Question
got the code for 1, 2, and 3 but need to put them all onto one menu can anyon help Create the an application that
got the code for 1, 2, and 3 but need to put them all onto one menu can anyon help
Create the an application that can use different types of Linked List to manage the Candidate Records of a company. The application should allow users can select the type of Linked List to work on. After finishing one, users can select to work with other type of Linked List until they want to exit
1. Singly Linked List
2. Singly Linked List with Iterator
3. Java Linked List with Iterator
singly linked list-------------------------------------------------------------------------------------------
import java.io.*;
import java.util.Scanner;
class linkedlist {
int CandidateId;
int salary;
String name;
linkedlist next;
linkedlist(int value,int salary,String name) {
this.CandidateId = value;
this.salary=salary;
this.name=name;
}
void display() {
System.out.println(CandidateId+"\t"+salary+"\t"+name);
}
}
class linked {
public linkedlist fstnode, lastnode;
linked() {
fstnode = null;
lastnode = null;
}
/* Insert node or create linked list */
void insertnode(int value,int salary,String name) {
linkedlist node = new linkedlist(value,salary,name);
node.next = null;
if(fstnode == null) {
fstnode = lastnode = node;
System.out.println("Linked list created successfully!");
}
else {
lastnode.next = node;
lastnode = node;
System.out.println("Node inserted successfully!");
}
}
/* Delete node from linked list */
void delete() {
int count = 0, number, i;
linkedlist node, node1;
Scanner input = new Scanner(System.in);
for(node = fstnode; node != null; node = node.next)
count++;
display();
node = node1 = fstnode;
System.out.println(count+" nodes available here!");
System.out.println("Enter the node number which you want to delete:");
number = Integer.parseInt(input.nextLine());
if(number != 1) {
if(number <= count) {
for(i = 2; i <= number; i++)
node = node.next;
for(i = 2; i <= number-1; i++)
node1 = node1.next;
node1.next = node.next;
node.next = null;
node = null;
}
else
System.out.println("Invalid node number! ");
}
else {
fstnode = node.next;
node = null;
}
System.out.println("Node has been deleted successfully! ");
}
/* Display linked list */
void display() {
linkedlist node = fstnode;
System.out.println("List of node:");
while(node != null) {
node.display();
node = node.next;
}
}
}
class Link {
public static void main(String args[ ]) {
linked list = new linked();
Scanner input = new Scanner(System.in);
int op = 0;
while(op != 4) {
System.out.println("1. Insert 2. Delete 3. Show All 4. Exit");
System.out.println("Enter your choice:");
op = Integer.parseInt(input.nextLine());
switch(op) {
case 1:
System.out.print("Enter the candidate id :");
int id=Integer.parseInt(input.nextLine());
System.out.print("Enter the candidate salary :");
int salary=Integer.parseInt(input.nextLine());
System.out.print("Enter the candidate name :");
String name=input.nextLine();
list.insertnode(id,salary,name);
break;
case 2:
list.delete();
break;
case 3:
list.display();
break;
case 4:
System.out.println("Bye Bye!");
System.exit(0);
break;
default:
System.out.println("Invalid choice!");
}
}
}
}
singly linked list with iterator--------------------------------------------------------------------------
package com.samples; import java.util.Scanner; class Test { String Title; Test ref=null; public static Test refval=null; public static void add(String val) { if(refval==null) { Test Temp=new Test(); Temp.Title=val; refval=Temp; Temp.ref=null; } else { Test tem=refval; while(tem.ref!=null) { tem=tem.ref; } Test obj=new Test(); obj.Title=val; tem.ref=obj; obj.ref=null; } } public static void Show() { Test tem=refval; if(tem==null) { System.out.println("No Data to view"); } else while(tem!=null) { System.out.println(tem.Title); tem=tem.ref; } } public static void addFirst(String val) { if(refval==null) { Test Temp=new Test(); Temp.Title=val; refval=Temp; Temp.ref=null; } else { Test temp=new Test(); temp.Title=val; temp.ref=refval; refval=temp; } } public static void deleteFirstNode() { if(refval==null) { System.out.println("No elemet to delete. add an element then delete"); } else { int heapSize = (int) Runtime.getRuntime().totalMemory(); System.out.println("Memory before deleting "+ heapSize); refval=refval.ref; System.out.println("memory after deleting"+Runtime.getRuntime()); } } } public class LinkedList { public static void main(String arvg[]) { Scanner sc=new Scanner(System.in); String title = null; System.out.println(" Read Candidate Data:press stop to stop the input"); do { title=sc.next(); Test.add(title); }while(!(title.equals("stop"))); int option; while(true) { System.out.println("Enter 1 to add data to link List "); System.out.println("Enter 2 to view Data "); System.out.println("enter 3 to Update title "); System.out.println("enter 4 to delete firstval"); System.out.println("enter 5 to exit"); option=sc.nextInt(); switch(option) { case 1: System.out.println("Enter val to insert into the list: "); title=sc.next(); Test.add(title); break; case 2: Test.Show(); break; case 3: System.out.println("Enter value "); title=sc.next(); Test.addFirst(title); case 4: Test.deleteFirstNode(); break; case 5: System.exit(0); default: System.out.println("Wrong User choice going to end"); System.exit(0); } } } }
java linked list with iterator------------------------------------------------------------------------------------
import java.util.LinkedList;
import java.util.Iterator;
import java.util.Scanner;
class LinkedListIterator
{
static LinkedList
static LinkedList
static Scanner scan=new Scanner(System.in);
public static void main(String a[])
{
int option,choice,k;
System.out.println("-------LinkedListIterator------ ");
do
{
System.out.println("Please select any one Option \t1.Insert\t2.Fetch\t3.Update\t4.Delete:\t");
choice=scan.nextInt();
switch(choice)
{
case 1:
System.out.println("Do you want to insert LastName for whome? \t1.Candidate\t2.ExperienceCandidate:\t");
k=scan.nextInt();
insert(k);
break;
case 2:
System.out.println("Do you want to Fetch Data of whome? \t1.Candidate\t2.ExperienceCandidate:\t");
k=scan.nextInt();
fetch(k);
break;
case 3:
System.out.println("Do you want to Update Data of whome? \t1.Candidate\t2.ExperienceCandidate:\t");
k=scan.nextInt();
update(k);
break;
case 4:
System.out.println("Do you want to DELETE Data of whome? \t1.Candidate\t2.ExperienceCandidate:\t");
k=scan.nextInt();
delete(k);
break;
default:
System.out.println("Enter Correct Option...");
break;
}
System.out.println("Do you want to Continue? Press 1. for Yes\t 2. for No:\t");
option=scan.nextInt();
}while(option!=2);
/* candidate.add("Dravid");
candidate.add("Dhoni");
candidate.add("Sachin");
candidate.add("Sastry");
ExperienceCandidate.add("Kapil");
ExperienceCandidate.add("Kumble");
ExperienceCandidate.add("Siddu");
ExperienceCandidate.add("Viswa");
Iterator it=candidate.iterator();
while(it.hasNext())
{
System.out.println(it.next());
}*/
}
public static void insert(int k)
{
if(k==1)
{
System.out.println("Enter Candidate LastName: ");
String lname=scan.next();
candidate.add(lname);
}
if(k==2)
{
System.out.println("Enter Candidate LastName: ");
String lname=scan.next();
ExperienceCandidate.add(lname);
}
}
public static void fetch(int k)
{
if(k==1)
{
Iterator it=candidate.iterator();
while(it.hasNext())
{
System.out.println(it.next());
}
}
if(k==2)
{
Iterator it=candidate.iterator();
while(it.hasNext())
{
System.out.println(it.next());
}
}
}
public static void update(int k)
{
if(k==1)
{
System.out.println("Enter Position of Candidate for Updation:\t");
int z=scan.nextInt();
System.out.println("Enter Candidate LastName: ");
String lname=scan.next();
candidate.set(z,lname);
}
if(k==2)
{
System.out.println("Enter Position of Candidate for Updation:\t");
int z=scan.nextInt();
System.out.println("Enter Candidate LastName: ");
String lname=scan.next();
ExperienceCandidate.set(z,lname);
}
}
public static void delete(int k)
{
if(k==1)
{
candidate.removeFirst();
}
if(k==2)
{
ExperienceCandidate.removeFirst();
}
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
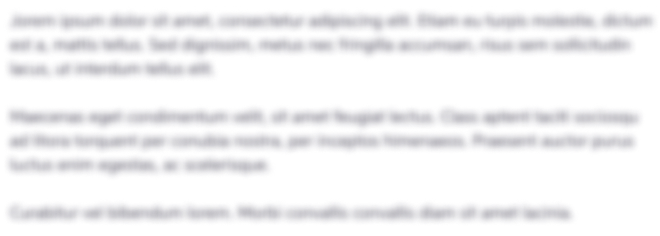
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started