Question
Get Thumbs up from me. Please help. Can anyone try this? this my 5th attempt to get this solved so far no one has submitted
Get Thumbs up from me. Please help. Can anyone try this? this my 5th attempt to get this solved so far no one has submitted a solution for this. :( I started feeling that Chegg can not help anymore because i got my question refunded 4 times still no answer. :(
Purpose Calculate payroll data using classes write complete program under one class
Upload Screenshot of output. and write a complete program under one class.
Java Topics Classes, arrays of classes, processing partial arrays, loops.
Files Used These files are in Flip_Files.zip and should be in the same folder as your program:
Employee.java Employee data tracked
EmployeeParameters.java Options used in the program
EmployeeParameters.txt The parameters for EmployeeParameters
Specification Except for the Toolkit and constants noted below, do NOT use static (global) variables. Also, all output to the output file should be echoed on the console.
Overview
Read this programs parameters of the maximum number of employees, savings rate, IRA rate, federal tax rate and state tax rate. A class, EmployeeParameters, and a method in that class are provided to read the parameter file named EmployeeParameters.txt.
Access the Employee class to see what data values will be stored.
Create an array of the Employee class of maximum number of employees
Read the input file into the Employee array. The input data is, in this order, the hours worked, the pay rate and the employee name
Calculate the gross pay for each employee based on a table.
Calculate the remaining values in the Employee class. Note that you can add variables to the Employee class if it will help write the program more efficiently and/or more easily.
Now that all the data has been calculated, print a heading and the detail lines per the spec below.
Calculate the totals of all columns, except the pay rate, which you will average.
Sort the employees alphabetically (using a provided method) and print the details the same way you did in step G above
Print the totals as in step H
Sort the employees by increasing gross pay ((using a provided method) and print the details the same way you did in step G above
Print the totals as in step H
Details follow for the main program
1. Read the Employee parameters. Access the EmployeeParameters class with, say
EmployeeParameters params = new EmployeeParameters();
The parameter class is as follows:
public class EmployeeParameters {
public int maxEmployees; // Maximum number of employees
public double savingsRate; // Savings rate as a percent
public double iraRate // IRA investment rate as a percent
public federalWithholdingRate; // Federal withholding tax rate as a percent
public stateWithholding; // State withholding tax rate as a percent
// Constructor and other methods follow. View the available methods in the class.
} // End class
Use the method in this class, getEmployeeParameters, to read the program parameters. Note that the variables in this class are declared public, not private, so you can access them from the main program. For example, after calling getEmployeeParameters, you can assign to a local variable the maximum number of employees with
maxEmployees = params.maxEmployees;
2. Use an array of a class to hold employee data. The array might be partially filled. The separate class is named Employee (in file Employee.java) and has this definition:
public class Employee {
String name; // Name of the employee
public double hoursWorked; // Hours worked in the payroll period
public double payRate; // Hourly pay rate
public double grossPay; // Gross pay based on the number of hours worked
public double adjustedGrossPay; // Gross pay less amount that goes into the IRA
public double netPay; // Gross pay less taxes
public double savingsAmount; // Amount of gross pay that goes to savings
public double iraAmount; // Amount of gross pay that goes into the IRA
public double taxAmount; // Amount of tax based on gross pay and tax rates
} // End class
You can add fields to the Employee class if it helps writing your program. In the main method, you will define an array of the above class as:
Employee[ ] empl = new Employee[maxEmployees];
where maxEmployees is a number read from the Employee Parameter file as described above in step 1. Note that this allocates space for the array but does not create instances for each employee.
Using the index i as an example, an instance of Employee can be created with a statement like:
empl[i] = new Employee();
Using the index i as an example, an instance variable can be accessed in a method with a statement like:
empl[i].netPay = empl[i].grossPay empl[i].taxAmount;
The main program should consist mostly of method calls. Use appropriate parameter passing between methods and no non-local (static) variables.
3. Data is read from a text file named YourName_S_10_Input.txt and writes to a text file named YourName_S_10_Output.txt. The input file has the employee data. Each line of employee data contains the number of hours worked, the pay rate (both doubles), followed by the name. One or more spaces separate the fields.
4. Gross pay is calculated in a separate method as follows:
hours 40.0 Paid at the pay rate.
40.0 < hours 50.0 hours > 40.0 and less 50.0 are paid at one and a half times the rate. The first 40 hours are paid at the pay rate.
50.0 < hours hours > 50.0 are paid at twice the pay rate. The first 40 hours are paid at the pay rate. Hours 40 to 50 are paid at time and a half.
Examples: with a pay rate is $10.00 an hour, gross pay is calculated as follows:
# Hours Gross Pay
30 30 x $10 = $300
40 40 x $10 = $400
46 40 x $10 + ( (46-40) x $10 x 1.5 ) = $400 + (6 x $15) = $490
50 40 x $10 + ( (50-40) x $10 x 1.5 ) = $400 + (10 x $15) = $400 + $150 = $550
58 40 x $10 + ( (50-40) x $10 x 1.5) + ( (58-50) x $10 x 2) = $400 + $150 + $160
= $710
Note how the pay rate ($10 in the example above) can be factored out, which you should do in the program. For example, the reimbursement for 46 hours is
$10 x (40 + (46-40) x 1.5) = $10 x (40 + (6 x 1.5)) = $10 x (40 + 9) = $490
Do you see why?
5. Use a method to calculate the variables for each instance of an entry in the Employee array, empl:
a. IRA amount = gross pay * IRA investment rate / 100
b. Adjusted gross pay = gross pay - IRA amount
c. Taxes = adjusted gross pay * (the sum of the tax rates as decimals)
d. Net pay = adjusted gross pay taxes
e. Savings amount = net pay * savings rate / 100
6. A method to create a report -- this method will be called after all input and all calculations are done and in turn calls three methods as follows:
a. A method to print heading information as shown in the sample output below. The information includes the report title, column headings for the name, gross pay, net pay, wealth accumulation, taxes for that employee, hours worked, and pay rate, in that order. Also shown here are the first few lines of the data in input file order and the respective totals and average that you can use to ensure you are getting the correct results. Heres how the report should look (partial list):
In Input Order
Mobile Apps Galore, Inc. - Payroll Report
Name Gross Pay Net Pay Wealth Taxes Hours Pay Rate
------------------ --------- ------- ------ --------- ----- --------
Hancock John 487.21 347.38 73.71 100.85 41.00 11.74
Light Karen L 583.00 415.68 88.21 120.68 50.00 10.60
Fagan Bert Todd 637.20 454.32 96.41 131.90 52.00 10.80
Antrim Forrest N 966.96 689.44 146.30 200.16 62.00 12.24
Camden Warren 566.02 403.57 85.64 117.17 38.40 14.74
Mulicka Al B 559.80 399.14 84.70 115.88 44.33 12.04
etc.
Totals: 14,729.71 10,502.28 2,228.60 3,049.05 1,103.14
Average: 12.36
This heading method has a parameter that indicates the print order of the data.
b. A method to print the above detail lines for all employees, matching the headings indicated in part a. Single space the detail lines. The wealth column has the sum of the savings amount and the IRA amount.
c. A method that calculates the totals for gross pay, net pay, wealth, taxes and hours, and the average(not sum) pay rate. Print a summary line with those totals and the average. (The summary line should print the totals at the bottom of the appropriate columns.) Also, print a line with a message that contains the number of employees processed which could be less than 30. You can store the totals in an array or in a separate class.
7. After the report has been printed, sort the employees alphabetically and print out the report again with the same print methods: heading, details and summary. The sort used is located in Toolkit and is called selectionSortArrayOfClass which is discussed at the end of this document and will be reviewed in class. Use the print report method described above to print the data in this order.
8. Next, sort the employees in ascending order by gross pay and print out the header, details and summary again. Use the same selectionSortArrayOfClass for the sort. Use the print report method described above to print the data in this order.
9. The Data. The input data file format is the number of hours (double), the pay rate (double) and a name (which may or may not have an initial). One or more spaces separate the fields. The file has been uploaded and is named CS1050_Assignment_10_Input.txt. Remember to trim the name once youve extracted it from the input file. The String method name is trim(). Use this data:
41.00 11.74 Hancock John
50.00 10.60 Light Karen L
52.00 10.80 Fagan Bert Todd
62.00 12.24 Antrim Forrest N
38.40 14.74 Camden Warren
44.33 12.04 Mulicka Al B
41.75 13.40 Lee Phoebe
24.00 11.40 Bright Harry
41.00 10.40 Garris Ted
43.00 12.00 Benson Martyne
31.90 12.40 Lloyd Jeanine D
44.00 13.50 Leslie Bennie A
48.40 14.40 Brandt Leslie
42.00 12.90 Schulman David
50.10 10.84 Worthington Dan
70.40 12.66 Hall Gus W
40.10 12.74 Prigeon Dale R
43.00 12.44 Fitzgibbons Rusty
50.00 12.24 Feistner Merle S
23.00 12.34 Hallquist Dottie
43.33 10.90 Bolton Seth
43.00 12.20 Taylor Gregg
42.00 12.94 Raskin Rose
50.10 12.44 Kenwood Pat
44.33 14.64 Slaughter Lew
___________________________________________________________________________
Using the Sort Routine in the Toolkit
Heres how to use the sort routine thats in the Toolkit. Assume the following declarations:
static Toolkit tools = new Toolkit(); // Access the Toolkit
// Assume
// sortResult is set when the sort routine is called. It is:
// 0 if the sort ended correctly
// 1 if the employee array is empty
// 2 if the sort type is not one of Name or Gross Pay
int sortResult = 0;
Employee[ ] empl = new Employee[maxEmployees];
nNames is an integer with the number of data lines read which could be less than maxEmployees.
// Some of the steps call for sorting the empl array. To sort by name:
sortResult = tools.selectionSortArrayOfClass(empl, nNames, Name);
// To sort by gross pay:
sortResult = tools.selectionSortArrayOfClass(empl, nNames, Gross Pay);
Employee Parameter
30 10.0 8.0 18.0 4.5
Employee Input
41.00 11.74 Hancock John 50.00 10.60 Light Karen L 52.00 10.80 Fagan Bert Todd 62.00 12.24 Antrim Forrest N 38.40 14.74 Camden Warren 44.33 12.04 Mulicka Al B 41.75 13.40 Lee Phoebe 24.00 11.40 Bright Harry 41.00 10.40 Garris Ted 43.00 12.00 Benson Martyne 31.90 12.40 Lloyd Jeanine D 44.00 13.50 Leslie Bennie A 48.40 14.40 Brandt Leslie 42.00 12.90 Schulman David 50.10 10.84 Worthington Dan 70.40 12.66 Hall Gus W 40.10 12.74 Prigeon Dale R 43.00 12.44 Fitzgibbons Rusty 50.00 12.24 Feistner Merle S 23.00 12.34 Hallquist Dottie 43.33 10.90 Bolton Seth 43.00 12.20 Taylor Gregg 42.00 12.94 Raskin Rose 50.10 12.44 Kenwood Pat 44.33 14.64 Slaughter Lew
Employee output
Input File Order Mobile Apps Galore, Inc. - Payroll Report
Name Gross Pay Net Pay Wealth Taxes Hours Pay Rate ------------------ --------- ------- ------ --------- ------- -------- Hancock John 487.21 347.38 73.71 100.85 41.00 11.74 Light Karen L 583.00 415.68 88.21 120.68 50.00 10.60 Fagan Bert Todd 637.20 454.32 96.41 131.90 52.00 10.80 Antrim Forrest N 966.96 689.44 146.30 200.16 62.00 12.24 Camden Warren 566.02 403.57 85.64 117.17 38.40 14.74 Mulicka Al B 559.80 399.14 84.70 115.88 44.33 12.04 Lee Phoebe 571.18 407.25 86.42 118.23 41.75 13.40 Bright Harry 273.60 195.08 41.40 56.64 24.00 11.40 Garris Ted 431.60 307.73 65.30 89.34 41.00 10.40 Benson Martyne 534.00 380.74 80.79 110.54 43.00 12.00 Lloyd Jeanine D 395.56 282.03 59.85 81.88 31.90 12.40 Leslie Bennie A 621.00 442.77 93.96 128.55 44.00 13.50 Brandt Leslie 757.44 540.05 114.60 156.79 48.40 14.40 Schulman David 554.70 395.50 83.93 114.82 42.00 12.90 Worthington Dan 598.37 426.64 90.53 123.86 50.10 10.84 Hall Gus W 1,212.83 864.75 183.50 251.06 70.40 12.66 Prigeon Dale R 511.51 364.71 77.39 105.88 40.10 12.74 Fitzgibbons Rusty 553.58 394.70 83.76 114.59 43.00 12.44 Feistner Merle S 673.20 479.99 101.86 139.35 50.00 12.24 Hallquist Dottie 283.82 202.36 42.94 58.75 23.00 12.34 Bolton Seth 490.45 349.69 74.20 101.52 43.33 10.90 Taylor Gregg 542.90 387.09 82.14 112.38 43.00 12.20 Raskin Rose 556.42 396.73 84.19 115.18 42.00 12.94 Kenwood Pat 686.69 489.61 103.90 142.14 50.10 12.44 Slaughter Lew 680.69 485.33 102.99 140.90 44.33 14.64
Totals: 14,729.71 10,502.28 2,228.60 3,049.05 1,103.14 Average: 12.36
Alphabetical Order Mobile Apps Galore, Inc. - Payroll Report
Name Gross Pay Net Pay Wealth Taxes Hours Pay Rate ------------------ --------- ------- ------ --------- ------- -------- Antrim Forrest N 966.96 689.44 146.30 200.16 62.00 12.24 Benson Martyne 534.00 380.74 80.79 110.54 43.00 12.00 Bolton Seth 490.45 349.69 74.20 101.52 43.33 10.90 Brandt Leslie 757.44 540.05 114.60 156.79 48.40 14.40 Bright Harry 273.60 195.08 41.40 56.64 24.00 11.40 Camden Warren 566.02 403.57 85.64 117.17 38.40 14.74 Fagan Bert Todd 637.20 454.32 96.41 131.90 52.00 10.80 Feistner Merle S 673.20 479.99 101.86 139.35 50.00 12.24 Fitzgibbons Rusty 553.58 394.70 83.76 114.59 43.00 12.44 Garris Ted 431.60 307.73 65.30 89.34 41.00 10.40 Hall Gus W 1,212.83 864.75 183.50 251.06 70.40 12.66 Hallquist Dottie 283.82 202.36 42.94 58.75 23.00 12.34 Hancock John 487.21 347.38 73.71 100.85 41.00 11.74 Kenwood Pat 686.69 489.61 103.90 142.14 50.10 12.44 Lee Phoebe 571.18 407.25 86.42 118.23 41.75 13.40 Leslie Bennie A 621.00 442.77 93.96 128.55 44.00 13.50 Light Karen L 583.00 415.68 88.21 120.68 50.00 10.60 Lloyd Jeanine D 395.56 282.03 59.85 81.88 31.90 12.40 Mulicka Al B 559.80 399.14 84.70 115.88 44.33 12.04 Prigeon Dale R 511.51 364.71 77.39 105.88 40.10 12.74 Raskin Rose 556.42 396.73 84.19 115.18 42.00 12.94 Schulman David 554.70 395.50 83.93 114.82 42.00 12.90 Slaughter Lew 680.69 485.33 102.99 140.90 44.33 14.64 Taylor Gregg 542.90 387.09 82.14 112.38 43.00 12.20 Worthington Dan 598.37 426.64 90.53 123.86 50.10 10.84
Totals: 14,729.71 10,502.28 2,228.60 3,049.05 1,103.14 Average: 12.36
Ascending Gross Pay Order Mobile Apps Galore, Inc. - Payroll Report
Name Gross Pay Net Pay Wealth Taxes Hours Pay Rate ------------------ --------- ------- ------ --------- ------- -------- Bright Harry 273.60 195.08 41.40 56.64 24.00 11.40 Hallquist Dottie 283.82 202.36 42.94 58.75 23.00 12.34 Lloyd Jeanine D 395.56 282.03 59.85 81.88 31.90 12.40 Garris Ted 431.60 307.73 65.30 89.34 41.00 10.40 Hancock John 487.21 347.38 73.71 100.85 41.00 11.74 Bolton Seth 490.45 349.69 74.20 101.52 43.33 10.90 Prigeon Dale R 511.51 364.71 77.39 105.88 40.10 12.74 Benson Martyne 534.00 380.74 80.79 110.54 43.00 12.00 Taylor Gregg 542.90 387.09 82.14 112.38 43.00 12.20 Fitzgibbons Rusty 553.58 394.70 83.76 114.59 43.00 12.44 Schulman David 554.70 395.50 83.93 114.82 42.00 12.90 Raskin Rose 556.42 396.73 84.19 115.18 42.00 12.94 Mulicka Al B 559.80 399.14 84.70 115.88 44.33 12.04 Camden Warren 566.02 403.57 85.64 117.17 38.40 14.74 Lee Phoebe 571.18 407.25 86.42 118.23 41.75 13.40 Light Karen L 583.00 415.68 88.21 120.68 50.00 10.60 Worthington Dan 598.37 426.64 90.53 123.86 50.10 10.84 Leslie Bennie A 621.00 442.77 93.96 128.55 44.00 13.50 Fagan Bert Todd 637.20 454.32 96.41 131.90 52.00 10.80 Feistner Merle S 673.20 479.99 101.86 139.35 50.00 12.24 Slaughter Lew 680.69 485.33 102.99 140.90 44.33 14.64 Kenwood Pat 686.69 489.61 103.90 142.14 50.10 12.44 Brandt Leslie 757.44 540.05 114.60 156.79 48.40 14.40 Antrim Forrest N 966.96 689.44 146.30 200.16 62.00 12.24 Hall Gus W 1,212.83 864.75 183.50 251.06 70.40 12.66
Totals: 14,729.71 10,502.28 2,228.60 3,049.05 1,103.14 Average: 12.36
Step by Step Solution
There are 3 Steps involved in it
Step: 1
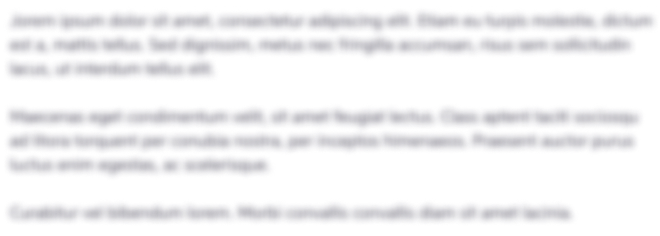
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started