Question
Forward propagation is simply the summation of the previous layer's output multiplied by the weight of each wire, while back-propagation works by computing the partial
Forward propagation is simply the summation of the previous layer's output multiplied by the weight of each wire, while back-propagation works by computing the partial derivatives of the cost function with respect to every weight or bias in the network. In back propagation, the network gets better at minimizing the error and predicting the output of the data being used for training by incrementally updating their weights and biases using stochastic gradient descent.
We are trying to estimate a continuous-valued function, thus we will use squared loss as our cost function and an identity function as the output activation function. f(x) is the activation function that is called on the input to our final layer output node, and a^ is the predicted value, while y is the actual value of the input.
C=12(ya^)2 | (6.1) |
f(x)=x | (6.2) |
When you're done implementing the function train (below and in your local repository), run the script and see if the errors are decreasing. If your errors are all under 0.15 after the last training iteration then you have implemented the neural network training correctly.
You'll notice that the train functin inherits from NeuralNetworkBase in the codebox below; this is done for grading purposes. In your local code, you implement the function directly in your Neural Network class all in one file. The rest of the code in NeuralNetworkBase is the same as in the original NeuralNetwork class you have locally.
In this problem, you will see the network weights are initialized to 1. This is a bad setting in practice, but we do so for simplicity and grading here.
You will be working in the file part2-nn/neural_nets.py in this problem
Implementing Train
Available Functions: You have access to the NumPy python library as np, rectified_linear_unit, output_layer_activation, rectified_linear_unit_derivative, and output_layer_activation_derivative
Note: Functions rectified_linear_unit, rectified_linear_unit_derivative, and output_layer_activation_derivative can only handle scalar input. You will need to use np.vectorize to use them.
class NeuralNetwork(NeuralNetworkBase):
def train(self, x1, x2, y):
### Forward propagation ### input_values = np.matrix([[x1],[x2]]) # 2 by 1
# Calculate the input and activation of the hidden layer hidden_layer_weighted_input = # TODO (3 by 1 matrix) hidden_layer_activation = # TODO (3 by 1 matrix)
output = # TODO activated_output = # TODO
### Backpropagation ###
# Compute gradients output_layer_error = # TODO hidden_layer_error = # TODO (3 by 1 matrix)
bias_gradients = # TODO hidden_to_output_weight_gradients = # TODO input_to_hidden_weight_gradients = # TODO
# Use gradients to adjust weights and biases using gradient descent self.biases = # TODO self.input_to_hidden_weights = # TODO self.hidden_to_output_weights = # TODO
Step by Step Solution
There are 3 Steps involved in it
Step: 1
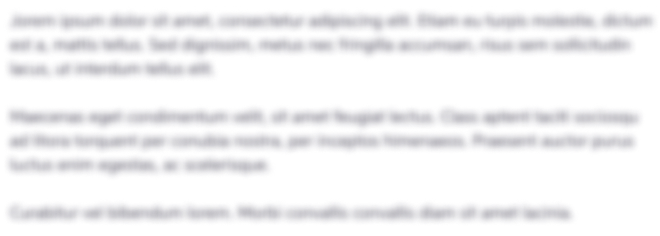
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started