Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Functional Programming in Racket and the map / reduce paradigm. 1 . Implement the function ( lookup var env ) where var is a variable,
Functional Programming in Racket and the mapreduce paradigm.
Implement the function lookup var env where var is a variable, ie a symbol, and env is an
environment. An environment is a list of bindings and a binding a list of length two where the
first element is a variable and the second is the value bound to the variable. The function
lookup returns the value bound to a given variable in the specified environment. If the variable
is not found in the environment, then use the Racket function error to throw an error. EG
lookup y x y returns
The following function is given a list and returns a new list whose elements are those in the
input but in reverse order. EGrev
define rev l
if null l
null
append rev rest lcons first l null
The function rev uses the function append which appends the list y after the list x
define append x y
if null x
y
cons first xappend rest x y
The function rev takes Theta n
time where n is the length of the list why Tail recursion can be
used to obtain a linear time algorithm. Implement reverse using tail recursion. See the example
from the lecture on functional programming. Hint: you will need a helper function that takes an
additional argument and is more general than reverse.
Implement the function map f L which takes two arguments: f a function of a single variable
and L a list of elements in the domain of f If L x x xn the output of the function is the
list f xf xf xn EG assuming the function sqr defined by define sqr x x x the
result of map sqr is First try this example using the Racket function map
and then see that you get the same result using your function. Note that Rackets map function
is more general in that it allows functions of more than one variable when additional lists are
provided.
Implement the function reduce f init L which takes three arguments: f a function of two
variables and L a list of elements in the domain of f and init the value to be returned when
L Reduce is the same as the Racket function foldr. EGreduce is
reduce First try the Racket function foldr, iefoldr
and then see that your implementation obtains the same result. Try the other examples
from the functional programming in Racket lecture.
Use map and reduce to solve the following problem: count the number of negative numbers in
a list. Try to make your code as general as possible. Can you use your code to count the
number of elements of a list that satisfy a given predicate?
Given a set of times dominoes, write a Racket function to generate all of the different ways to to
tile a times n rectangle placing a single domino vertically times or stacking two dominoes
horizontally? For example, for n there are ways.
Think recursively: What are the base cases and how many do you need? How do you solve the
times n using solutions to smaller problems?
Represent tilings with V for one vertical tile and HH for two stacked
horizontal tiles. In the above times example, the three tilings are represented by
the strings VVVVHH and HHV Note that all three solutions have length
Your function, GenDominoes n should return a list of strings, one for each
possible tiling. In the above example, GenDominoes should return the list
VVVVHHHHV
Your function should recursively generate all solutions of size n and
concatenate a V in front of each of them, and recursively generate all
solutions of size n and concatenate a HH in front of them. Use map to
perform the concatenation and use append to combine the solutions. What is
the base case of your recursion?
You should use Rackets built in string concatenation function: stringappend.
See the Racket documentation for details on stringappend. Make sure you try
it out before you write a function that uses it
Step by Step Solution
There are 3 Steps involved in it
Step: 1
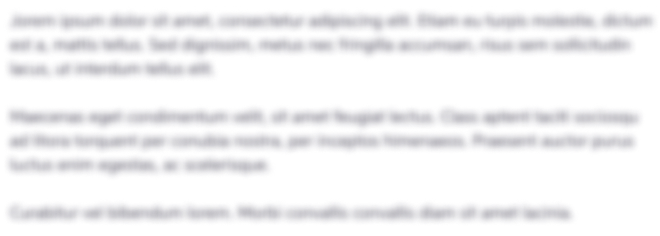
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started