Question
Golf Python code (Assignment repost due to incorrect code!) Below is the code from the previous post which doesn't even works: class GolfScore:
Golf Python code (Assignment repost due to incorrect code!)
Below is the code from the previous post which doesn't even works:
class GolfScore:
def __init__(self, par, strokes):
self.par = par
self.strokes = strokes
def calculate_score(self):
if self.par not in {3, 4, 5}:
return "Error"
score = self.strokes - self.par
if score == -2:
return "Eagle"
elif score == -1:
return "Birdie"
elif score == 0:
return "Par"
elif score == 1:
return "Bogey"
elif score == 2:
return "Double Bogey"
elif score == 3:
return "Triple Bogey"
elif score == 4:
return "Quadruple Bogey"
else:
return "Oh, not good..."
if __name__ == "__main__":
par, strokes = map(int, input("Enter par and strokes: ").split())
golf_score = GolfScore(par, strokes)
result = golf_score.calculate_score()
print(result)
*Note please check and verify your code actually works and send a screenshot of the code, including another screenshot of a successful run of the program using Python IDLE 3.11.6. Either you can correct this code or start fresh with different code that works.
Instructions
The score on a golf hole is the number of strokes (hitting the ball) taken to get the ball in the hole. "Par" is the number of strokes expected for it to take to get the ball into the hole, e.g. 3, 4, or 5.
We describe the situation on a given hole in a player's game by checking the difference between the par and the actual number of strokes the player took:
"Eagle": number of strokes is two less than par
"Birdie": number of strokes is one less than par
"Par": number of strokes equals par
"Bogey": number of strokes is one more than par
"Double Bogey:" number of strokes is two more than par
"Triple Bogey:" number of strokes is three more than par
"Quadruple Bogey:" number of strokes is four more than par
Just output the message "Oh, not good..." if the number of strokes is more than four over par.
Write a program like this:
CONSOLE INPUT: Two integers, the first of which represents par and the second specifying the number of strokes actually used by the player.
CONSOLE OUTPUT:
Appropriate score name. For example if the par was 4 and the strokes was 6, print "Double Bogey"
Print "Error" if par is not 3, 4, or 5.
NOTE: Using what you have learned lately about Class design, you are expected to create a nicely organized custom class when implementing these specifications.
HINTS:
Use branching, i.e. "if-else" or "match" to write this program. Documentation of match statement. https://docs.python.org/3/whatsnew/3.10.html
WHAT TO SUBMIT: Your code in the box below.
Additional resources for assignment (download file via dropbox):
- golfTemplateCode.py https://www.dropbox.com/scl/fi/vjeu6fhrm1fod0n1dormp/golfTemplateCode.py?rlkey=xgi1010bop3y3pbq7u1zdkn5t&dl=0
Step by Step Solution
3.34 Rating (157 Votes )
There are 3 Steps involved in it
Step: 1
Answer It appears there were some issues with the indentation in th...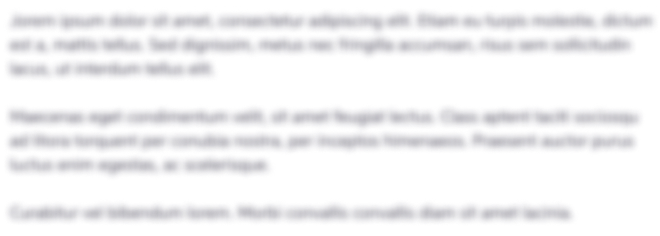
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started