Question
Given the code fragment: struct NodeType { int data; NodeType* next; }; NodeType* p; NodeType* q; p = new NodeType; p->data = 12; p->next =
Given the code fragment:
struct NodeType
{
int data;
NodeType* next;
};
NodeType* p;
NodeType* q;
p = new NodeType;
p->data = 12;
p->next = NULL;
q = new NodeType;
q->data = 5;
q->next = p;
Which of the following expressions has the value 12?
i) q
ii) q->data
iii) q->next->data
iv) q->next
v) None of the above
2) Given the declarations:
struct ListNode
{
float volume;
ListNode* next;
};
ListNode* headPtr;
Assume that headPtr is the external pointer to a linked list of many nodes. Which statement deletes the first node? (Ignore deallocation of the node.)
i) headPtr = headPtr->next;
ii) *headPtr = headPtr->next;
iii) headPtr->next = headPtr->next->next;
iv) headPtr->(*next) = headPtr->next->next;
v) none of the above
3) Given the declarations:
struct ListNode
{
float volume;
ListNode* next;
};
ListNode* headPtr;
Assume that headPtr is the external pointer to a linked list of many nodes. Which statement deletes the second node? (Ignore deallocation of the node.)
i) headPtr = headPtr->next;
ii) *headPtr = headPtr->next;
iii) headPtr->next = headPtr->next->next;
iv) headPtr->(*next) = headPtr->next->next;
v) none of the above
4) Given the declarations:
struct NodeType
{
int score;
NodeType* next;
};
NodeType* somePtr;
Write a single C++ statement that will deallocate (that is, return to the free store) the dynamic node that is currently pointed to by somePtr: __________________
5) Given the declarations:
struct NodeType
{
int data;
NodeType* next;
};
NodeType* headPtr; //External pointer to a linked list
NodeType* p;
the following code sums all the components in a list:
sum = 0;
p = headPtr;
while ( /* Loop condition */ )
{
sum = sum + p->data;
p = p->next;
}
Write the missing loop condition: ____________________
Step by Step Solution
There are 3 Steps involved in it
Step: 1
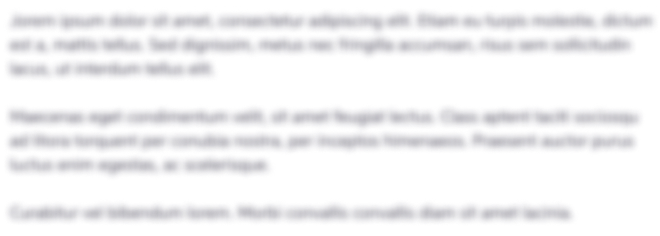
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started