Question
Given main(), define the Team class (in file Team.java). Forclass method getWinPercentage(), the formula is: wins / (wins + losses). Note: Use casting to prevent
Given main(), define the Team class (in file Team.java). Forclass method getWinPercentage(), the formula is:
wins / (wins + losses). Note: Use casting to prevent integerdivision.
For class method printStanding(), output the win percentage ofthe team with two digits after the decimal point and whether theteam has a winning or losing average. A team has a winning averageif the win percentage is 0.5 or greater.
Ex: If the input is:
Ravens133
where Ravens is the team's name, 13 is the number of team wins,and 3 is the number of team losses, the output is:
Win percentage: 0.81Congratulations, Team Ravens has a winning average!
Ex: If the input is:
Angels8082
the output is:
Win percentage: 0.49Team Angels has a losing average.
On the homework this part can not change onWinningteam.java
import java.util.Scanner;
public class WinningTeam {
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
Team team = new Team();
String name = scnr.next();
int wins = scnr.nextInt();
int losses = scnr.nextInt();
team.setName(name);
team.setWins(wins);
team.setLosses(losses);
team.printStanding();
}
}
This is the part that i need to change onTeam.java
public class Team {
private String teamName;
private int teamWins;
private int teamLosses;
public String getTeamName() {
return teamName;
}
public void setTeamName(String teamName) {
this.teamName = teamName;
}
public int getTeamWins() {
return teamWins;
}
public void setTeamWins(int teamWins) {
this.teamWins = teamWins;
}
public int getTeamLosses() {
return teamLosses;
}
public void setTeamLosses(int teamLosses) {
this.teamLosses = teamLosses;
}
public double getWinPercentage() {
return teamWins / (double) (teamWins + teamLosses);
}
}
Step by Step Solution
3.44 Rating (157 Votes )
There are 3 Steps involved in it
Step: 1
Teamjava public class Team private String teamName private int teamWins private int teamLosses method to return the name of the team public String get...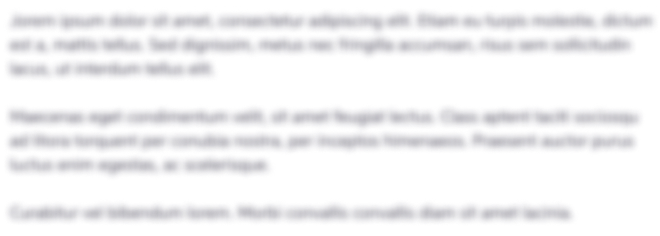
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started