Answered step by step
Verified Expert Solution
Question
1 Approved Answer
GIVEN CODE public class ArrayLongestPlateau { /** * longestPlateau() returns the longest plateau of an array of ints. * * @return an array int[3] of
GIVEN CODE
public class ArrayLongestPlateau { /** * longestPlateau() returns the longest plateau of an array of ints. * * @return an array int[3] of the form {value, start, len} representing the longest plateau of * ints[] as a length len contiguous subarray starting at index start with common * element values value. * * For example, on the input array [2, 3, 3, 3, 3, 6, 6, 1, 1, 1], it returns [6, 5, 2], * indicating the longest plateau of this array is the subarray [6, 6]; it starts at * index 5 and has length 2. * * @param ints * the input array. */ public static int[] longestPlateau(int[] ints) { // TODO: Replace the following one line stub with your solution. Ours takes linear time and is // 24 lines long, not counting blank/comment lines or lines already present in this file. return new int[] { 0, 0, 0 }; } /** * main() runs test cases on your longestPlateau() method. Prints summary * information on basic operations and halts with an error (and a stack * trace) if any of the tests fail. */ public static void main(String[] args) { String result; System.out.println("Let's find longest plateaus of arrays! "); int[] test1 = { 4, 1, 1, 6, 6, 6, 6, 1, 1 }; System.out.println("longest plateau of " + TestHelper.stringInts(test1) + ":"); result = TestHelper.stringInts(longestPlateau(test1)); System.out.println("[ value , start , len ] = " + result + " "); TestHelper.verify(result.equals("[ 6 , 3 , 4 ]"), "Wrong: that's not the longest plateau!!! No chocolate."); int[] test2 = { 3, 3, 1, 2, 4, 2, 1, 1, 1, 1 }; System.out.println("longest plateau of " + TestHelper.stringInts(test2) + ":"); result = TestHelper.stringInts(longestPlateau(test2)); System.out.println("[ value , start , len ] = " + result + " "); TestHelper.verify(result.equals( "[ 3 , 0 , 2 ]"), "Wrong: that's not the longest plateau!!! No chocolate."); int[] test3 = { 3, 3, 1, 2, 4, 0, 1, 1, 1, 1 }; System.out.println("longest plateau of " + TestHelper.stringInts(test3) + ":"); result = TestHelper.stringInts(longestPlateau(test3)); System.out.println("[ value , start , len ] = " + result + " "); TestHelper.verify(result.equals("[ 1 , 6 , 4 ]"), "Wrong: that's not the longest plateau!!! No chocolate."); int[] test4 = { 3, 3, 3, 4, 1, 2, 4, 4, 0, 1 }; System.out.println("longest plateau of " + TestHelper.stringInts(test4) + ":"); result = TestHelper.stringInts(longestPlateau(test4)); System.out.println("[ value , start , len ] = " + result + " "); TestHelper.verify(result.equals("[ 4 , 6 , 2 ]"), "Wrong: that's not the longest plateau!!! No chocolate."); int[] test5 = { 7, 7, 7, 7, 9, 8, 2, 5, 5, 5, 0, 1 }; System.out.println("longest plateau of " + TestHelper.stringInts(test5) + ":"); result = TestHelper.stringInts(longestPlateau(test5)); System.out.println("[ value , start , len ] = " + result + " "); TestHelper.verify(result.equals("[ 5 , 7 , 3 ]"), "Wrong: that's not the longest plateau!!! No chocolate."); int[] test6 = { 4 }; System.out.println("longest plateau of " + TestHelper.stringInts(test6) + ":"); result = TestHelper.stringInts(longestPlateau(test6)); System.out.println("[ value , start , len ] = " + result + " "); TestHelper.verify(result.equals("[ 4 , 0 , 1 ]"), "Wrong: that's not the longest plateau!!! No chocolate."); int[] test7 = { 4, 4, 4, 5, 5, 5, 6, 6 }; System.out.println("longest plateau of " + TestHelper.stringInts(test7) + ":"); result = TestHelper.stringInts(longestPlateau(test7)); System.out.println("[ value , start , len ] = " + result + " "); TestHelper.verify(result.equals("[ 6 , 6 , 2 ]"), "Wrong: that's not the longest plateau!!! No chocolate."); System.out.println(" Additional tests done by the student or TA: "); // Insert your additional test cases here. } }
-------------------------- HELPER CLASS---------------
public class TestHelper { /** * stringInts() converts an array of ints to a String. * * @return a String representation of input int array ints[0..n-1] formatted * as "[ ints[0] , ints[1] , ... , ints[n-1] ]". in O(n) time. * * @param ints * the input array. */ public static String stringInts(int[] ints) { /* * To ensure O(n) time, we can use StringBuilder with repeated appends. * If we use String with repeated concatenations, it will take O(n^2) * time, and it will wastefully create O(n) distinct intermediate * strings. This is because String is immutable. But the mutable * StringBuilder is internally implemented as a dynamically expandable * array, representing a character sequence. See the Java API. We prefer * StringBuilder over the older version called StringBuffer. */ StringBuilder s = new StringBuilder("["); for (int i = 0; i Consider a non-empty int array ints. A contiguous subarray ints[ start .. start + len -1 ] (with starting index start and length len) is called a flat if all elements of that subarray are equal. Furthermore, such a subarray is called a plateau if it is flat and each of the elements ints[start-1] and ints[start + len) that immediately proceed/succeed the subarray are either nonexistent (i.e., out of array's index range) or are strictly smaller than the elements of the subarray. Your task includes the design of a public static method longest Plateau(int[] ints) that returns (a compact description of) the longest plateau of the input array ints. You may break ties arbitrarily if ints has more than one longest plateau. The return type should be a 3-element array representing { value, start, len}: The first indicates common element value ints[start] of the plateau, the second its starting index, the third its length. Implement the longestPlateau() method in the provided ArrayLongest Plateau class so that it performs as indicated. The main() method in this class runs some test cases on longestPlateau(). You should also add a few nontrivial and interesting test cases of your own at the end of main()
Step by Step Solution
There are 3 Steps involved in it
Step: 1
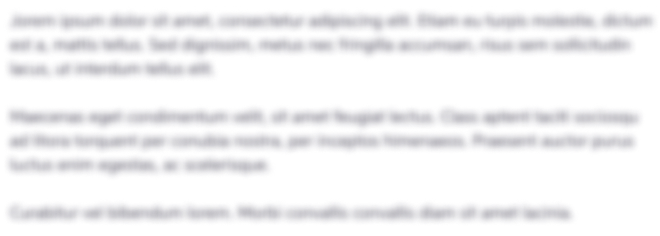
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started