Question
game of yahtzee using previous functions below: def drawCenterDot(t, x, y, width, dot_width): t.penup() t.goto(x + width / 2, y - width / 2) t.pendown()
game of yahtzee using previous functions below:
def drawCenterDot(t, x, y, width, dot_width): t.penup() t.goto(x + width / 2, y - width / 2) t.pendown() t.dot(dot_width)
def drawCornerDots(t, x, y, width, dot_width): t.penup()
t.goto(x + dot_width, y - dot_width) t.pendown() t.dot(dot_width)
t.penup() t.goto(x + width - dot_width, y - width + dot_width) t.pendown() t.dot(dot_width)
def drawOtherCornerDots(t, x, y, width, dot_width): t.penup()
t.goto(x + width - dot_width, y - dot_width) t.pendown() t.dot(dot_width)
t.penup() t.goto(x + dot_width, y - width + dot_width) t.pendown() t.dot(dot_width)
def drawSideDots(t, x, y, width, dot_width): t.penup()
t.goto(x + dot_width, y - width / 2) t.pendown() t.dot(dot_width)
t.penup() t.goto(x + width - dot_width, y - width / 2) t.pendown() t.dot(dot_width)
def drawDots(t, value, x, y, width):
dot_width = width / 5.0
if value == 1: drawCenterDot(t, x, y, width, dot_width)
elif value == 2: drawCornerDots(t, x, y, width, dot_width)
elif value == 3: drawCenterDot(t, x, y, width, dot_width) drawOtherCornerDots(t, x, y, width, dot_width)
elif value == 4: drawCornerDots(t, x, y, width, dot_width) drawOtherCornerDots(t, x, y, width, dot_width)
elif value == 5: drawCenterDot(t, x, y, width, dot_width) drawCornerDots(t, x, y, width, dot_width) drawOtherCornerDots(t, x, y, width, dot_width)
elif value == 6: drawCornerDots(t, x, y, width, dot_width) drawOtherCornerDots(t, x, y, width, dot_width) drawSideDots(t, x, y, width, dot_width)
def drawDie(t, value, x, y, width, color='white'): t.penup()
t.goto(x, y) t.setheading(0) t.fillcolor(color)
# drawing blank square t.pendown() t.begin_fill() for _ in range(4): t.fd(width) t.rt(90)
t.end_fill() t.penup()
drawDots(t, value, x, y, width):
t.penup()
t.goto(x, y) t.setheading(0) t.fillcolor(color)
t.pendown() t.begin_fill() for _ in range(4): t.forward(width) t.right(90) t.end_fill() t.penup() drawDots(t, value, x, y, width)
I will post the rest, but nobody is answering my other posts so i wanted to start easy.
Global Data We will need some global variables because of the nature of detecting a mouse click on the screen. Create global variables for: 1. A list of lists for five dice. Each sublist should contain: a. die value b. x coordinate of the upper left-hand corner of the die c. y coordinate of the upper left-hand corner of the die d. width e. color 2. A list of lists for two buttons. Each sublist should contain: a. x coordinate of the upper left-hand corner of the button b. y coordinate of the upper left-hand corner of the button C. width d. height e. border color (or pen color) f. background color (or fill color) g. Text 3. A window object initialized from the turtle.Screen() 4. A turtle object (please don't call it "turtle") main() Create a main() function that does the following: 1. Declare the list of dice, list of buttons, and turtle object as global 2. Set the background color, height, and width of the window to values of your choice. 3. Hide the turtle and set its speed to zero (the fastest). 4. Register the mouseClick() function (see below) as a listener for the window. 5. Draw the dice using the drawDie() function (see below). 6. Draw the buttons using the drawRectangle() function (see below). 7. Call the turtle.mainloop function. mouseClick() Create a mouseClick() function that: 1. Takes as a parameters: a. x coordinate of the mouse click b. y coordinate of the mouse click 2. And then does: a. Declare the list of dice and the list of buttons as global b. Iterate through the list of buttons to see if the mouse click was within a button (using the isWithin function (see below); you will need to get the coordinates of the button from the sublist). If it was, then call the buttonClick() function (see below) with the index of the button within the list of buttons. c. Iterate through the list of dice to see if the mouse click was within a dice (using the isWithino function; you will need to get the coordinates of the die from the sublist; note that the height of the dice is the same as the width). If it was, then call the dieClick() function (see below) with the index of the die within the list of dice isWithin() Create a function isWithin (from Lab 19) that: 1. Takes as a parameters: a. x coordinate of the mouse click b. y coordinate of the mouse click c. a coordinate of the upper left-hand corner of a rectangle d. b coordinate of the upper left-hand corner of a rectangle e. width of a rectangle f. height of a rectangle 2. And then does: a. returns True if the (x, y) coordinates of the mouse click are within the rectangle b. returns False otherwise buttonClick() Create a function buttonClick() that: 1. Takes as a parameters: a. The index of the button within the list of buttons 2. And then does: a. Declare the list of dice, the list of buttons, and the turtle object as global b. If the 1st button was clicked, then set the colors of all dice to "white" c. For each dice in the list of dice: i. If its color is "white" then assign a new random number between 1 and 6 to it and redraw it. dieClick() Create a function dieClick() that: 1. Takes as a parameters: a. The index of the die within the list of dice 2. And then does: a. Declare the list of dice and the turtle object as global b. If the die's color is white, change it to a different color (your choice); c. Otherwise, change its color back to white d. Redraw the die. drawRectangle() 1. Create a function drawRectangle() that: 2. Take as parameters: i. A Turtle Object ii. x coordinate iii. y coordinate iv. width v. height vi. line color vii. fill color viii. Text (default is None) 3. The drawRectangle() should: i. Raise the pen ii. Move the turtle to (x,y) iii. Set the pen color iv. Set the fill color v. Lower the pen vi. Begin filling vii. Draw a rectangle with the width and height given. Be sure to turn the turtle to the right so that the x and y coordinates are the upper left-hand corner viii. End filling ix. Raise the pen x. If the text is not None, then write the text on the rectangle Global Data We will need some global variables because of the nature of detecting a mouse click on the screen. Create global variables for: 1. A list of lists for five dice. Each sublist should contain: a. die value b. x coordinate of the upper left-hand corner of the die c. y coordinate of the upper left-hand corner of the die d. width e. color 2. A list of lists for two buttons. Each sublist should contain: a. x coordinate of the upper left-hand corner of the button b. y coordinate of the upper left-hand corner of the button C. width d. height e. border color (or pen color) f. background color (or fill color) g. Text 3. A window object initialized from the turtle.Screen() 4. A turtle object (please don't call it "turtle") main() Create a main() function that does the following: 1. Declare the list of dice, list of buttons, and turtle object as global 2. Set the background color, height, and width of the window to values of your choice. 3. Hide the turtle and set its speed to zero (the fastest). 4. Register the mouseClick() function (see below) as a listener for the window. 5. Draw the dice using the drawDie() function (see below). 6. Draw the buttons using the drawRectangle() function (see below). 7. Call the turtle.mainloop function. mouseClick() Create a mouseClick() function that: 1. Takes as a parameters: a. x coordinate of the mouse click b. y coordinate of the mouse click 2. And then does: a. Declare the list of dice and the list of buttons as global b. Iterate through the list of buttons to see if the mouse click was within a button (using the isWithin function (see below); you will need to get the coordinates of the button from the sublist). If it was, then call the buttonClick() function (see below) with the index of the button within the list of buttons. c. Iterate through the list of dice to see if the mouse click was within a dice (using the isWithino function; you will need to get the coordinates of the die from the sublist; note that the height of the dice is the same as the width). If it was, then call the dieClick() function (see below) with the index of the die within the list of dice isWithin() Create a function isWithin (from Lab 19) that: 1. Takes as a parameters: a. x coordinate of the mouse click b. y coordinate of the mouse click c. a coordinate of the upper left-hand corner of a rectangle d. b coordinate of the upper left-hand corner of a rectangle e. width of a rectangle f. height of a rectangle 2. And then does: a. returns True if the (x, y) coordinates of the mouse click are within the rectangle b. returns False otherwise buttonClick() Create a function buttonClick() that: 1. Takes as a parameters: a. The index of the button within the list of buttons 2. And then does: a. Declare the list of dice, the list of buttons, and the turtle object as global b. If the 1st button was clicked, then set the colors of all dice to "white" c. For each dice in the list of dice: i. If its color is "white" then assign a new random number between 1 and 6 to it and redraw it. dieClick() Create a function dieClick() that: 1. Takes as a parameters: a. The index of the die within the list of dice 2. And then does: a. Declare the list of dice and the turtle object as global b. If the die's color is white, change it to a different color (your choice); c. Otherwise, change its color back to white d. Redraw the die. drawRectangle() 1. Create a function drawRectangle() that: 2. Take as parameters: i. A Turtle Object ii. x coordinate iii. y coordinate iv. width v. height vi. line color vii. fill color viii. Text (default is None) 3. The drawRectangle() should: i. Raise the pen ii. Move the turtle to (x,y) iii. Set the pen color iv. Set the fill color v. Lower the pen vi. Begin filling vii. Draw a rectangle with the width and height given. Be sure to turn the turtle to the right so that the x and y coordinates are the upper left-hand corner viii. End filling ix. Raise the pen x. If the text is not None, then write the text on the rectangleStep by Step Solution
There are 3 Steps involved in it
Step: 1
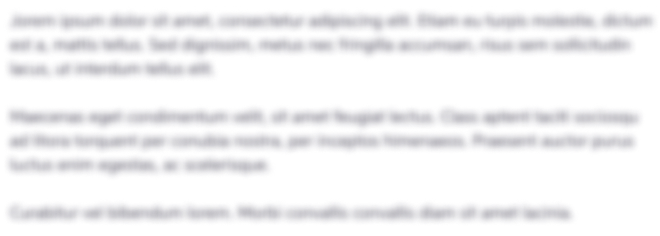
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started