Question
Function 1: divisible-by-5 In CLISP define your own function that takes a single integer as an argument and returns a Boolean that indicates whether the
Function 1: divisible-by-5
In CLISP define your own function that takes a single integer as an argument and returns a Boolean that indicates whether the number is divisible by 5. You do not have to perform error checking on the input.
Input: An integer
Output: A Boolean
Examples:
> (divisible-by-5 14)
#f
> (divisible-by-5 25)
#t
> (divisible-by-5 30)
#t
Function 2: function-7
In CLISP define a function that takes a function as an argument and passes the number 7 to that function. The function argument must be able to accept a single integer as its argument.
Input: A named function which takes a single number as an argument.
Output: The value returned by applying the name function to the number 7.
Examples:
> (function-7 #'sqrt)
2.645751311064591
> (function-7 #'(lambda (x) (+ x 7)))
14
> (function-7 #'1+)
8
Function 3: fib
In CLISP define a function using recursion to compute the Fibonacci number of n (where n is a positive integer)
Fib(n) = 1 for n = 0 or n = 1
Fib(n) = Fib(n-1) + Fib(n-2) for n > 1
Input: A positive integer used to calculate a Fibonacci number
Output: The value returned given the input integer
Examples:
> (fib 1)
1
> (fib 5)
8
> (fib 10)
89
Function 4: my-map
In CLISP define your own function that duplicates the functionality of mapcar from the standard library. You may not use the built-in mapcar function as a helper function. Your implementation must be recursive.
Input: The input to my-map is a function that takes a single argument and a homogeneous list of elements of the same data type compatible with the procedure. Note: the function argument can be named or anonymous (lambda).
Output: A new list of the original elements with the same procedure applied to each.
Examples:
> (my-map #'sqrt '(9 25 81 49))
'(3 5 9 7)
> (my-map #'1+ '(6 4 8 3))
'(7 5 9 4)
> (my-map (lambda (n) (* n n)) '(5 7))
'(25 49)
> (my-map #'evenp '(2 5 7 12))
'(#t #f #f #t)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
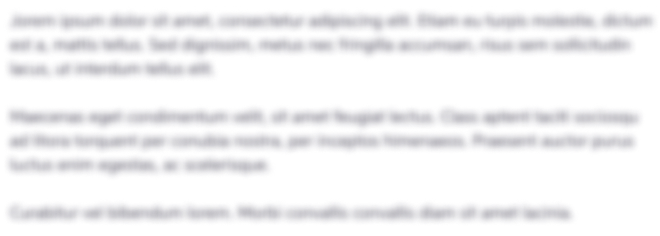
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started