Question
Given the following code, what is output by the method call, mystery(6 * 8)? public static void mystery (int x[]) { System.out.println(A); } public static
Given the following code, what is output by the method call, mystery(6 * 8)?
public static void mystery (int x[]) { System.out.println("A"); } public static void mystery (int x) { System.out.println("B"); } public static void mystery (String x) { System.out.println("C"); }
A
B
C
CA
CB
Which of the following is true about overloaded methods?
Java cannot use a method's return type to tell two overloaded methods apart.
Java cannot use a method's parameters to tell two overloaded methods apart.
You can only overload methods that have parameters.
All overloaded methods must have different names.
None of the above
Given the following code, what is output by the method call, mystery (5, 7.0015)?
public static void mystery (int a) { System.out.println("A"); } public static void mystery (double a) { System.out.println("B"); } public static void mystery (int a, double b) { System.out.println("C"); } public static void mystery (double a, int b) { System.out.println("D"); }
A
B
C
D
Nothing is printed - there is an error.
Consider the following methods:
public static double doStuff(int a) { return a/2; } public static double doStuff(double val) { return val/10; }
What is output by the following?
System.out.println(doStuff(5) + doStuff(5.0));
2.00.5
2.50.5
22.5
2.5
5
What is output to the screen by the following code?
System.out.println("Sum=" + 4 + 5);
Sum= 5 4
Sum=45
Sum=9
Sum= 4 5
Sum=54
What is output to the screen by the following code?
System.out.print(21/5);
3.5
4
4.2
5
5.5
Consider the following three classes: Clothing, Socks, and Sweater. Which would you choose be an abstract class?
Clothing
Socks
Sweater
Socks and Sweater
all of the above
Which of the following keywords allows a child class to access the overridden methods in a parent class?
extends
new
super
this
None of the above
Questions 9 and 10 refer to the following code:
public abstract class Phone { abstract void dial(); } public class MobilePhone extends Phone { } public class RotaryPhone extends Phone { public void dial () { //code not shown } }
Which of the following statements is true?
RotaryPhone can be instantiated.
MobilePhone can be instantiated.
RotaryPhone cannot be instantiated.
Neither can be instantiated since you cannot extend an abstract class.
Neither can be instantiated since they do not include constructors.
Which of the following statements is true?
A Phone object can access methods in MobilePhone.
A RotaryPhone object can access methods in MobilePhone.
RotaryPhone inherits from Phone and MobilePhone.
RotaryPhone inherits from Phone.
None of the above.
Suppose a class implements the Comparable interface. Which of the following methods must the class include?
length
charAt
substring
indexOf
compareTo
Questions 12-14 refer to the following:
public class A { public A () { System.out.print(one ); } public A (int z) { System.out.print(two ); } public void doStuff() { System.out.print(six ); } } public class B extends A { public B () { super (); System.out.print(three ); } public B (int val) { super (val); System.out.print(four ); } }
What is printed when the following line of code is executed?
B b = new B();
one three
two four
four two
three two
one four
What is printed when the following line of code is executed?
A a = new B(5);
four
two four
one
two
four one
Assume that variable b has been instantiated as a B object. What is printed when the following line of code is executed?
b.doStuff();
six
four
five
two
three
Which of the following is true about interfaces:
All methods in an interface must be abstract.
A class can only implement one interface.
An interface can have only non abstract methods.
Can not contain constants but can have variables.
None of the above.
What is the rule for a super reference in a constructor?
It must be in the parent class' constructor.
It must be the last line of the constructor in the child class.
It must be the first line of the constructor in the child class.
Only one child class can use it.
You cannot use super in a constructor.
Consider the following class definition.
public class WhatsIt { private int length; private int width; public int getArea () { // implementation not shown } private int getPerimeter () { // implementation not shown } }
A child class Thingy that extends WhatsIt would have access to:
getArea()
getPerimeter()
width, length, getPerimeter()
width, length, getArea()
all of the above
Questions 18 - 20 pertain to the following class, Point:
public class Point { private double x; private double y; public Point() { this (0, 0); } public Point(double a, double b) { /* missing code */ } // ... other methods not shown }
Which of the following correctly implements the equals method?
public boolean equals(Point p) { return (x == Point.x && y == Point.y); }
public void equals(Point p) { System.out.println(x == p.x && y == p.y); }
public boolean equals(Point p) { System.out.println(x == p.x && y == p.y); }
public boolean equals(Point p) { return (x == p.x && y == p.y ); }
public void equals(Point p) { return (x == p.x && y == p.y ); }
The default constructor sets x and y to (0, 0) by calling the second constructor. What could be used to replace /* missing code */ so that this works as intended?
a = 0; b = 0;
this(0, 0);
this (x, y);
a = x; b = y;
x = a; y = b;
Which of the following correctly implements a mutator method for Point?
public double getX() { return x; }
public double getX() { return a; }
public void setCoordinates (double a, double b) { x = a; y = b; }
public void setCoordinates (double a, double b) { Point p = new Point(a,b);
Given the following code, what is output by the method call, mystery(6 * 8)?
public static void mystery (int x[]) { System.out.println("A"); } public static void mystery (int x) { System.out.println("B"); } public static void mystery (String x) { System.out.println("C"); }
A
B
C
CA
CB
Which of the following is true about overloaded methods?
Java cannot use a method's return type to tell two overloaded methods apart.
Java cannot use a method's parameters to tell two overloaded methods apart.
You can only overload methods that have parameters.
All overloaded methods must have different names.
None of the above
Given the following code, what is output by the method call, mystery (5, 7.0015)?
public static void mystery (int a) { System.out.println("A"); } public static void mystery (double a) { System.out.println("B"); } public static void mystery (int a, double b) { System.out.println("C"); } public static void mystery (double a, int b) { System.out.println("D"); }
A
B
C
D
Nothing is printed - there is an error.
Consider the following methods:
public static double doStuff(int a) { return a/2; } public static double doStuff(double val) { return val/10; }
What is output by the following?
System.out.println(doStuff(5) + doStuff(5.0));
2.00.5
2.50.5
22.5
2.5
5
What is output to the screen by the following code?
System.out.println("Sum=" + 4 + 5);
Sum= 5 4
Sum=45
Sum=9
Sum= 4 5
Sum=54
What is output to the screen by the following code?
System.out.print(21/5);
3.5
4
4.2
5
5.5
Consider the following three classes: Clothing, Socks, and Sweater. Which would you choose be an abstract class?
Clothing
Socks
Sweater
Socks and Sweater
all of the above
Which of the following keywords allows a child class to access the overridden methods in a parent class?
extends
new
super
this
None of the above
Questions 9 and 10 refer to the following code:
public abstract class Phone { abstract void dial(); } public class MobilePhone extends Phone { } public class RotaryPhone extends Phone { public void dial () { //code not shown } }
Which of the following statements is true?
RotaryPhone can be instantiated.
MobilePhone can be instantiated.
RotaryPhone cannot be instantiated.
Neither can be instantiated since you cannot extend an abstract class.
Neither can be instantiated since they do not include constructors.
Which of the following statements is true?
A Phone object can access methods in MobilePhone.
A RotaryPhone object can access methods in MobilePhone.
RotaryPhone inherits from Phone and MobilePhone.
RotaryPhone inherits from Phone.
None of the above.
Suppose a class implements the Comparable interface. Which of the following methods must the class include?
length
charAt
substring
indexOf
compareTo
Questions 12-14 refer to the following:
public class A { public A () { System.out.print(one ); } public A (int z) { System.out.print(two ); } public void doStuff() { System.out.print(six ); } } public class B extends A { public B () { super (); System.out.print(three ); } public B (int val) { super (val); System.out.print(four ); } }
What is printed when the following line of code is executed?
B b = new B();
one three
two four
four two
three two
one four
What is printed when the following line of code is executed?
A a = new B(5);
four
two four
one
two
four one
Assume that variable b has been instantiated as a B object. What is printed when the following line of code is executed?
b.doStuff();
six
four
five
two
three
Which of the following is true about interfaces:
All methods in an interface must be abstract.
A class can only implement one interface.
An interface can have only non abstract methods.
Can not contain constants but can have variables.
None of the above.
What is the rule for a super reference in a constructor?
It must be in the parent class' constructor.
It must be the last line of the constructor in the child class.
It must be the first line of the constructor in the child class.
Only one child class can use it.
You cannot use super in a constructor.
Consider the following class definition.
public class WhatsIt { private int length; private int width; public int getArea () { // implementation not shown } private int getPerimeter () { // implementation not shown } }
A child class Thingy that extends WhatsIt would have access to:
getArea()
getPerimeter()
width, length, getPerimeter()
width, length, getArea()
all of the above
Questions 18 - 20 pertain to the following class, Point:
public class Point { private double x; private double y; public Point() { this (0, 0); } public Point(double a, double b) { /* missing code */ } // ... other methods not shown }
Which of the following correctly implements the equals method?
public boolean equals(Point p) { return (x == Point.x && y == Point.y); }
public void equals(Point p) { System.out.println(x == p.x && y == p.y); }
public boolean equals(Point p) { System.out.println(x == p.x && y == p.y); }
public boolean equals(Point p) { return (x == p.x && y == p.y ); }
public void equals(Point p) { return (x == p.x && y == p.y ); }
The default constructor sets x and y to (0, 0) by calling the second constructor. What could be used to replace /* missing code */ so that this works as intended?
a = 0; b = 0;
this(0, 0);
this (x, y);
a = x; b = y;
x = a; y = b;
Which of the following correctly implements a mutator method for Point?
public double getX() { return x; }
public double getX() { return a; }
public void setCoordinates (double a, double b) { x = a; y = b; }
public void setCoordinates (double a, double b) { Point p = new Point(a,b); }
None of the above
}
None of the above
Step by Step Solution
There are 3 Steps involved in it
Step: 1
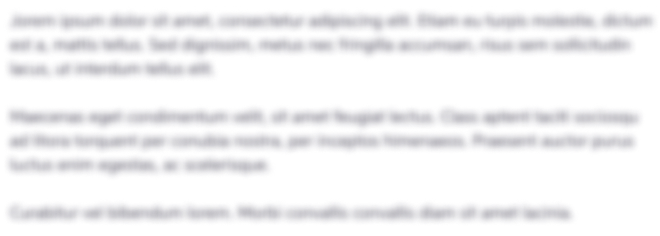
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started