Question
For today's lab we will be completing Exercise P8.1 from the book with some slight modifications. Starter code is posted on Google Drive in two
For today's lab we will be completing Exercise P8.1 from the book with some slight modifications. Starter code is posted on Google Drive in two files ComboLock.java and ComboLockTest.java.
Follow the directions given for P8.1, and fill in the gaps in the starter code . More details are given inside the comments for the ComboLock class.
Here is sample output of an interaction with the program.
Please enter 3 values for the new combo lock: 12 12 12
Combo is: 12 12 12
Current Number: 0
Enter number of ticks to turn to the right 0 - 40. Enter an invalid number to quit (negative, or >40).
28
Current Number: 12
Enter number of ticks to turn to the left 0 - 40. Enter an invalid number to quit (negative, or >40).
40
Current Number: 12
Enter number of ticks to turn to the right 0 - 40. Enter an invalid number to quit (negative, or >40).
40
You opened the lock!
Would you like to run simulation again? (Yes or No)
y
Please enter 3 values for the new combo lock: 12 13 14
Combo is: 12 13 14
Current Number: 0
Enter number of ticks to turn to the right 0 - 40. Enter an invalid number to quit (negative, or >40).
28
Current Number: 12
Enter number of ticks to turn to the left 0 - 40. Enter an invalid number to quit (negative, or >40).
1
Current Number: 13
Enter number of ticks to turn to the right 0 - 40. Enter an invalid number to quit (negative, or >40).
39
You opened the lock!
Would you like to run simulation again? (Yes or No)
y
Please enter 3 values for the new combo lock: 1 2 3
Combo is: 1 2 3
Current Number: 0
Enter number of ticks to turn to the right 0 - 40. Enter an invalid number to quit (negative, or >40).
39
Current Number: 1
Enter number of ticks to turn to the left 0 - 40. Enter an invalid number to quit (negative, or >40).
3
Current Number: 4
Enter number of ticks to turn to the right 0 - 40. Enter an invalid number to quit (negative, or >40).
3
Current Number: 1
Enter number of ticks to turn to the left 0 - 40. Enter an invalid number to quit (negative, or >40).
3
Current Number: 4
Enter number of ticks to turn to the right 0 - 40. Enter an invalid number to quit (negative, or >40).
333
Invalid entry. The program will now exit.
Some things to note are that an entry of 40 will spin the lock all the way back around to the same number you are on. Our ComboLockTest class will verify that values sent to the turnLeft() and turnRight() methods receive a value between 0 40 (inclusive).
ComboLockTest is not written with a lot of error checking. This is ok because we are simply using it as a testing class. So if you feed bad input to ComboLockTest this may cause an exception. (i.e. entering a double value instead of an int while scanning for input). You just need to be careful that you use valid inputs and if you cause an exception be aware of what caused it.
Notice what happens when a user spins the lock right, then right, then right. Also assume our combination is 1,3,5.
myLock.turnRight(39); //spins once to the right, OK! First Value locked in!
myLock.turnRight(3); //spins the dial and updates value, but now we have //wiped out our initial good entry,
//looking for a right spin to 1 again now.
myLock.turnRight(37); //spins the dial and updates value, but the middle //spin reset the tumblers.
This implies that you will need to use an instance variable to keep track of what state your lock is in
SAMPLE CODE:
/** A class to simulate a combination lock. */ public class ComboLock { //********* you will need to create appropriate instance variables here private int currentNumber = 0; //current value lock dial is set to //more variables here .... /** Initializes the combination of the lock. */ //**** COMPLETE THIS CONSTRUCTOR - input should be 3 number combination //**** You may need to set other instance variables other than the //**** arguments here //You should verify that the secret number are in the range 0-39 (inclusive) //if the values given are not in that range, clamp them. //i.e. the call new ComboLock(0, -20, 45) would create a combination of // 0, 0, 39 (the -20 gets clamped to 0 because it was less than 0) // (the 45 gets clamped to 39 because it was > 39). public ComboLock(int secret1, int secret2, int secret3) { //fill in } /** Resets the state of the lock so that it can be opened again. */ //********* COMPLETE THIS METHOD public void reset() { } /** Turns lock left given number of ticks. @param ticks number of ticks to turn left */ //*********COMPLETE THIS METHOD //you can assume that ticks will be a valid value between 0-40 //note that 40 ticks in either direction should return us back to the //number we started on public void turnLeft(int ticks) { } /** Turns lock right given number of ticks @param ticks number of ticks to turn right */ //*********COMPLETE THIS METHOD //you can assume that ticks will be a valid value between 0-40 //note that 40 ticks in either direction should return us back to the //number we started on public void turnRight(int ticks) { } /** Returns true if the lock can be opened now @return true if lock is in open state */ //**** COMPLETE THIS METHOD public boolean open() { return false; //dummy value for now } /** Returns current value dial is pointing at @return value dial is pointing at currently */ public int getCurrentNumber() { return currentNumber; } }
TESTER CODE: Here is an alternate tester you can use to test your ComboLock class. The percentage shown is not your grade, it's just the percentage of the 11 tests that you got correct.
public static void main(String[] args) { /*ComboLock lock1 = new ComboLock(0, 0, 0); //**** Test #1 // do first test R-L-R lock1.turnRight(40); lock1.turnRight(40); lock1.turnLeft(40); lock1.turnRight(40); if(lock1.open()) { System.out.println("OPEN!"); } else { System.out.println("CLOSED!"); } */ ComboLock cl = new ComboLock(1,3,5); cl.turnRight(39); System.out.println(cl.getCurrentNumber()); cl.turnRight(3); System.out.println(cl.getCurrentNumber()); cl.turnRight(37); System.out.println(cl.getCurrentNumber()); cl = new ComboLock(0,0,0); cl.turnRight(0); cl.turnLeft(0); cl.turnRight(0); System.out.println(cl.open()); double correct = 0; //tracks correct answers double tests = 0; //tracks total possible points // create a new lock with the combo shown as parameter ComboLock lock = new ComboLock(12, 12, 12); //**** Test #1 // do first test R-L-R lock.turnRight(28); lock.turnLeft(40); lock.turnRight(40); if (lock.open()) correct++; else System.out.println("Failed #1"); tests++; System.out.println("Lock is open: " + lock.open()); System.out.println("Expected: true"); // Next Test #2 lock = new ComboLock(12, 13, 14); lock.turnRight(28); lock.turnLeft(1); lock.turnRight(39); if (lock.open()) correct++; else System.out.println("Failed #2"); tests++; System.out.println("Lock is open: " + lock.open()); System.out.println("Expected: true"); // Next Test #3 lock = new ComboLock(1, 2, 3); lock.turnRight(39); lock.turnLeft(3); lock.turnRight(3); lock.turnLeft(3); lock.turnRight(3); if (!lock.open()) correct++; else System.out.println("Failed #3"); tests++; System.out.println("Lock is open: " + lock.open()); System.out.println("Expected: false"); // Next Test #4 lock = new ComboLock(0, 0, 0); lock.turnRight(40); lock.turnLeft(40); lock.turnRight(40); if (lock.open()) correct++; else System.out.println("Failed #4"); tests++; System.out.println("Lock is open: " + lock.open()); System.out.println("Expected: true"); // Next Test #5 lock = new ComboLock(39, 39, 39); lock.turnRight(1); lock.turnLeft(40); lock.turnRight(40); if (lock.open()) correct++; else System.out.println("Failed #5"); tests++; System.out.println("Lock is open: " + lock.open()); System.out.println("Expected: true"); // Next Test #6 lock = new ComboLock(39, 39, 39); lock.turnRight(1); lock.turnLeft(1); lock.turnRight(1); if (!lock.open()) correct++; else System.out.println("Failed #6"); tests++; System.out.println("Lock is open: " + lock.open()); System.out.println("Expected: false"); // Next Test #7 lock = new ComboLock(39, 39, 39); lock.turnRight(1); lock.turnLeft(40); lock.turnRight(3); if (!lock.open()) correct++; else System.out.println("Failed #7"); tests++; System.out.println("Lock is open: " + lock.open()); System.out.println("Expected: false"); // Next Test #8 lock = new ComboLock(39, 39, 39); lock.turnRight(1); // current 39 lock.turnLeft(1); // current 0 - should reset to state 0 now. lock.turnRight(1); // 39 lock.turnLeft(40); // 39 lock.turnRight(40); // 39 if (lock.open()) correct++; else System.out.println("Failed #8"); tests++; System.out.println("Lock is open: " + lock.open()); System.out.println("Expected: true"); // Next Test #9 lock = new ComboLock(23, 32, 13); lock.turnRight(17); // 23 lock.turnLeft(9); // 32 lock.turnRight(19); // 13 if (lock.open()) correct++; else System.out.println("Failed #9"); tests++; System.out.println("Lock is open: " + lock.open()); System.out.println("Expected: true"); // Next Test #10 lock = new ComboLock(23, 32, 13); lock.turnRight(17); // 23 lock.turnLeft(9); // 32 lock.turnRight(1); // reset to state 0 on 31 now if (!lock.open()) correct++; else System.out.println("Failed #10"); tests++; lock.turnLeft(1); // on 32 now lock.turnRight(9); // 23 lock.turnLeft(9); // 32 lock.turnRight(19); // 13 //#11 if (lock.open()) correct++; else System.out.println("Failed #11"); tests++; System.out.println("Lock is open: " + lock.open()); System.out.println("Expected: true"); System.out.println(); System.out.println("Final Score: " + correct + "/" + tests + " = " + 100 * correct / tests); }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
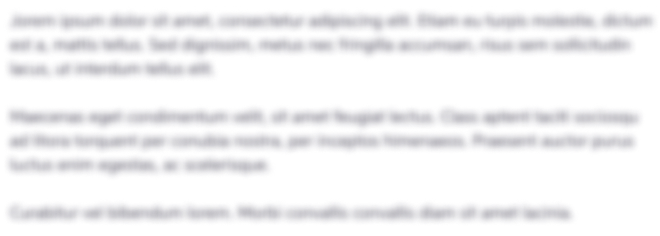
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started