Question
Four integers are read from input, where the first two integers are the miles and feet of distance1 and the second two integers are the
Four integers are read from input, where the first two integers are the miles and feet of distance1 and the second two integers are the miles and feet of distance2. Complete the function to overload the + operator.
Ex: If the input is 13 1415 2 642, then the output is:
13 miles, 1415 feet 2 miles, 642 feet Sum: 15 miles, 2057 feet
Note: The sum of two distances is:
the sum of the number of miles
the sum of the number of feet
#include
class Distance { public: Distance(int miles = 0, int feet = 0); void Print() const; Distance operator+(Distance rhs); private: int mi; int ft; };
Distance::Distance(int miles, int feet) { mi = miles; ft = feet; }
// No need to accommodate for overflow or negative values Distance Distance::operator+(Distance rhs) {
/* Your code goes here */
}
void Distance::Print() const { cout << mi << " miles, " << ft << " feet"; }
int main() { int miles1; int feet1; int miles2; int feet2; cin >> miles1; cin >> feet1; cin >> miles2; cin >> feet2; Distance distance1(miles1, feet1); Distance distance2(miles2, feet2); Distance sum = distance1 + distance2; distance1.Print(); cout << endl; distance2.Print(); cout << endl; cout << "Sum: "; sum.Print(); cout << endl; return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
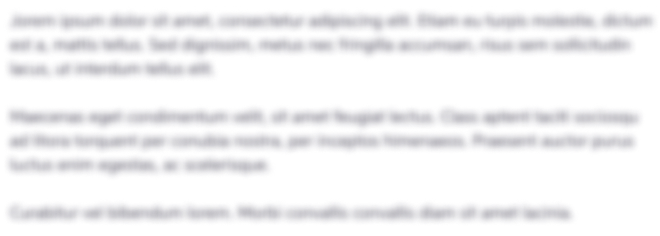
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started