Question
Given four classes: Progression, ArithmeticProgression, FibonacciProgesssion, and TestProgression, you are first to test these classes and go over the code to understand what they do.
Given four classes: Progression, ArithmeticProgression, FibonacciProgesssion, and TestProgression, you are first to test these classes and go over the code to understand what they do. Then work on the following requirements (For the testing of new implementations: you would use ONLY one test classyou just need to add code in the current test class): 1) Modify Progression class to an abstract class such that method advance() should be an abstract method (with declaration only; no implementation) which should be implemented in the subclasses. Make necessary changes in other classes. Test your modified program so the outputs should be same. 2) Define (i. e., add) a new Java class that extends the abstract class from the previous step so that each value in the (newly-defined) progression is the absolute value of the difference between the previous two values. You should include a default constructor that starts with 7 and 99 as the first two values and a parametric constructor that starts with a specified pair of numbers as the first two values. Test this new class and the two constructors in the test class The output sequence (if starting with 7 and 99) for this new progression is: 7 99 92 7 85 78 7 71 ------------------------------- public class ArithmeticProgression extends Progression {
protected long increment;
/** Constructs progression 0, 1, 2, ... */ public ArithmeticProgression() { this(1, 0); } // start at 0 with increment of 1
/** Constructs progression 0, stepsize, 2*stepsize, ... */ public ArithmeticProgression(long stepsize) { this(stepsize, 0); } // start at 0
/** Constructs arithmetic progression with arbitrary start and increment. */ public ArithmeticProgression(long stepsize, long start) { super(start); increment = stepsize; }
/** Adds the arithmetic increment to the current value. */ protected void advance() { current += increment; } } ------------------ public class FibonacciProgression extends Progression {
protected long prev;
/** Constructs traditional Fibonacci, starting 0, 1, 1, 2, 3, ... */ public FibonacciProgression() { this(0, 1); }
/** Constructs generalized Fibonacci, with give first and second values. */ public FibonacciProgression(long first, long second) { super(first); prev = second - first; // fictitious value preceding the first }
/** Replaces (prev,current) with (current, current+prev). */ protected void advance() { long temp = prev; prev = current; current += temp; } }
-------------------- public class GeometricProgression extends Progression {
protected long base;
/** Constructs progression 1, 2, 4, 8, 16, ... */ public GeometricProgression() { this(2, 1); } // start at 1 with base of 2
/** Constructs progression 1, b, b^2, b^3, b^4, ... for base b. */ public GeometricProgression(long b) { this(b, 1); } // start at 1
/** Constructs geometric progression with arbitrary base and start. */ public GeometricProgression(long b, long start) { super(start); base = b; }
/** Multiplies the current value by the geometric base. */ protected void advance() { current *= base; // multiply current by the geometric base } }
---------------------------- /** Generates a simple progression. By default: 0, 1, 2, ... */ public class Progression {
// instance variable protected long current;
/** Constructs a progression starting at zero. */ public Progression() { this(0); }
/** Constructs a progression with given start value. */ public Progression(long start) { current = start; }
/** Returns the next value of the progression. */ public long nextValue() { long answer = current; advance(); // this protected call is responsible for advancing the current value return answer; }
/** Advances the current value to the next value of the progression. */ protected void advance() { current++; }
/** Prints the next n values of the progression, separated by spaces. */ public void printProgression(int n) { System.out.print(nextValue()); // print first value without leading space for (int j=1; j < n; j++) System.out.print(" " + nextValue()); // print leading space before others System.out.println(); // end the line } } -----------------
/** Test program for the progression hierarchy. */ public class TestProgression { public static void main(String[] args) { Progression prog; // test ArithmeticProgression System.out.print("Arithmetic progression with default increment: "); prog = new ArithmeticProgression(); prog.printProgression(10); System.out.print("Arithmetic progression with increment 5: "); prog = new ArithmeticProgression(5); prog.printProgression(10); System.out.print("Arithmetic progression with start 2: "); prog = new ArithmeticProgression(5, 2); prog.printProgression(10); // test GeometricProgression System.out.print("Geometric progression with default base: "); prog = new GeometricProgression(); prog.printProgression(10); System.out.print("Geometric progression with base 3: "); prog = new GeometricProgression(3); prog.printProgression(10); // test FibonacciProgression System.out.print("Fibonacci progression with default start values: "); prog = new FibonacciProgression(); prog.printProgression(10); System.out.print("Fibonacci progression with start values 4 and 6: "); prog = new FibonacciProgression(4, 6); prog.printProgression(8); } }
/* Output from main method:
Arithmetic progression with default increment: 0 1 2 3 4 5 6 7 8 9 Arithmetic progression with increment 5: 0 5 10 15 20 25 30 35 40 45 Arithmetic progression with start 2: 2 7 12 17 22 27 32 37 42 47 Geometric progression with default base: 1 2 4 8 16 32 64 128 256 512 Geometric progression with base 3: 1 3 9 27 81 243 729 2187 6561 19683 Fibonacci progression with default start values: 0 1 1 2 3 5 8 13 21 34 Fibonacci progression with start values 4 and 6: 4 6 10 16 26 42 68 110
*/
Step by Step Solution
There are 3 Steps involved in it
Step: 1
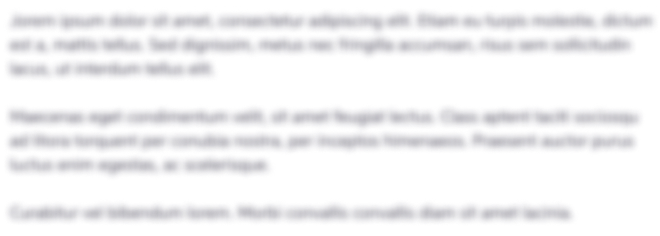
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started