Question
Given the following program: #include #include using namespace std; struct point_t{ double x_coord, y_coord; }; class Point{ public: Point(); void set_point_data (double, double); double distance(Point);
Given the following program:
#include
#include
using namespace std;
struct point_t{
double x_coord, y_coord;
};
class Point{
public:
Point();
void set_point_data (double, double);
double distance(Point);
private:
point_t pt;
};
Point::Point(){
pt.x_coord = 0.0;
pt.y_coord = 0.0;
}
void Point::set_point_data(double x, double y){
pt.x_coord = x;
pt.y_coord = y;
}
double Point::distance(Point other){
// This is sqrt(x^2 + y^2)
return sqrt((pt.x_coord - other.pt.x_coord)*(pt.x_coord - other.pt.x_coord)
+ (pt.y_coord - other.pt.y_coord)*(pt.y_coord - other.pt.y_coord));
}
int main(){
Point point1, point2;
point1.set_point_data (2.0, -3.0);
point2.set_point_data (5.0, 1.0);
cout << point1.distance(point1) << ',';
cout << point1.distance(point2);
return 0;
}
What is the output?
a) 5,5 b) 0,25 c) 1,5 d) 5,0 e) 0,5
Given the following program:
#include
using namespace std;
class Circle{
public:
Circle(){
double radius = 5;
}
void setRadius(double radius_temp){
radius = radius_temp;
}
double getRadius() const{
return radius;
}
double radius;
};
int main(){
Circle bob;
cout << bob.getRadius() << ',';
bob.setRadius(10);
cout << bob.getRadius() << ',';
bob.radius = 20;
cout << bob.getRadius() << ',';
cout << bob.radius;
return 0;
}
What is the output?
Circle the correct answer: ? denotes an undefined value
a) 5,10,20,20 b) ?,10,20,20 c) ?,?,20,20 d) ?,?,?,? e) 5,5,10,10
Given the following program:
#include
#include
using namespace std;
int w = 99;
int f(int &w, int x, int &z){
static int g = 1;
if (w < z){
int t = z;
z = w;
w = t;
}else{
g++;
}
x = 1;
return (z);
z = 13;
}
int main(){
int a = 5;
int b = 10;
int t = 20;
int d = 30;
int w = 100;
w = f(a, b, t);
cout << a << ',' << b << ',' << t << ',' << d << ',' << w << endl;
return 0;
}
What is the output?
a) 5,5,10,5,5 b) 5,10,20,30,100 c) 20,10,5,30,5 d) 5,0,10,20,30 e) 5,8,10,12,13
Given the following program fragment, what is the output if a 4 is input by the user when asked to enter a number?
int num;
int total = 0;
cout << "Enter a number from 1 to 10: ";
cin >> num;
switch (num)
{
case 1:
case 2: total = 5;
case 3: total = 10;
case 4: total = total + 3;
case 8: total = total + 6;
default: total = total + 4;
}
cout << total << endl;
a. | 0 |
b. | 3 |
c. | 13 |
d. | 28 |
e. | None of these |
What is the output of the following program segment?
void maxValue (int value1, int value2, int max){
if (value1 > value2){
max = value1;
}else{
max = value2;
}
}
int main(){
int max = 0;
maxValue (1, 2, max);
cout << "max is " << max << endl;
return 0;
}
Output:
What is the output of the following program segment?
class C
{
public:
C() { cout << Object C Instantiated << endl;}
C(int input) { cout << Object has value of << input << endl; }
C(double input) { cout << Object has value of << input << endl; }
~C() { cout << Object C destroyed << endl;}
};
int main()
{
C X;
C X1(3);
C X2(3.5);
}
Output?
What is the output of the following code fragment?
int * myFunction (int a [ ], int size){
int * temp = new int [size];
for (int i = 0; i < size; i++)
temp[i] = 2 * a[i];
return temp;
}
int main()
{
int a[] = {1, 2, 3, 4, 5};
int *b;
b = myFunction (a, 5);
cout << *b << endl;
}
Output?
What does the following code do? What is the output?
#include
#include
using namespace std;
struct point {
int x, y;
};
class pointy {
public:
pointy() {
point1.x = 0;
point1.y = 0;
point2.x = 0;
point2.y = 0;
}
pointy(point in_point1, point in_point2) {
point1 = in_point1;
point2 = in_point2;
}
void mystic_print() {
cout << abs(point1.x - point2.x) << " " ;
cout << abs(point1.y - point2.y) << " " ;
cout << abs(point1.x - point2.x)*abs(point1.y - point2.y)
<< endl;
}
private:
point point1;
point point2;
};
int main(){
point x = {3,4};
point y = {5,6};
pointy A1(x, y);
A1.mystic_print();
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
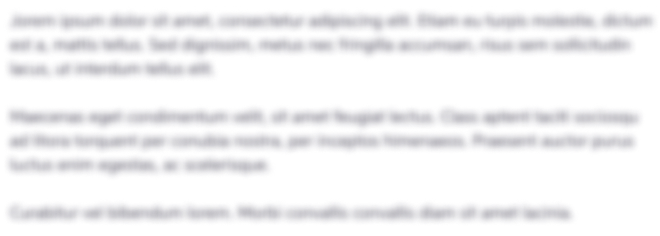
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started