Question
Geometric Shapes Lab In this lab, you will receive a skeleton of the domain class, GeometricShape, and the driver class, GeometryDriver. In the GeometricShape class,
Geometric Shapes Lab In this lab, you will receive a skeleton of the domain class, GeometricShape, and the driver class, GeometryDriver. In the GeometricShape class, you will be given the attributes, the overloaded constructors, the getters, and the setters. You only have to code 2 methods: 1.) .toString() depending on the shape that has been created, output the appropriate attributes for that geometric shape 2.) calculatePerimeter() - calculates the perimeter of the Geometric object depending on what the object is. Here are the formulas for each of the 3 geometric shapes that can be created: Perimeter of a Triangle = Side1 + Side2+ Side3 Perimeter of a Rectangle = (2 * length) + (2 * width) Perimeter of a Circle = 2 * Math.PI * radius Use an if-statement and check the 3 boolean variables (isTriangle, isRectangle, isCircle) to determine what geometric shape was created, and then calculate the perimeter using the correct formula, for the specific geometric shape. In the GeometryDriver class, you will be given an empty main method, and you will be asked to code the following within it: 1.) Define local variables to hold the following data: userChoice (from a menu of options: 1 = Circle Perimeter; 2 = Rectangle Perim.; 3 = Triangle Perim.) radius side1, side2, and side3 length, width perimeter keyboard (a Scanner object) 2.) Display the following menu: System.out.println("Welcome to the Geometry Calculator! " + "In this program we will use a menu to decide what kind of shape we will create. " + " 1.Create and Calculate Perimeter of a Circle" + " 2. Create and Calculate Perimeter of a Rectangle" + " 3. Create and Calculate Perimeter of a Triangle"); 3.) Get the users input; a default geometric shape is created before the switch to allow for a single print afterwards. 4.) Use a switch statement to evaluate what the user entered (1, 2 or 3) a. Obtain the remaining data from the user, and re-create the appropriate geometric object b. The number of arguments that are passed to the constructor determines which constructor will be executed (1 argument = circles constructor, 2 arguments= rectangles constructor, 3 arguments = triangles constructor). 5.) Call the calculatePerimeter() method 6.) Print the contents of the geometric object and print its perimeter ******************************* /**
* * @ */
public class GeometricShape
{
private double side1, side2, side3;
//These are the variables necessary to calculat the perimeter of a triangle private double radius;
//Radius is needed to calculate the perimeter of a circle private double length, width;
//Length and Width are needed to calculate the perimeter of a rectangle private boolean triangle = false;
private boolean rectangle = false; private boolean circle = false;
//Constructor for a triangle public GeometricShape(double aSide1, double aSide2, double aSide3)
{ side1 = aSide1; side2 = aSide2; side3 = aSide3; triangle = true; }
//Constructor for a rectangle public GeometricShape(double aLength, double aWidth)
{ length = aLength; width = aWidth; rectangle = true; }
//Constructor for a circle public GeometricShape(double aRadius)
{ radius = aRadius; circle = true; }
public double getRadius()
{ return radius; }
public double getSide1()
{ return side1; }
public double getSide2()
{ return side2; }
public double getSide3()
{ return side3; } public double getLength()
{ return length; } public double getWidth()
{ return width; } public void setRadius(double aRadius)
{ radius = aRadius; } public void setSide1(double aSide1)
{ side1 = aSide1; } public void setSide2(double aSide2)
{ side2 = aSide2; } public void setSide3(double aSide3)
{ side3 = aSide3; } public void setLength(double aLength)
{ length = aLength; } public void setWidth(double aWidth)
{ width = aWidth; } public double getPerimeter()
{
//Calculate the perimeter of the Geometric object depending on what the object is.
//Remember the following formulas:
//Perimeter of a Triangle = Side1 + Side2+ Side3
//Perimeter of a Rectangle = (2 * length) + (2 * width)
//Perimeter of a Circle = 2 * Math.PI * radius if (triangle == true) { double perimeter = side1 + side2 + side3; return perimeter; } else if (rectangle == true) { double perimeter = (2 * length) + (2 * width); return perimeter; } else if (circle == true) { double perimeter = 2 * Math.PI * radius; return perimeter; } return 0; }
public String toString() { if (triangle == true) { return "It's a triangle, with perimeter of " + side1 + side2 + side3; } else if (rectangle == true) { return " It's a rectangle and preimeter of " + (2 * length) + (2 * width); } else if (circle == true) { return "It's a circle and perimeter of " + 2 * Math.PI * radius; }
//complete formula //Continue with the rest of the else if logic here. //change return statement to match the perimeter of the shape //return the content of the Geometric object, depending on what the Geometric object is...
******************************
import java.util.Scanner; /* * To change this license header, choose License Headers in Project Properties. * To change this template file, choose Tools | Templates * and open the template in the editor. */ /** * * @author Matt */ public class GeometryDriver { public static void main(String[] args) { //In main, define local variables to hold the following data: // 1.) userChoice (from a menu of options, 1 = Circle Perimeter; 2 = Rectangle Perimeter; 3 = Triangle Perimeter // 2.) radius // 3.) side1, side2, and side3 // 4.) length, width // 5.) perimeter // 6.) keyboard (a Scanner object) Scanner keyboard = new Scanner(System.in); int option; //Display the following menu displayMenu(); System.out.println("Welcome to the Geometry Calculator! " + "In this program we will use a menu to decide what kind of shape we will create. " + " 1.Create and Calculate Perimeter of a Circle" + " 2. Create and Calculate Perimeter of a Rectangle" + " 3. Create and Calculate Perimeter of a Triangle"); //Use a switch statement to determine the option that the user selected. //Depending on the option that the user selected, ask the user for the appropriate information needed to create the //a specific geometric object. Then, call the getPerimeter() method to calculate the perimeter for the geometric object created. //Print the object created and its perimeter. option = keyboard.nextInt(); //The code below is a default shape, so only 1 sout statement //is needed at the end of the switch statement. GeometricShape aShape = new GeometricShape(-1, -1); switch (option) { case 1: // case 1: System.out.print("Enter radius: "); int radius = scanner.nextInt(); double aShape = aShape.getRadius(); System.out.println("Perimeter = "); break; case 2: System.out.print("Enter side: "); int side = scanner.nextInt(); double areaOfSquare = area.areaOfSquare(side); System.out.println("Area = " + areaOfSquare); break; case 3: System.out.print("Enter length: "); int length = scanner.nextInt(); System.out.print("Enter breadth: "); int breadth = scanner.nextInt(); double areaOfRectangle = area.area(length, breadth); System.out.println("Area = " + areaOfRectangle); break; //re-create a GeometricShape with the radius //put logic here to call the perimeter method case 2: //ask user for the length and width //re-create a GeometricShape with the length and width //put logic here to call the perimeter method break; case 3: //ask user for side1, side2, and side3 //re-create a GeometricShape with the the 3 sides //put logic here to call the perimeter method break; default: //Give message to user that option is not valid. } //Print the geometric shape and its perimeter } }
Need help to complete this.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
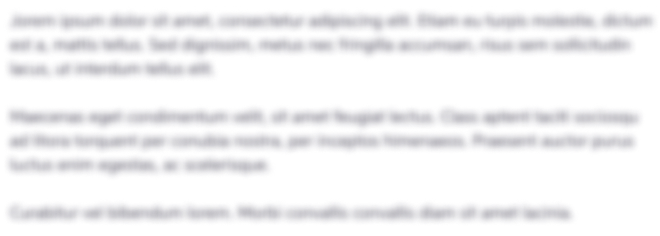
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started