Question
Foundations Of Computer Science (COP 3014) The files will be at the bottom of the page. The cryptoaccount.cpp, cryptoaccount.h, and the cryptoaccountmain.cpp files are in
Foundations Of Computer Science (COP 3014)
The files will be at the bottom of the page. The cryptoaccount.cpp, cryptoaccount.h, and the cryptoaccountmain.cpp files are in C++ format, they need to be converted to a CLASS format using the guidelines below.
The cryptobroker.cpp file is as follows:
/*
* The program implements and shows how to use following functions
* Sell coins: let us say that somebody else has provided you with a C function:
* bool sell(double qty);
* Buy coin: The function available to you is bool buy(double qty);
* Check Price: double checkPrice() Will return the current market price of the currency.
*/
#include "cryptobroker.h"
#include
double sell(double quantity){
return quantity * currentPrice;
}
double buy(double quantity){
return quantity * currentPrice;
}
double checkPrice(){
//sleep(1);
//srand(time(NULL));
return currentPrice = 1000 + (0.5 - ((double) rand())/RAND_MAX)*RANGE;
}
void print(){
cout
}
void test()
{
// for(int i = 0; i
// checkPrice();
// print();
//}
double soldAmount;
double boughtAmount;
cout
soldAmount = sell(1.0);
cout
cout
boughtAmount = buy(1.0);
cout
}
The cryptobroker.h file is as follows:
/* * The program implements and shows how to use following functions * Sell coins: let us say that somebody else has provided you with a C function: * bool sell(double qty); * Buy coin: The function available to you is bool buy(double qty); * Check Price: double checkPrice() Will return the current market price of the currency. */ #ifndef CRYPTOBROKER_H #define CRYPTOBROKER_H
#include
#define RANGE 300
using namespace std;
extern double currentPrice;
double sell(double quantity); //Returns the $value of the coins sold. double buy(double quantity); //Returns the $value of the coins bought. double checkPrice(); //Returns the current value of one coin. void print(); void test();
#endif
The cryptobrokermain.cpp file is as follows:
/*
* The program implements and shows how to use following functions
* Sell coins: let us say that somebody else has provided you with a C function:
* bool sell(double qty);
* Buy coin: The function available to you is bool buy(double qty);
* Check Price: double checkPrice() Will return the current market price of the currency.
*/
#include "cryptobroker.h"
double currentPrice = 0;
int main()
{
test();
return 0;
}
The cryptoaccount.cpp file is as follows (This needs to be turned into a Class):
/*
* The program implements and shows how to use following functions
* Sell coins: let us say that somebody else has provided you with a C function:
* bool sell(double qty);
* Buy coin: The function available to you is bool buy(double qty);
* Check Price: double checkPrice() Will return the current market price of the currency.
*/
#include "cryptobroker.h"
#include
#define COIN_PRICE 9063.79
using namespace std;
// getting the user choice for trade and return it
int getUserOption()
{
int input = 0;
cout
cout
cout
cout
cout
cout
cout
cin >> input;
return input;
}
// Asking for initinal deposit from user
double getInitialDepositFromUser()
{
double deposit = 0;
cout
cin >> deposit;
return deposit;
}
// Top level function called by main
void process()
{
int input = 1;
// getting initial deposit
double deposit = getInitialDepositFromUser();
Account *account = new Account();
// initialize Account
initialize(account, deposit);
print(account);
while (input != 0)
{
input = getUserOption();
if (input == 1)
{
cout
double qty = 0;
cin >> qty;
if (!buyMore(account, qty))
{
cout
}
}
else if (input == 2)
{
cout
double qty = 0;
cin >> qty;
if (!sellSome(account, qty))
{
cout
}
}
else if (input == 3)
{
print(account);
}
else if (input == 0)
{
break;
}
else
{
cout
}
}
}
double sell(double quantity) {
return quantity * currentPrice;
}
double buy(double quantity) {
return quantity * currentPrice;
}
double checkPrice() {
return currentPrice = COIN_PRICE + (0.5 - ((double)rand()) / RAND_MAX)*RANGE;
}
void print(Account *account)
{
cout balance coins
balance + (account->coins*checkPrice()))
}
// Here we are buying coins if we have sufficent coins
bool sellSome(Account *account, double coins)
{
if (coins > account->coins)
return false;
account->coins = account->coins - coins;
account->balance = account->balance + sell(coins);
return true;
}
// Here we are buying coins if we have sufficent balance
bool buyMore(Account *account, double coins)
{
double price = buy(coins);
if (price > account->balance || coins == 0)
return false;
account->coins = account->coins + coins;
account->balance = account->balance - price;
return true;
}
// initialize Account with the balance provided
void initialize(Account *account, double deposit)
{
account->balance = deposit;
account->coins = 0;
}
void test(Account *account)
{
cout
cout
checkPrice();
initialize(account, 5000);
cout
cout
buyMore(account, .1);
print(account);
cout
cout
buyMore(account, .3);
print(account);
cout
cout
sellSome(account, .2);
print(account);
cout
cout
sellSome(account, .02);
print(account);
}
The cryptoaccount.h file is as follows (This needs to be turned into a Class):
/*
* The program implements and shows how to use following functions
* Sell coins: let us say that somebody else has provided you with a C function:
* bool sell(double qty);
* Buy coin: The function available to you is bool buy(double qty);
* Check Price: double checkPrice() Will return the current market price of the currency.
*/
#ifndef CRYPTOBROKER_H
#define CRYPTOBROKER_H
#include
#include
#include
#define RANGE 300
using namespace std;
extern double currentPrice;
typedef struct
{
double balance;
double coins;
} Account;
double sell(double quantity); //Returns the $value of the coins sold.
double buy(double quantity); //Returns the $value of the coins bought.
double checkPrice(); //Returns the current value of one coin.
void initialize(Account *account, double deposit);
bool buyMore(Account *account, double coins);
bool sellSome(Account *account, double coins);
void print(Account *account);
int getUserOption();
double getInitialDepositFromUser();
void process();
void test(Account *account);
#endif
The cryptoaccountmain.cpp file is as follows (This needs to be turned into a Class):
/*
* The program implements and shows how to use following functions
* Sell coins: let us say that somebody else has provided you with a C function:
* bool sell(double qty);
* Buy coin: The function available to you is bool buy(double qty);
* Check Price: double checkPrice() Will return the current market price of the currency.
*/
#include "cryptobroker.h"
double currentPrice = 0;
int main()
{
// UNCOMMENT THIS CODE TO SEE THE test function
/*
Account *account = new Account();
test(account);
*/
process();
return 0;
}
Problem We will convert the code for HW2 into a C+ class. In the class we have discussed a (very) incomplete stock trading code. (What are Requirements, Function Specifications and Design2) Requirements In this problem, we will develop a cryptocurrency trading application. Background Currently the three most popular cryptocurrencies are: Bitcoin: Symbol BTC Ethereum: Symbol ETH Rippl Symbol XRP The concept is the same as trading stocks. You want to buy when the price is low, and sell when the price is high to make a profit. For professors and students it only makes sense to trade a fraction of a coin at a time
Step by Step Solution
There are 3 Steps involved in it
Step: 1
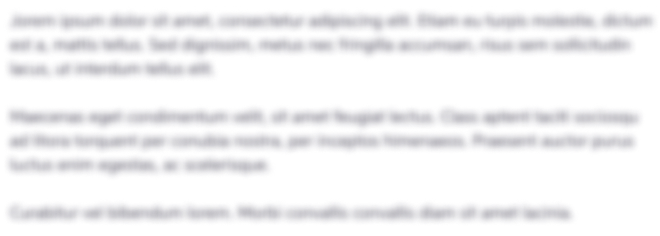
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started