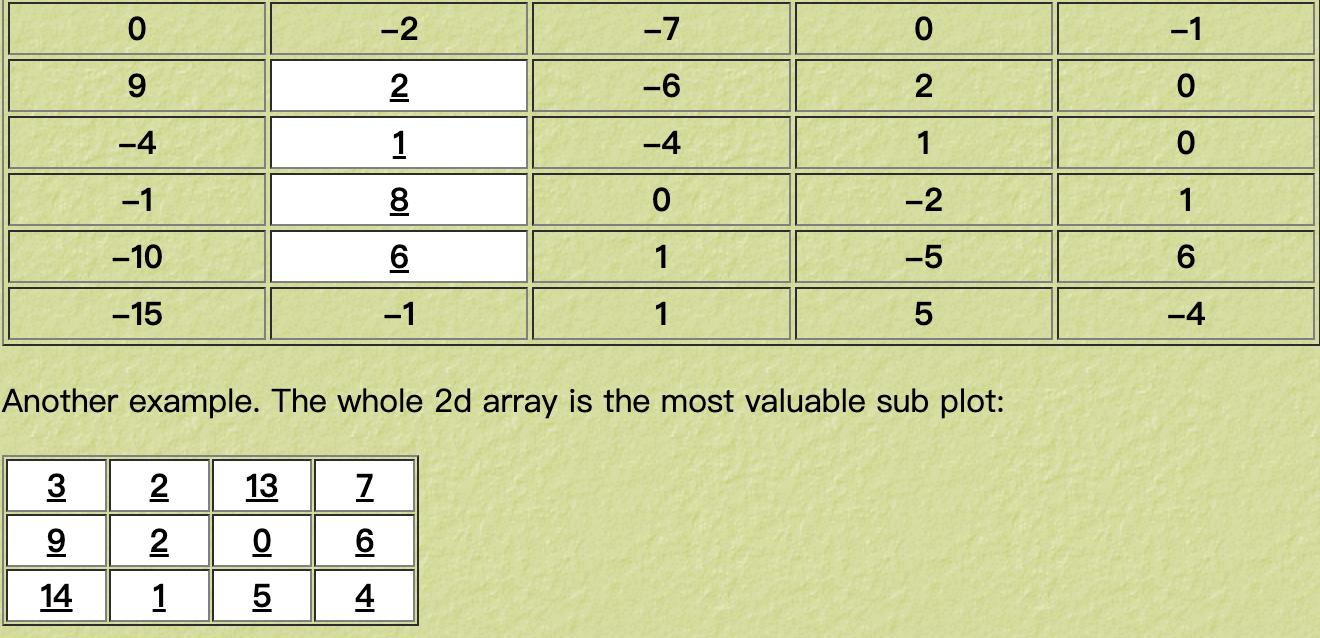
Here is the starter code
Here is the starter code
/**
* Given a 2D array of ints return the value of the
* most valuable contiguous sub rectangle in the 2D array.
* The sub rectangle must be at least 1 by 1.
*
pre: mat != null, mat.length > 0, mat[0].length > 0,
* mat is a rectangular matrix.
*
post: return the value of the most valuable contiguous sub rectangle
* in city.
* @param city The 2D array of ints representing the value of
* each block in a portion of a city.
* @return return the value of the most valuable contiguous sub rectangle
* in city.
*/
public static int getValueOfMostValuablePlot(int[][] city) {
// check preconditions
if (city == null || city.length == 0 || city[0].length == 0
|| !isRectangular(city) ) {
throw new IllegalArgumentException("Violation of precondition: " +
"getValueOfMostValuablePlot. The parameter may not be null," +
" must value at least one row and at least" +
" one column, and must be rectangular.");
}
}
/*
* pre: mat != null, mat.length > 0
* post: return true if mat is rectangular
*/
private static boolean isRectangular(int[][] mat) {
// check preconditions
if (mat == null || mat.length == 0) {
throw new IllegalArgumentException("Violation of precondition: " +
"isRectangular. Parameter may not be null and must contain" +
" at least one row.");
}
boolean correct = mat[0] != null;
int row = 0;
while(correct && row < mat.length) {
correct = (mat[row] != null)
&& (mat[row].length == mat[0].length);
row++;
}
return correct;
}
Here is some samples to test
city = new int[][] { { 1, 2, 3, 4, 5, 6, 7 } };
expected = 28;
city = new int[][] { {-10, -10, -10, -5},
{-15, -15, -10, -10},
{-5, -10, -20, -5},
{-5, -5, -10, -20 }};
expected = -5;
9 -4 -1 -10 -15 -2 2 1 8 1 -1 -7 -6 -4 1 1 2 1 -2 -5 5 -1 1 6 -4
Step by Step Solution
3.41 Rating (154 Votes )
There are 3 Steps involved in it
Step: 1
Here is the completed code for the getValueOfMostValuablePlot method java public static int getValue...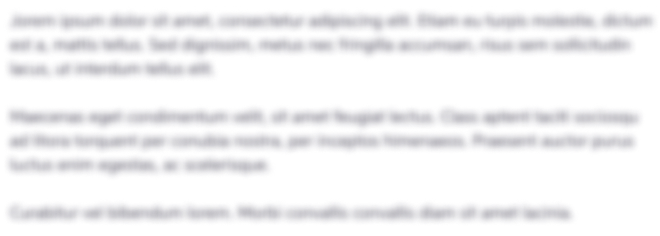
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started