Question
For your lab, you will create a program to display a yearly calendar, using a three-dimensional array to represent the calendar itself. Your array should
For your lab, you will create a program to display a yearly calendar, using a three-dimensional array to represent the calendar itself. Your array should use a standard size of 6 by 7 by 12, representing weeks, days, and months, respectively.
Your program should begin by calling an initialization function which asks the user to enter the four-digit calendar year (e.g. 2020), and then prompts them to select what day January 1st of that year was (e.g. Wednesday for 2020). This can be seen demonstrated in the screenshot on the right.
With this information, you should then initialize the calendar. The day of January 1st will let you know where you should start numbering from - the first few locations may be left blank if the year did not begin on a Sunday. The other thing you must consider is whether or not the year is a leap year, which will determine whether February has 28 or 29 days. To check if it is a leap year or not, remember that a leap year is any that:
- Is evenly divisible by 4
- Is NOT evenly divisible by 100, unless
- It is also evenly divisible by 400.
After verifying whether the year is a leap year or not, and properly initializing the calendar, the main program loop (in the main function) should prompt the user to view the calendar for any given month. This menu can be seen in the screenshot on the right.
Selecting any given month will call a separate function to display that months calendar in a nicely formatted manner, as can be seen for January and February of 2020 in the screenshots below.
*Do not use pushback function. Do not use #include
*Must be written using C++*
THIS IS MY CODE SO FAR, PLEASE UTILIZE WHAT IS WRITTEN CURRENTLY:
#include "stdafx.h" #include
using namespace std;
int getDaysInMonth(int year, int month); int getMonth(); void displayMonthSelMenu();
int main() { int calYear, dayPos, month, daysInMonth;
cout > calYear; cout > dayPos; cout
do { displayMonthSelMenu(); month = getMonth(); daysInMonth = getDaysInMonth(calYear, month); if (month != 13) { cout
return 0; }
/*displayMonthSelMenu function*/ void displayMonthSelMenu() { //system("cls"); // Clear the screen cout
}
/*getMonth function*/ int getMonth() { int month;
cin >> month; while (month 13) { cout > month; } return month; }
/*getDaysInMonth function*/
int getDaysInMonth(int year, int month) { //if statement for calendar days in month of february known to have either 28 days or 29 days depending on leap year if (month == 2) { if ((year % 400 == 0) || (year % 4 == 0 && year % 100 != 0)) //check if calendar year is leap year return 29; // return 29 calendar days in February if it is a leap year else return 28; // return 28 calendar days in February if it is not a leap year } //Else if statement for known months of year with 31 calendar days else if (month == 1 || month == 3 || month == 5 || month == 7 || month == 8 || month == 10 || month == 12) return 31; //Else statement for all other months (4, 6, 9, 11) known to have 30 calendar days else return 30; // return 30 calendar days }
Your Program For your lab, you will create a program to display a yearly calendar, using a three-dimensional array to represent the calendar itself. Your array should use a standard size of 6 by 7 by 12, representing weeks, days, and months, respectively. Your program should begin by calling an Welcome to the Calendar Utility initialization function which asks the user to What year would you like to build a calendar for? enter the four-digit calendar year (e.g. 2020), Please enter the four-digit year: 2020 and then prompts them to select what day January 1* of that year was (e.g. Wednesday January 1st of 2020 was a... 1) Sunday for 2020). This can be seen demonstrated in 2) Monday the screenshot on the right. 3) Tuesday 4) Wednesday With this information, you should then 5) Thursday initialize the calendar. The day of January 1* 6) Friday 7) Saturday will let you know where you should start numbering from - the first few locations may be left blank if the year did not begin on a Sunday. The other thing you must consider is whether or not the year is a leap year, which will determine whether February has 28 or 29 days. To check if it is a leap year or not, remember that a leap year is any that: View Monthly Calendars Is evenly divisible by 4 2) February Is NOT evenly divisible by 100, unless... 4) April It is also evenly divisible by 400. After verifying whether the year is a leap year or not, and properly 9) September initializing the calendar, the main program loop (in the main function) should prompt the user to view the calendar for any given month. This menu can be seen in the screenshot on the right. 1) January 3) March 5) May 6) June 7) July 8) August 10) October 11) November 12) December 13) Exit Selecting any given month will call a separate function to display that month's calendar in a nicely formatted manner, as can be seen for January and February of 2020 in the screenshots below. TW Th F SIM TW Th F Sa Sa -- --- -- 1 2 3 4 1 --- 5 6 7 00 9 10 11 2 3 4 | 5 6 7 00 --- 12 13 14 15 16 17 18 9 10 11 12 13 14 15 19 20 21 22 23 24 25 16 17 18 19 20 21 22 26 27 | 28 29 30 31 23 24 25 26 27 28 29 1 Press any key to continue Press any key to continue
Step by Step Solution
There are 3 Steps involved in it
Step: 1
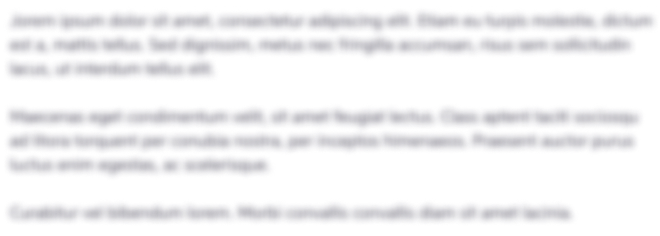
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started