Question
For your third programming assignment you will be implementing the following two functions in a C++ program: string addbin(string, string); // adds two binary numbers
For your third programming assignment you will be implementing the following two functions in a C++ program:
string addbin(string, string); // adds two binary numbers together and returns the sum
string addhex(string, string); // adds two hexadecimal numbers together and returns the sum
NOTE: In some cases, you may need to sign-extend a number when doing addition. For example, when you add two binary/hexadecimal numbers, the two numbers need to be the same length. So if you are trying to do the addition:
A1FC + B You need to actually make it A1FC + 000B
After doing that you can add the numbers column-by-column, starting at the right end (i.e., C + B first).
You need to have a variable to maintain the carry. Each time you add the numbers in a column, it is possible that a carry will be generated, which means a 1 is carried into the next column to the left. The carry variable should always have a value of 0 or 1.
Treat the numbers as UNsigned numbers, so you can sign-extend a binary/hexadecimal number by simply adding the appropriate number of 0s to the left.
As another example, the addition 1 + ABCD would need to be 0001 + ABCD
Since binary/hexadecimal numbers are represented as strings, this means you need to concatenate the character 0 with the string (possibly several times) to do the sign-extension.
Also, if there is a carry out of the most significant bit (MSB), make sure to include that in your answer; otherwise it will be incorrect. So the sum string can be larger than the two input strings. For example, binary 1 + 1 = 10, because 1+1=0, and you carry 1 over to the next column.
Also remember the formula we learned in class to recalculate the sum when the sum is larger than the base of the number system: sum = sum mod base sum = sum mod 2 (for binary) sum = sum mod 16 (for hexadecimal)
Do you see why the formula works for both numbering systems? Make sure you are comfortable with it as it will appear in quizzes and tests.
NOTE: The numbers need to be added directly in their hexadecimal or binary format. For example, it is NOT allowed to convert the numbers to decimal first,
add the decimal values together, and then convert the result back to binary or hexadecimal. Your functions for adding binary and hexadecimal numbers should work exactly the same as the algorithm we used in class for adding binary and hexadecimal numbers together by hand.
Sample Run:
Here is a sample run of the program. After you finish implementing the two functions, run the program with these test cases (which Ive provided to you in the 260_assign3.cpp file) and make sure you get the same results. The test cases also show you how to properly call each function. Feel free to come up with and run your own test cases as well.
DocViewer
Page
of 2
Pages
// NOTE: The ONLY files that should be #included for this assignment are iostream,
vector, and string
// No other files should be #included
#include
#include
#include
// NOTE: The ONLY files that should be #included for this assignment are iostream,
vector, and string
// No other files should be #included
using namespace std;
string addbin(string, string);
string addhex(string, string);
int main()
{
cout<<"binary 1101 + 1000 = "< get 10101 cout<<"binary 11000 + 1011 = "< get 100011 cout<<"binary 11111111 + 1 = "< get 100000000 cout<<"binary 101010 + 10 = "< should get 101100 cout<<"hexadecimal A4 + A5 = "< 149 cout<<"hexadecimal 2B + C = "< 37 cout<<"hexadecimal FABC + 789 = "< get 10245 cout<<"hexadecimal FFFFFF + FF = "< should get 10000FE system("PAUSE"); return 0; } string addbin(string bin1, string bin2) { //IMPLEMENT THIS FUNCTION //IMPLEMENT THIS FUNCTION //IMPLEMENT THIS FUNCTION } string addhex(string hex1, string hex2) { //IMPLEMENT THIS FUNCTION //IMPLEMENT THIS FUNCTION //IMPLEMENT THIS FUNCTION } Annotations
Step by Step Solution
There are 3 Steps involved in it
Step: 1
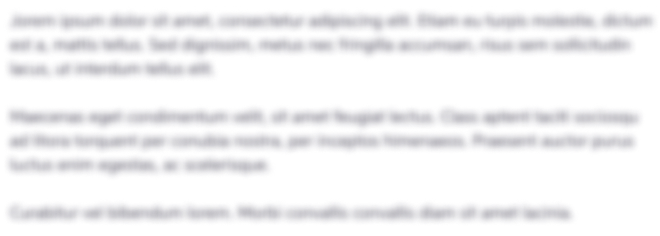
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started