Question
For this programming project, you will be coding a simple, turn based RPG Game, similar to Final Fantasy or Pokemon. The game will begin with
For this programming project, you will be coding a simple, turn based RPG Game, similar to Final Fantasy or Pokemon. The game will begin with a player character fighting an enemy character. The game is won when the player character defeats the computer-controlled character. This assignment is meant to be creative, so feel free to name / theme your game however you like.
Here we will be building an RPG "game engine" of sorts that we will use for future projects later on in this course.
Character Class
Each character will be represented by a Character C++ Class. Each character, both the player and the "computer" will have the following properties:
- Name, so the characters have some way to identify which one is which
- Strength, to represent how hard they can hit
- Health, to represent how much damage they can take before dying
- Defense, to reduce incoming damage
- Speed, to determine which character will attack first
Custom RPG Mechanics
Using the described character classes, each turn the characters take turns in combat. Combat follows the following rules:
- Each turn the characters will deal damage to each other
- The character with higher "speed" will deal damager first
- A character will deal damage based on how strong they are
- Characters can reduce incoming damage based on how tough they are
- damage taken = attacker's strength - defender's defense
- Characters are defeated when their health reaches 0
Requirements
Using the mechanics described above, create a combat program that has the following requirements:
- (20 pts) Two characters should take turns attacking each other until one runs out of health
- (20 pts) Once one character runs out of health the game ends
- (20 pts) Characters should be represented by a Character class
- (20 pts) The character class should not utilize any public member variables
- (20 pts) The character class should have an overloaded constructor for setting initial values
- Bonus! (20 pts) Add an option for the player character, such as run away or use a healing potion. How this extra option is used is up to you.
Note: Some students have asked why we have an "if" condition evaluating speed if it's going to be the same for the entire execution of the code. The reason is if you were to randomize speed values or decide to change it, the code will react appropriately to these changes.
******************************************************
Current Code:
main.cpp
character.h
character.cpp
Step by Step Solution
There are 3 Steps involved in it
Step: 1
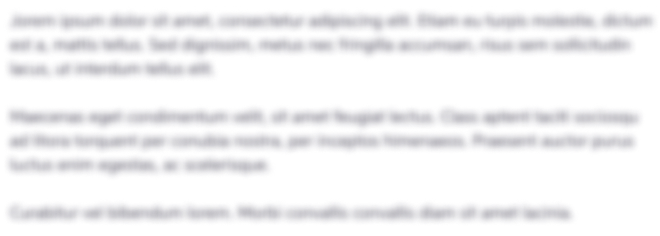
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started