Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Given a TestCellularData.java + csv files. Need to create CellularData.java class that will parse CSV file. package cellularData; public class TestCSVReader { // Uses a
Given a TestCellularData.java + csv files. Need to create CellularData.java class that will parse CSV file.
package cellularData; public class TestCSVReader { //Uses a CSVReader to parse a CSV file. //Adds each parsed line to an instance of the CellularData class. public static void main(String[] args) { // NOTE: Make sure to use relative path instead of specifying the entire // path // (such as /Users/alicew/myworkspace/so_on_and_so_forth). final String FILENAME = "resources/cellular_short_oneDecade.csv"; // Directory path for Mac OS X // TODO: Make sure to test with the full input file as well // final String FILENAME = "resources/cellular.csv"; // Directory path for Mac OS X // final String FILENAME = "resources\\cellular.csv"; // Directory path for Windows OS (i.e. Operating System) // TODO: Create the class CSVReader to parse the CSV data file // The class constructor should only take a string as argument // for the name of the input file. // The constructor should fill the array of country names, year labels, etc. // NOTE: Handle all exceptions in the constructor. // For full credit, do *not* throw exceptions to main. CSVReader parser = new CSVReader(FILENAME); // TODO: In class CSVReader the accessor methods should only return values // at instance variables. String [] countryNames = parser.getCountryNames(); int [] yearLabels = parser.getYearLabels(); double [][] parsedTable = parser.getParsedTable(); // Stores the 2D array of cellular data for all countries. CellularData datatable; int numRows = parsedTable.length; int numColumns = parser.getNumberOfYears(); int startingYear = yearLabels[0]; datatable = new CellularData(numRows, numColumns, startingYear); // From the array that stores parsed information, // add one country at a time to an object of type CellularData. for (int countryIndex = 0; countryIndex < countryNames.length; countryIndex++) { double [] countryData = parsedTable[countryIndex]; datatable.addCountry(countryNames[countryIndex], countryData); } // Display the string representation of the data table. System.out.println(datatable); // Given the cellular_short_oneDecade.csv file, the output is: // Country Name 2005 2006 2007 2008 2009 2010 2011 2012 2013 2014 // Bangladesh 6.29 13.21 23.47 30.17 34.35 44.95 55.19 62.82 74.43 80.04 // "Bahamas, The" 69.21 75.38 109.34 102.79 101.22 118.83 81.56 80.65 76.05 82.30 // Tests finding a country and retrieving subscriptions between a requested period // double totalSubscriptions; int countryNum; int requestedStart = 0, requestedEndYear = 0; try { countryNum = 1; requestedStart = 2005; requestedEndYear = 2014; System.out.printf("Requesting subscriptions for \"%s\" between %d - %d. ", countryNames[countryNum], requestedStart, requestedEndYear); totalSubscriptions = datatable.getNumSubscriptionsInCountryForPeriod(countryNames[countryNum], requestedStart,requestedEndYear); System.out.printf("Total subscriptions = %.2f ", totalSubscriptions); } catch (IllegalArgumentException ex) { System.out.println(ex.getMessage()); } // Given the shorter cellular_short_oneDecade.csv file, the output is: // Requesting subscriptions for ""Bahamas, The"" between 2005 - 2014. // Total subscriptions = 897.33 try { countryNum = 2; requestedStart = 1960; requestedEndYear = 2014; System.out.printf("Requesting subscriptions for \"%s\" between %d - %d. ", countryNames[countryNum], requestedStart, requestedEndYear); totalSubscriptions = datatable.getNumSubscriptionsInCountryForPeriod(countryNames[countryNum], requestedStart,requestedEndYear); System.out.printf("Total subscriptions = %.2f ", totalSubscriptions); } catch (IllegalArgumentException ex) { System.out.println(ex.getMessage()); } // Given the shorter cellular_short_oneDecade.csv file, the output is: // Requesting subscriptions for "Brazil" between 1960 - 2014. // Illegal Argument Request of start year: 1960. // Valid period for Brazil is 2005 to 2014. // Total subscriptions = 948.34 try { countryNum = 0; requestedStart = 1960; requestedEndYear = 2014; System.out.printf("Requesting subscriptions for \"%s\" between %d - %d. ", countryNames[countryNum], requestedStart, requestedEndYear); totalSubscriptions = datatable.getNumSubscriptionsInCountryForPeriod(countryNames[countryNum], requestedStart,requestedEndYear); System.out.printf("Total subscriptions = %.2f ", totalSubscriptions); } catch (IllegalArgumentException ex) { System.out.println(ex.getMessage()); } // Given the full cellular.csv file, the output is: // Requesting subscriptions for "Afghanistan" between 1960 - 2014. // Total subscriptions = 420.07 // TODO: Comment this line if your test input has fewer than countryNum countries. try { countryNum = 100; requestedStart = 1950; requestedEndYear = 2014; System.out.printf("Requesting subscriptions for \"%s\" between %d - %d. ", countryNames[countryNum], requestedStart, requestedEndYear); totalSubscriptions = datatable.getNumSubscriptionsInCountryForPeriod(countryNames[countryNum], requestedStart,requestedEndYear); System.out.printf("Total subscriptions = %.2f ", totalSubscriptions); } catch (IllegalArgumentException ex) { System.out.println(ex.getMessage()); } // Given the full cellular.csv file, the output is: // Requesting subscriptions for "Hong Kong SAR, China" between 1950 - 2014. // Illegal Argument Request of start year: 1950. // Valid period for "Hong Kong SAR, China" is 1960 to 2014. // Total subscriptions between 1960 - 2014 = 2561.16 // // NOTE: For a more user friendly output adjust the invalid requested range to a valid range. // Then, inform the user of the adjusted date and the total subscriptions. // TODO: Use the full cellular.csv for the input file of your additional test cases. // TODO: Also, test for additional cases where the requested range of years is invalid. System.out.println(" Done with TestCSVReader. "); } }
====================================
cellular.csv
World Development Indicators Number of countries,264 Country Name,1960,1961,1962,1963,1964,1965,1966,1967,1968,1969,1970,1971,1972,1973,1974,1975,1976,1977,1978,1979,1980,1981,1982,1983,1984,1985,1986,1987,1988,1989,1990,1991,1992,1993,1994,1995,1996,1997,1998,1999,2000,2001,2002,2003,2004,2005,2006,2007,2008,2009,2010,2011,2012,2013,2014 Afghanistan,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0.112598381,0.865196277,2.498055472,4.826865367,9.833164022,17.71624331,29.22037376,37.89493697,45.77817474,60.32631999,65.45219346,70.66135885,74.88284241 Albania,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0.068840778,0.099059921,0.168397958,0.331772024,0.901406013,11.94887288,26.07553141,33.95706284,39.16395669,47.87802749,60.06734238,73.35038415,58.91235149,78.1845877,85.468247,98.29153459,110.6865316,116.1572081,105.4699657 Algeria,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0.001791178,0.017777422,0.017363261,0.016979454,0.004688247,0.016001794,0.039202273,0.057339703,0.05840281,0.230206295,0.27112703,0.311040075,1.382262358,4.384169991,14.59120666,40.22671305,60.85091077,78.53288666,75.6646235,89.95836607,88.44487548,94.31443964,97.52089467,100.7877205,92.94793073 American Samoa,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,1.411375688,1.774273041,2.316289305,2.364110905,2.410710974,2.548141677,2.683411152,3.170800451,3.463022843,3.7059956,3.466771101,3.552277687,3.796699403,0,0,0,0,0,0,0,0,0,0 Andorra,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,1.307211734,1.278625641,1.250259142,4.424155104,8.538444783,13.44671556,22.12730607,32.14530928,35.99902139,43.27794118,45.77115817,68.60251444,73.82494308,79.48487497,84.27763597,78.1171579,80.2836099,82.06181111,84.06818386,83.53432222,81.50204186,80.70261809,82.64319489 Angola,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0.009672748,0.015532086,0.016472597,0.026485822,0.055130843,0.07474762,0.177638088,0.185322296,0.521366177,0.940444726,2.269621281,4.63174063,9.738161173,17.83989624,28.01098233,36.98368954,42.84657348,48.10120904,59.82618856,61.40627578,61.87329711,63.4792082 Antigua and Barbuda,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,1.850665528,1.938199136,2.021399887,11.17818019,28.3329899,31.65678975,47.73834812,56.98111342,66.08091241,104.1603585,132.0006709,133.1575767,160.0393678,156.3441483,192.5532769,199.6642164,143.0138432,127.0856254,132.0539476 Arab World,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0.001365523,0.002032515,0.002740589,0.00448781,0.011380109,0.017361085,0.019766805,0.028727652,0.038825634,0.056061866,0.063570087,0.081298004,0.105012027,0.197623255,0.355462105,0.611174873,0.935105895,1.490951415,3.178495929,5.746610803,8.526644585,11.59638248,16.83769437,26.79003996,38.84567204,52.5371804,62.92096882,76.15636661,87.62881811,98.87331388,105.0371746,110.0126103,108.7006346 Argentina,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0.007149428,0.036781751,0.075585347,0.138988317,0.329780857,0.701068622,1.163818921,1.891500329,5.62910957,7.396595596,10.54064245,17.58105905,18.08742442,17.4519491,20.6535373,35.27228837,57.32899426,80.81882641,102.7215283,117.2211834,131.1294492,141.383022,149.0906224,156.564756,162.5274217,158.7985611 Armenia,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0.009453508,0.15935483,0.251561377,0.263783931,0.568447429,0.833474947,2.341613166,3.767384533,6.719510373,10.54901346,41.95135986,62.7586975,48.43008603,73.83377008,130.4322327,108.336201,111.9146632,112.4206552,115.9232102 Aruba,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0.029310471,0,0,2.138784453,3.605985937,3.98141538,6.16435217,13.48254011,16.50927821,57.05427692,65.05605558,72.10431377,99.64250268,103.3849507,108.1325002,112.2180618,119.2038996,126.2103374,129.72824,0,131.8565401,134.873823,135.0658893 Australia,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0.027108122,0.19063123,0.560966912,1.081736077,1.682627998,2.835372025,3.892879466,6.807810813,12.37017796,21.7568676,24.66137476,26.16715738,33.18891382,44.45626668,57.12450962,64.2670099,71.9035383,81.50958522,89.76286231,94.70140374,100.0646043,102.1940537,100.7390114,100.4263074,104.610551,105.585695,106.8434974,131.2305468 Austria,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0.128856131,0.25189156,0.345358749,0.48515954,0.664667026,0.96086037,1.493599184,2.211730703,2.805498884,3.504549191,4.80296973,7.475118128,14.47070198,28.62886002,53.07787628,76.26932886,81.26031128,83.24472516,89.33569564,97.54481697,105.1755856,112.124675,119.2569828,129.6551516,136.57901,145.6928199,154.426039,160.5397387,156.2304116,151.9112515 Azerbaijan,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0.006514549,0.077212068,0.216497822,0.504939253,0.814081353,4.597452067,5.178780011,8.907406533,9.592056203,12.62937463,17.20615651,26.18119583,38.35542641,51.52721935,73.75810024,86.32194952,100.0593201,109.9720704,108.7683381,107.6134072,110.9053634 "Bahamas, The",0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0.749791291,0.773644017,0.977087303,0.885634717,0,1.464024281,1.744231135,2.161446077,2.784158716,5.422195868,10.58708553,19.98481873,39.3992344,38.72582567,57.69447891,69.21279415,75.37855087,109.340767,102.7875065,101.2186453,118.8292307,81.5628489,80.65383375,76.05187427,82.29635806 Bahrain,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0.142563513,0.381286664,0.620306327,0.883522952,1.037818786,1.442965201,1.852315921,2.120546339,3.207749877,4.895960832,6.913956774,9.799631738,14.90809458,20.82466731,30.78644018,42.87476619,53.10146463,57.39322693,79.19074229,87.21698081,95.42373897,108.1005238,129.0979339,117.6607732,125.2084477,131.009991,161.1670576,165.9088811,173.2739335 Bangladesh,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0.000222359,0.00043517,0.000940619,0.0020856,0.00326795,0.020809104,0.058833439,0.114644643,0.210751714,0.385958523,0.784635571,0.980702181,1.969454675,6.28776238,13.20573922,23.46762823,30.16828408,34.35334451,44.94535882,55.19256723,62.82023906,74.42964608,80.03535051 Barbados,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0.186797348,0.305024084,0.59607052,1.130539817,1.753272662,2.380970426,3.028276003,4.521988167,7.628652994,10.6542161,19.79567344,36.06098158,51.69027189,73.5247332,75.37065739,86.2492407,93.23830793,103.972136,120.8077962,124.8452189,123.4606322,123.3298378,108.1027529,128.7304328 Belarus,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0.003162103,0.016869419,0.057875715,0.064472565,0.080697147,0.120576083,0.23375711,0.494446704,1.394421246,4.695385079,11.42668966,23.03795343,42.41718896,61.95195003,72.61067076,85.05828031,101.6840082,108.8697059,113.1688625,113.5179254,118.786176,122.5011386 Belgium,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0.038348549,0.072839954,0.192853855,0.309387231,0.429735061,0.513631256,0.611545879,0.671587939,1.264320986,2.315095365,4.694050625,9.548274196,17.1799361,31.11171947,54.81877375,74.72253301,78.36155617,82.86829993,87.46025396,91.40294897,93.00741585,100.5624899,105.283158,108.4032585,111.0841886,113.5309512,111.3315482,110.9031799,114.2699575 Belize,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0.201592581,0.411172831,0.747480214,1.028369629,1.164387324,1.570531759,2.842663676,7.046515722,15.96872731,20.54645981,23.38066005,28.29761545,35.30450132,42.2961808,41.34020042,54.51721037,53.74564807,62.93070205,70.31965347,53.20712214,52.61072612,50.712566 Benin,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0.017541931,0.043828702,0.067517635,0.096020877,0.107840809,0.79828865,1.742181889,2.950472734,3.08093958,5.797473518,7.28722342,12.50317546,23.56334604,40.40173435,54.46885832,74.39604921,79.40049868,83.65431589,93.25782193,99.65221977 Bermuda,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,1.253828806,1.859593966,2.393935364,3.200793585,5.592013289,8.390338101,10.29850017,12.9329206,16.57633243,20.18755219,20.46133926,20.68844789,21.12459598,47.32383702,62.83281758,76.66911799,82.19904267,93.41436498,106.9568452,122.1643187,131.1485527,135.794676,0,139.5363101,144.3197992,90.88006599 Bhutan,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0.365843964,3.019121524,5.53491068,12.33202317,21.99686472,36.61427504,48.10756491,54.99993723,66.37918152,75.6097824,72.19831102,82.07006186 Bolivia,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0.004240494,0.021773635,0.036460894,0.054342489,0.094677895,0.427823762,1.484397923,2.935775674,5.050749735,6.858168503,8.996551647,11.57177993,14.18292347,19.60018982,25.88431131,30.21985533,33.63225131,51.23601575,64.68655431,70.68598048,80.90771949,90.44349501,97.69945273,96.33724828 Bosnia and Herzegovina,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0.043034507,0.254525031,0.691629718,1.402104049,2.435501689,11.46353529,19.21141303,27.58857728,36.21150774,41.09375467,48.7159617,63.34032541,82.33282857,84.52795238,80.87078571,82.60007887,87.57471473,91.09530785,91.27895029 Botswana,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0.897675617,5.33356832,12.65769422,18.63146249,18.36751842,24.28121327,28.18941102,30.05546952,43.41214719,60.13830503,76.83593118,96.02329234,120.0102471,145.9838697,153.7856491,160.6410528,167.2975939 Brazil,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0.000445712,0.004403447,0.020698847,0.115915349,0.360031692,0.794074075,1.519628432,2.725767586,4.347740579,8.739617433,13.28797717,16.24346532,19.44379918,25.51445011,35.65289881,46.31418452,53.11025849,63.67475185,78.5549801,87.54187651,100.8810115,119.0023853,125.0018521,135.3050481,138.9514906
====================================
cellular_short_oneDecade.csv
Number of countries,4 Country Name,2005,2006,2007,2008,2009,2010,2011,2012,2013,2014 Bangladesh,6.28776238,13.20573922,23.46762823,30.16828408,34.35334451,44.94535882,55.19256723,62.82023906,74.42964608,80.03535051 "Bahamas, The",69.21279415,75.37855087,109.340767,102.7875065,101.2186453,118.8292307,81.5628489,80.65383375,76.05187427,82.29635806 Brazil,46.31418452,53.11025849,63.67475185,78.5549801,87.54187651,100.8810115,119.0023853,125.0018521,135.3050481,138.9514906 Germany,94.55486999,102.2828888,115.1403608,126.5575074,126.2280577,106.4836959,109.6595675,111.5940398,120.9211651,120.4201855
=======================
Step by Step Solution
There are 3 Steps involved in it
Step: 1
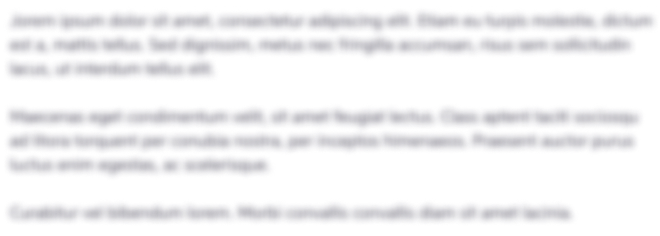
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started