Question
Fullness Experiment Part 1: in JAVA! a) design and implement a method height for BinarySearchTree that returns the height of the tree. b) Define the
Fullness Experiment Part 1: in JAVA!
a) design and implement a method height for BinarySearchTree that returns the height of the tree.
b) Define the fullness ratio of a binary tree to be the ratio between its minimum height and its height (given the number of nodes in the tree). For example, the tree in Figure 7.5a has a fullness ratio of 1.00 (its minimum height is 3 and its height is 3) and the tree in Figure 7.6c has a fullness ratio of 0.33 (its minimum height is 3 and its height is 9). Implement a method fRatio to be added to the BinarySearchTree class that returns the fullness ratio of the tree.
c) Create an application that generates 10 random trees, each with 1,000 nodes (random integers between 1 and 3,000). For each tree output its height, optimal height, and fullness ratio.
Binary Search and Binary Node below:
public class BinaryTreeNode
public BinaryTreeNode
public BinaryTreeNode
public T data; // the data in this node
public BinaryTreeNode() {
this(null, null, null);
}
public BinaryTreeNode(T theData) {
this(theData, null, null);
}
public BinaryTreeNode(T theData, BinaryTreeNode
data = theData;
left = leftChild;
right = rightChild;
}
public T getData() {
return data;
}
public BinaryTreeNode
return left;
}
public BinaryTreeNode
return right;
}
public void setLeft(BinaryTreeNode
left = newLeft;
}
public void setRight(BinaryTreeNode
right = newRight;
}
public void setData(T newData) {
data = newData;
}
public void preOrder() {
System.out.println(data);
if (left != null) {
left.preOrder();
}
if (right != null) {
right.preOrder();
}
}
public int height() {
int leftHeight = 0; // Height of the left subtree
int rightHeight = 0; // Height of the right subtree
int height = 0; // The height of this subtree
// If we have a left subtree, determine its height
if (left != null) {
leftHeight = left.height();
}
// If we have a right subtree, determine its height
if (right != null) {
rightHeight = right.height();
}
// The height of the tree rooted at this node is one more than the
// height of the 'taller' of its children.
if (leftHeight > rightHeight) {
height = 1 + leftHeight;
} else {
height = 1 + rightHeight;
}
// Return the answer
return height;
}
/**
* @param pathString
* @return the tree nodes in pre-order traversal
*/
public String toStringPreOrder(String pathString) {
String treeString = pathString + " : " + data + " ";
if (left != null) {
treeString += left.toStringPreOrder(pathString + "L");
}
if (right != null) {
treeString += right.toStringPreOrder(pathString + "R");
}
return treeString;
}
}
====================
import java.util.*;
public class BinarySearchTree
private BinaryTreeNode
public BinarySearchTree() {
root = null;
comparator = null;
}
public
return true;
}
public static void main(String[] args) {
Integer[] a = { 1, 5, 2, 7, 4 };
BinarySearchTree
for (Integer n : a)
bst.insert(n);
System.out.println("InOrder Traversal is");
bst.InorderTraversal();
System.out.println();
System.out.println("Preorder Traversal is");
bst.PreorderTraversal();
System.out.println();
System.out.println("Height is :" + bst.height());
System.out.println("Height is :" + bst.heightWithoutRecursion());
System.out.println("Left Count is: " + bst.LeafCount());
System.out.println("Is the element Present: " + bst.find(2));
System.out.println();
}
public int LeafCount() {
return LeafCount(root);
}
private int LeafCount(BinaryTreeNode
if (root == null)
return 0;
else if (root.left == null && root.right == null)
return 1;
else
return LeafCount(root.left) + LeafCount(root.right);
}
private Comparator
private int compare(T x, T y) {
if (comparator == null)
return x.compareTo(y);
else
return comparator.compare(x, y);
}
public void insert(T data) {
root = insert(root, data);
}
private BinaryTreeNode
if (root == null)
return new BinaryTreeNode
if (compare(toInsert, root.getData()) == 0)
return root;
if (compare(toInsert, root.getData()) <= 0)
root.left = insert(root.getLeft(), toInsert);
else
root.right = insert(root.right, toInsert);
return root;
}
public int getSizeUtil() {
return getSize(root);
}
public int getSize(BinaryTreeNode
if (root == null)
return 0;
else
return 1 + getSize(root.getRight()) + getSize(root.getLeft());
}
public void SearchUtil(T key) {
List
Search(root, key, list);
System.out.println(list.size());
}
public void Search(BinaryTreeNode
if (root == null)
return;
if (root.data.compareTo(key) == 0) {
list.add(root.data);
} else if (root.data.compareTo(key) <= 0)
Search(root.getLeft(), key, list);
else
Search(root.getRight(), key, list);
}
public void InorderTraversal() {
inOrderHelper(root);
}
private void inOrderHelper(BinaryTreeNode
if (root != null) {
inOrderHelper(root.getLeft());
System.out.print(root.getData() + " ");
inOrderHelper(root.getRight());
}
}
public int height() {
return height(root);
}
private int height(BinaryTreeNode
if (root == null)
return -1;
else
return 1 + Math.max(height(root.left), height(root.right));
}
public int heightWithoutRecursion() {
return heightWithoutRecursion(root);
}
int heightWithoutRecursion(BinaryTreeNode
{
if (node == null)
return 0;
Queue
q.add(node);
int height = -1;
while (1 == 1)
{
int nodeCount = q.size();
if (nodeCount == 0)
return height;
height++;
while (nodeCount > 0)
{
BinaryTreeNode newnode = q.peek();
q.remove();
if (newnode.left != null)
q.add(newnode.left);
if (newnode.right != null)
q.add(newnode.right);
nodeCount--;
}
}
}
public void PreorderTraversal() {
preOrderHelper(root);
}
private void preOrderHelper(BinaryTreeNode root) {
if (root != null) {
System.out.print(root.data + " ");
preOrderHelper(root.left);
preOrderHelper(root.right);
}
}
public boolean find(T key) {
return find(root, key);
}
private boolean find(BinaryTreeNode
if (root == null)
return false;
else if (compare(key, root.data) == 0)
return true;
else if (compare(key, root.data) < 0)
return find(root.left, key);
else
return find(root.right, key);
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
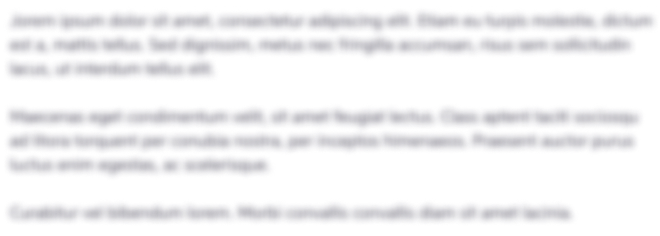
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started