Question
Give an explanation as to why everything in this code is there. /* Final Project: Black Jack Programmers: Brittany Tomblin and Kyle Galante CIT163 2/13/18
Give an explanation as to why everything in this code is there.
/*
Final Project: Black Jack
Programmers: Brittany Tomblin and Kyle Galante
CIT163
2/13/18
Description: This program will simulate a real game of Black Jack with a dealer vs human. This Blackjack game
resembles the casino version of Black Jack this game where betting takes place.
*/
#include
#include
#include
#include
#include
#include
using namespace std;
// Function Prototypes for Black Jack Game
void menu();
void gameComputer(bool CardsDealt[], int HouseHand[], int PlayerHand[], int HouseCardCount, int PlayerCardCount, int x);
void shuffle(bool CardDelt[]);
void displayCard(int Card);
void displayHand(int Hand[], int CardCount);
int nextCard(bool CardsDealt[]);
int scoreHand(int Hand[], int CardCount);
//=============================
// main() =
// The section for BlackJack =
//=============================
int main() {
// For random numbers
srand(time(0));
// Declarations for variables
bool CardsDealt[52]; // Number of cards in the deck (0-51)
int HouseCardCount = 0; // Starting card count for the house
int HouseHand[12];
int PlayerCardCount = 0; // Starting card count for the player
int PlayerHand[12];
int x = 0;
// The menu for which game mode to play in
menu();
shuffle(CardsDealt);
gameComputer(CardsDealt, HouseHand, PlayerHand, HouseCardCount, PlayerCardCount, x);
// For windows to show final screen
system("pause");
return 0; // Return success
}
//=============================
// menu() =
// Selection to play with =
// computer or another user =
//=============================
void menu(){
cout << "**********************" << endl;
cout << "|Welcome to BlackJack|" << endl;
cout << "**********************" << endl;
cout << "Here are the rules: " << endl;
cout << " 1. The goal of the game is to assemble a hand whose numerical point value is as close to 21" << endl;
cout << " without going over. Whoever gets closes to 21 without going over wins. " << endl;
cout << " 2. Aces are worth either 1 point or 11 points at the players dicretion. Picture cards are worth" << endl;
cout << " 10 points. All other cards are worth a number of points equal to their numerical value. " << endl;
cout << " 3. The dealer must draw on 17 or less and hold on 18 or more. " << endl;
cout << "LET'S START THE GAME !" << endl;
}
//=============================
// gameComputer() =
//The Game With the computer =
//=============================
void gameComputer(bool CardsDealt[], int HouseHand[], int PlayerHand[], int HouseCardCount, int PlayerCardCount, int x)
{
//Declaring Variables
char endgame;
endgame = 'n';
int bets;
// Loops for hand (player)
while ((endgame != 'n' || endgame != 'N')) {
// Shuffles the cards
shuffle(CardsDealt); // Shuffles the deck of cards before the beginning of the game
// Two cards are dealt for each player and house
PlayerHand[0] = nextCard(CardsDealt); // first card
HouseHand[0] = nextCard(CardsDealt); // first card
PlayerHand[1] = nextCard(CardsDealt); // second card
HouseHand[1] = nextCard(CardsDealt); // second card
HouseCardCount = 2; // total number of cards
PlayerCardCount = 2; // total number of cards
//Declaring more Variables
char PlayerChoice = '\0'; // allows the player to choose what they want to do with their 2 cards
bool PlayerHits = true;
int PlayerScore = scoreHand(PlayerHand, PlayerCardCount);
// The start of a new hand
cout << "===========================================" << endl;
cout << "= Black Jack 21 =" << endl;
cout << "===========================================" << endl;
cout << "Money: $" << "1000" << endl; // This pulls the users money amount up
cout << "Place your bets!" << endl;
cout << "Bet: ";
cin >> bets;
while (bets > 1000) { // Validation if user enters more money than in account
cout << "You entered a bet more than you have in your account. "
<< "Please enter your bet: ";
cin >> bets;
}
// The start of a new hand
cout << "===========================================" << endl;
cout << "= Black Jack 21 =" << endl;
cout << "===========================================" << endl;
// money betting
PlayerScore = 1000 - bets;
cout << "You bet: $" << bets << " Money left: $" << endl;
// Get Player's hits. Calculate the score and redisplay after each hit.
do { // Do loop only runs once
// Displays the dealt cards, but only the House's second card.
cout << "House's Hand" << endl;
cout << "** "; // House's hidden other card
displayCard(HouseHand[1]); // House has one card currently
cout << endl;
cout << " Player's Hand: Score = " << scoreHand(PlayerHand, PlayerCardCount) << endl;
displayHand(PlayerHand, PlayerCardCount); // shows the player their hand
// Ask the Player whether he wants a hit or to stay or do a double
if (PlayerScore <= 21) { // If player's score is equal to or less than 21
cout << " Hit(h) or stay(s): ";
cin >> PlayerChoice; //Player's choice
}
// If the player choices to hit or stay option
if (PlayerChoice == 'h') { // H is for if the player wants another hit
PlayerHand[PlayerCardCount] = nextCard(CardsDealt);
PlayerCardCount++; // Adds one card to the player's card count
}
else if (PlayerChoice == 's') { // S is for if the player wants to stay
PlayerHits = false; // Does not add another card to the player's hand
}
else {
cout << "Error: Try Again!" << endl; // This is for anything other than D, H, and S.
}
cout << endl;
// Get the Player's current score to update and check for bust.
PlayerScore = scoreHand(PlayerHand, PlayerCardCount);
} // End of do loop
while (PlayerHits && PlayerScore < 22); // If the cards are less than 22 if this is true then the game is over and the player loses
// Once the player is done, a check is taken place to see if busted
if (PlayerScore > 21) {
// The Player busted. The House wins.
cout << "The House Wins!" << endl;
cout << "You lost $" << bets << endl;
}
else {
// If the player didn't bust, then the house takes hits below 17
int HouseScore = scoreHand(HouseHand, HouseCardCount);
while (HouseScore < 17) {
HouseHand[HouseCardCount] = nextCard(CardsDealt);
HouseCardCount++; // Adds to the house's card count
HouseScore = scoreHand(HouseHand, HouseCardCount); // Displays the house's hand
}
bool HouseBusts = (HouseScore > 21);
if (HouseBusts) { // The House busted. Player wins
cout << "You Win!" << endl;
bets = bets * 2;
cout << "You gained $" << bets << endl;
PlayerScore = bets + bets * 2;
}
// Compare scores and determine the winner
else if (PlayerScore == HouseScore) {
// Tie. This is called a "push."
cout << "Tie!" << endl;
cout << "You gained $0" << endl;
PlayerScore = bets + PlayerScore; // Allows the money from the round to be returned to the player and added to their score
}
else if (PlayerScore > HouseScore) {
// The Player wins
cout << "You Win!" << endl;
bets = bets * 2; // Gives money from both the house(player) to the winner(player) of the round
cout << "You gained $" << bets << endl;
PlayerScore = bets + bets * 2; // Money won is added to the winnner's account
}
else {
// The House wins
cout << "The House Wins!" << endl;
cout << "You lost $" << bets << endl << endl;
}
}
cout << "Would you like to play another game or end? yes(y) or no(n) " << endl; // Gives the option to play another round.
cin >> endgame;
}
}
// Shuffling the deck
void shuffle(bool CardsDealt[]) { // How the deck of cards is shuffled
for (int Index = 0; Index < 52; Index++) {
CardsDealt[Index] = false;
}
}
// Displays the card for the player and computer
void displayCard(int Card) { // The ranking of cards in the deck
int Rank = (Card % 13); // Rank of cards is 0-12
if (Rank == 0) {
cout << "Ace";
}
else if (Rank < 9) {
cout << (Rank + 1);
}
else if (Rank == 9) {
cout << "Ten";
}
else if (Rank == 10) {
cout << "Jack";
}
else if (Rank == 11) {
cout << "Queen";
}
else {
cout << "King";
}
int Suit = (Card / 13); // Allows the cards to be placed into different suits
if (Suit == 0) {
cout << " Club";
}
else if (Suit == 1) {
cout << " Diamond";
}
else if (Suit == 2) {
cout << " Heart";
}
else {
cout << " Spade";
}
}
//=============================================
// displayHand() =
// This is were the whole hand is displayed =
// this is different from displayCard because =
// it shows the whole hand not just one card =
//=============================================
void displayHand(int Hand[], int CardCount) {
for (int CardIndex = 0; CardIndex < CardCount; CardIndex++) {
int NextCard = Hand[CardIndex];
displayCard(NextCard);
cout << " ";
}
cout << endl;
}
//============================================
// nextCard() =
// A new card is dealt to the user and the =
// computer based on hit or who's turn =
//============================================
int nextCard(bool CardsDealt[]) {
bool CardDealt = true; // If newcard is required it is true
int NewCard = -1; // It is -1 because there is two less cards in the deck
do {
NewCard = (rand() % 52); // How the new card being dealt is randomized
if (!CardsDealt[NewCard]) { // If no new card is required
CardDealt = false; // No card is dealt
}
} while (CardDealt);
return NewCard; // Gives the new card back to the player
}
//============================================
// scoreHand() =
// The scoreHand is the score for the hand =
// If the user has 1 ace and 1 jack the =
// hand score will be 21 =
//============================================
int scoreHand(int Hand[], int CardCount) {
int AceCount = 0;
int Score = 0;
for (int CardIndex = 0; CardIndex < CardCount; CardIndex++) {
int NextCard = Hand[CardIndex];
int Rank = (NextCard % 13); // Ranking of cards is between 0-12
if (Rank == 0) {
AceCount++; // Different ways that the ace can be counted
Score++;
}
else if (Rank < 9) {
Score = Score + (Rank + 1);
}
else {
Score = Score + 10;
}
}
while (AceCount > 0 && Score < 12) {
AceCount--;
Score = Score + 10;
}
return Score; // Returned the scores from the round
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
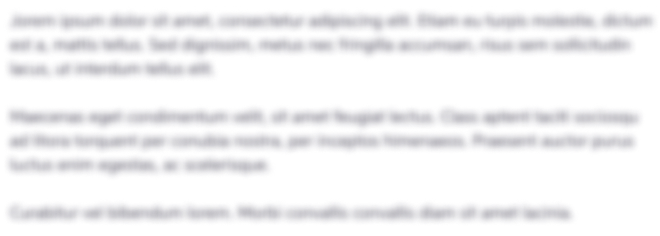
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started