Question
Good morning everyone!!! It was given me as a data structures project the creation of a linked list that performs basic arithmetic operations (Add, subtract,
Good morning everyone!!!
It was given me as a data structures project the creation of a linked list that performs basic arithmetic operations (Add, subtract, multiplication, division, and reminder) with two polynomials. Ive been having some issues to create the methods that perform the operations before mentioned. Ive been trying for almost a week and I couldnt do it. I attached my current java code; I wrote in bold the functions that I have to work on (Last class of the code) and the current output. Any advice, any guide, any help to approach this solution will be welcome.
class DNode
private T data;
private DNode
public DNode(T d, DNode
data = d;
next = n;
prev = p;
}
public T getData() {
return data;
}
public DNode
return next;
}
public DNode
return prev;
}
public void setData(T d) {
data = d;
}
public void setNext(DNode
next = n;
}
public void setPrev(DNode
prev = p;
}
}
class DList
private DNode
private int size;
public DList() {
size = 0;
header = new DNode
trailer = new DNode
header.setNext(trailer);
}
// utility methods
public int size() {
return size;
}
public boolean isEmpty() {
return size == 0;
}
// give clients access to nodes, but not to the header or trailer
public DNode
if (isEmpty())
throw new Exception("Empty");
return header.getNext();
}
public DNode
if (isEmpty())
throw new Exception("Empty");
return trailer.getPrev();
}
public DNode
DNode
if (ans == null || ans == trailer)
throw new Exception("No such node");
return ans;
}
public DNode
DNode
if (ans == null || ans == header)
throw new Exception("No such node");
return ans;
}
// methods to change the list
public void addBefore(T d, DNode
DNode
DNode
u.setNext(x);
v.setPrev(x);
size++;
}
public void addAfter(T d, DNode
DNode
DNode
v.setNext(x);
w.setPrev(x);
size++;
}
public void addFirst(T d) {
addAfter(d, header);
}
public void addLast(T d) {
addBefore(d, trailer);
}
public T remove(DNode
if (v == header || v == trailer)
throw new Exception("Sentinel");
DNode
DNode
w.setPrev(u);
u.setNext(w);
size--;
return v.getData();
}
// LinkedList testing methods:
public String toString() {
String ans = "";
DNode
ans += "(H)<-->";
do {
n = n.getNext();
if (n == trailer)
ans += "(T)";
else
ans += (n.getData() + "<-->");
} while (n != trailer);
return ans;
}
}
class Term {
Double coefficient;
int degree;
public Term() {
this(null, 0);
}
public boolean isPositive() {
return coefficient > 0;
}
public Term(Double coefficient, int degree) {
this.coefficient = coefficient;
this.degree = degree;
}
public Double getCoefficient() {
return coefficient;
}
public void setCoefficient(Double coefficient) {
this.coefficient = coefficient;
}
public int getDegree() {
return degree;
}
public void setDegree(int degree) {
this.degree = degree;
}
public String toString() {
String ans = "";
if (coefficient.doubleValue() == 0) return "";
if (degree == 0) return coefficient.toString();
if (coefficient != 1) {
if (coefficient == -1) ans += "- ";
else ans += coefficient + " ";
}
ans = ans + "X";
if (degree == 1) return ans;
return ans + "^" + degree;
}
}
abstract class Polynomial {
DList
public Polynomial() {
data = new DList<>();
}
public final String toString() {
String ans = "";
boolean starting = true;
try {
DNode
while (n != null) {
if (!starting && n.getData().isPositive()) ans += " +";
starting = false;
ans += " " + n.getData().toString();
n = data.getNext(n);
}
} catch (Exception e) {
if (starting) return "0";
}
return ans;
}
abstract public Polynomial add(Polynomial p);
abstract public Polynomial subtract(Polynomial p);
abstract public Polynomial multiply(Polynomial p);
abstract public Polynomial divide(Polynomial p) throws Exception;
abstract public Polynomial remainder(Polynomial p) throws Exception;
}
class Utility {
public static void run(Polynomial p, Polynomial q) throws Exception {
System.out.println("Polynomials p = " + p + " q = " + q);
System.out.println("Sum " + p.add(q));
System.out.println("Difference " + p.subtract(q));
System.out.println("Product " + p.multiply(q));
System.out.println("Quotient " + p.divide(q));
System.out.println("Remainder " + p.remainder(q));
}
}
public class X00000000 extends Polynomial {
public static void main(String args[]) throws Exception {
Polynomial p = new X00000000(" X^5"),
q = new X00000000("X^2 - X + 1");
Utility.run(p, q);
}
public X00000000(String s) {
//parse string character by character
double coef = 0; //coefficient of term
int deg = 0; //degree of term
String[] terms = s.split(" ");
for(int i = 0; i < terms.length; i++)
{
String term= terms[i];
//System.out.println("term:"+term);
String prevTerm="";
if(i != 0)
prevTerm=terms[i-1];
if(term.startsWith("X"))
{
if(term.length()==3 && term.contains("^"))
{
coef=1;
deg=Integer.parseInt(term.substring(2));
}
else if (term.length() == 1)
{
deg=1;
if(prevTerm.equals("-"))
coef=-1;
else
coef=1;
}
}
else if(term.startsWith("-") || term.startsWith("+"))
continue;
else if(term.length()==1 && Character.isDigit(term.charAt(0)))
{
coef=Integer.parseInt(term);
if(prevTerm.equals("-"))
coef*=-1;
deg=0;
}
Term T = new Term(coef, deg);
if(data.isEmpty())
{
data.addFirst(T);
}
else
{
data.addLast(T);
}
}
}
public X00000000() {
super();}
public Polynomial add(Polynomial p) {
Polynomial ans = new X00000000();
// complete this code
return ans;
}
public Polynomial subtract(Polynomial p) {
Polynomial ans = new X00000000();
// complete this code
return ans;
}
public Polynomial multiply(Polynomial p) {
Polynomial ans = new X00000000();
// complete this code
return ans;
}
public Polynomial divide(Polynomial p) throws Exception {
Polynomial ans = new X00000000();
// complete this code
return ans;
}
public Polynomial remainder(Polynomial p) throws Exception {
Polynomial ans = new X00000000();
// complete this code
return ans;
}
}
Actual Output:
Polynomials p = + X^5 q = X^2 - X + 1.0 Sum 0 Difference 0 Product 0 Quotient 0 Remainder 0
Step by Step Solution
There are 3 Steps involved in it
Step: 1
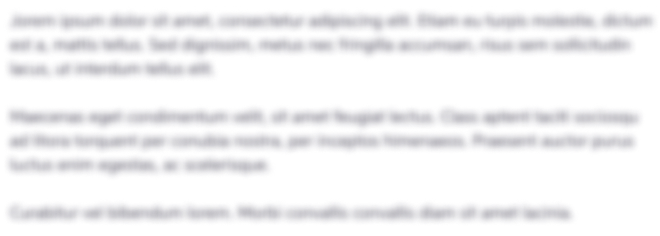
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started