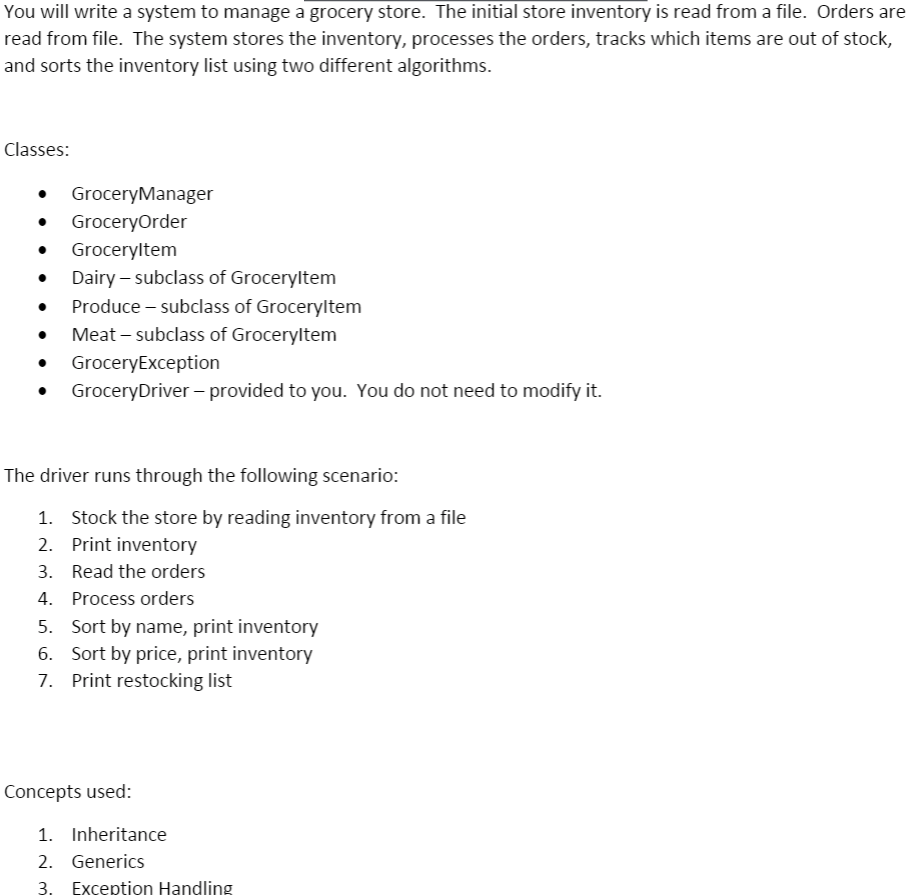
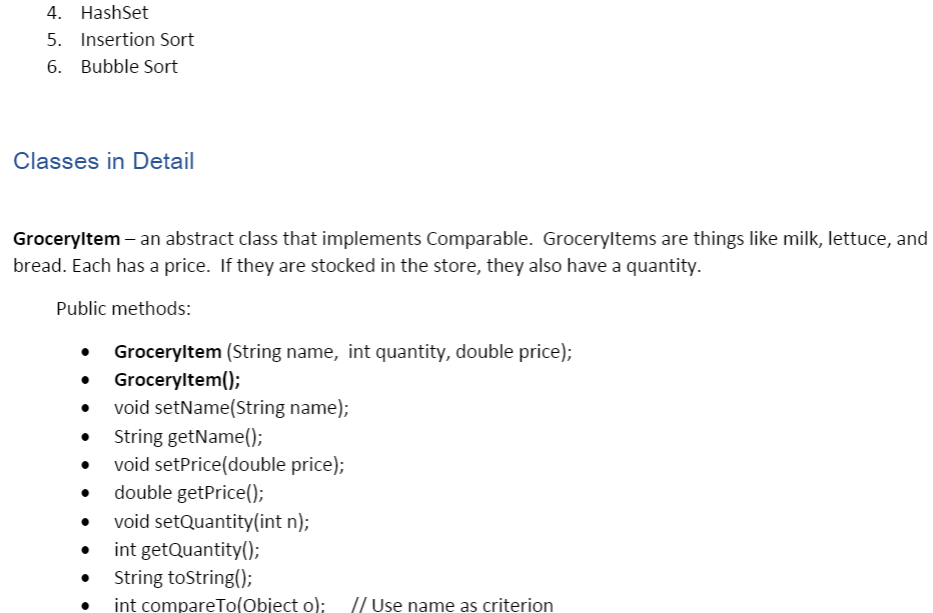
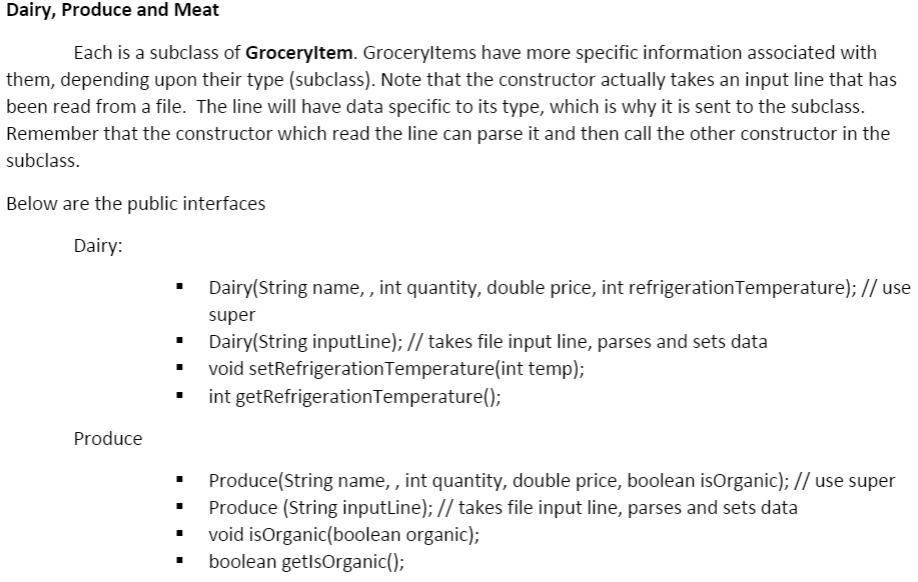

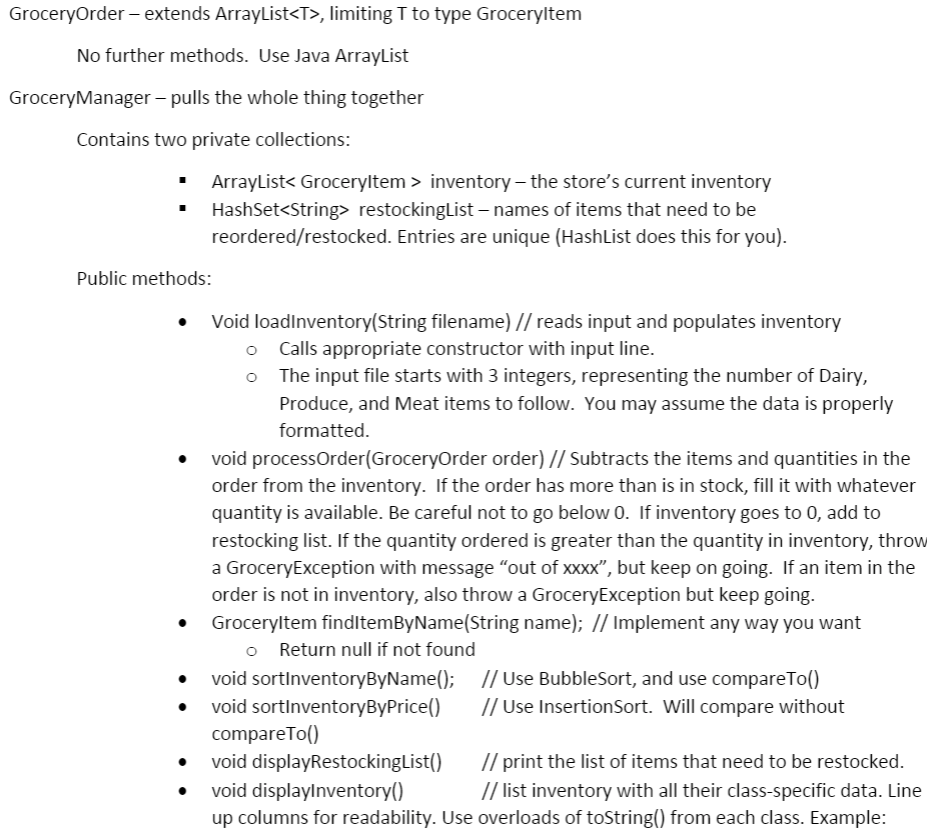
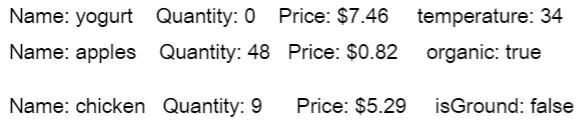
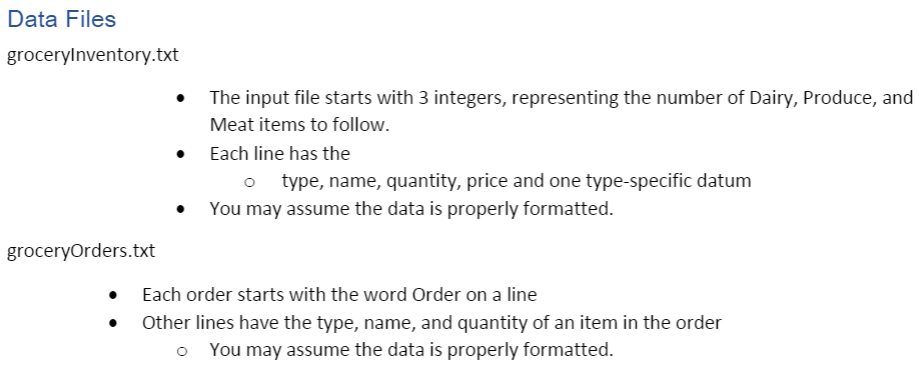
GroceryDriver.java
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.util.ArrayList;
import java.util.Scanner;
/** Driver for Grocery Manager: Read files, process orders, sort and print results*/
public class GroceryDriver {
static ArrayList> orders = new ArrayList();
public static void main(String[] args) {
GroceryManager manager = new GroceryManager();
// stock storemanager.loadInventory("groceryInventory.txt");
System.out.println("******** Initial Inventory ********");
manager.displayInventory();
// purchase itemsSystem.out.println(" ******** Processing Orders ********");
readOrders();
for (GroceryOrder order : orders) {
try {manager.processOrder(order);
} catch (GroceryException e) {
System.out.println(e.getMessage());} }
manager.displayInventory();
// sort inventorymanager.sortInventoryByName();
System.out.println(" ******** Sort by name ********");
manager.displayInventory();
manager.sortInventoryByPrice();
System.out.println(" ******** Sort by price ********");
manager.displayInventory();
System.out.println(" ******** Restocking List ********");
manager.displayReorders();}
public static void readOrders() {
Scanner input = null;
String line;
String[] parts;
try {input = new Scanner(new FileInputStream("groceryOrders.txt"));
while (input.hasNext()) {
GroceryOrder list = new GroceryOrder();
input.nextLine();// ORDER
line = input.nextLine();
parts = line.split(" ");
list.add(new Dairy(parts[1], Integer.parseInt(parts[2]), 0,0));
line = input.nextLine();
parts = line.split(" ");
list.add(new Produce(parts[1], Integer.parseInt(parts[2]), 0, false));
line = input.nextLine();
parts = line.split(" ");
list.add(new Meat(parts[1], Integer.parseInt(parts[2]), 0, false));
orders.add(list);}
} catch (Exception e) {System.out.println(e);
} finally {input.close(); }}}
Grocery Inventory txt
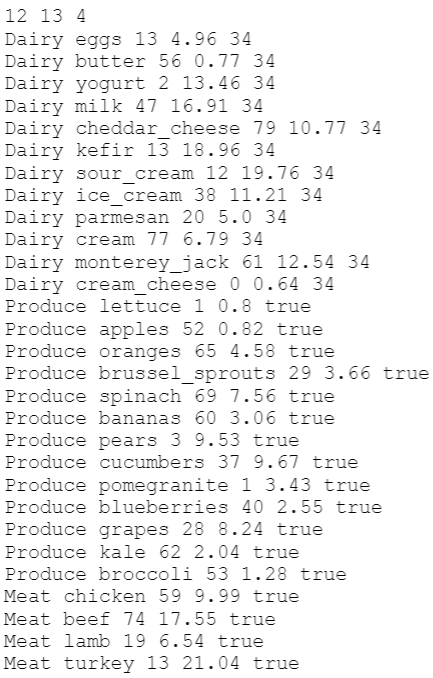
Grocery Orders txt
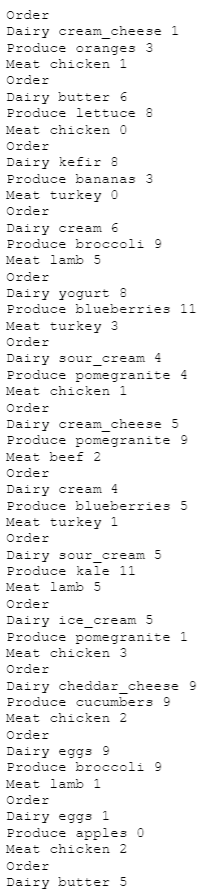
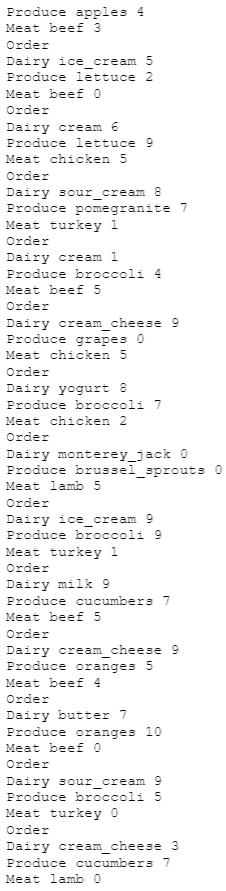
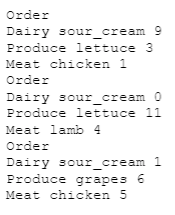
Grocery Sample Output Doc
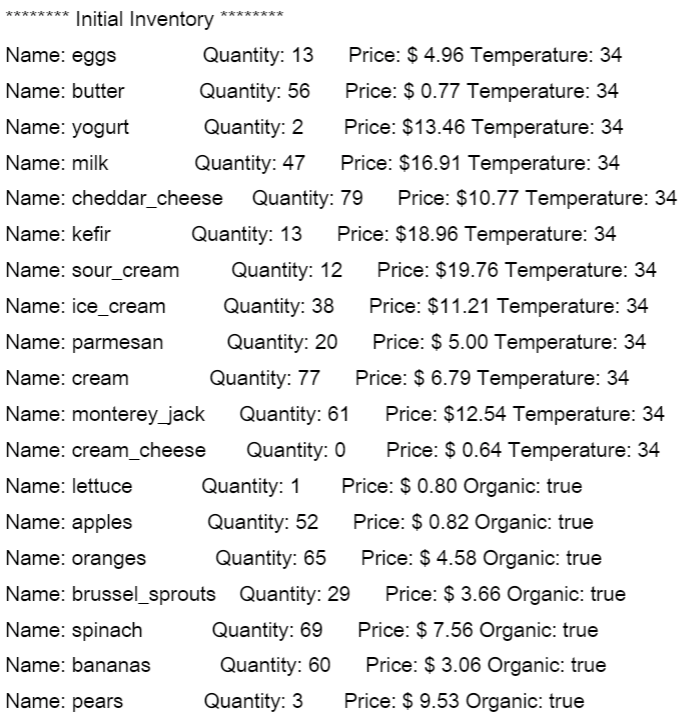
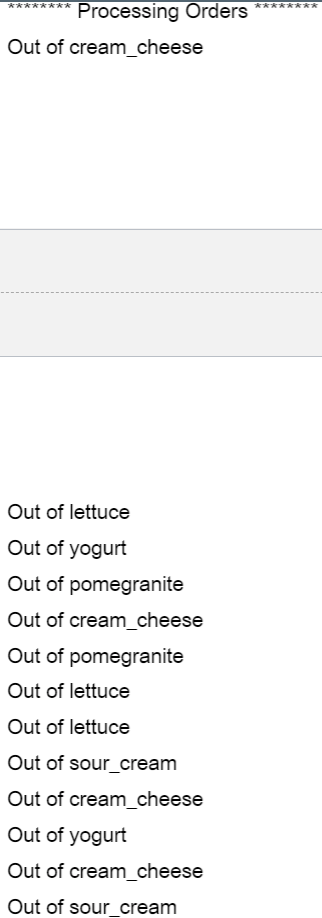
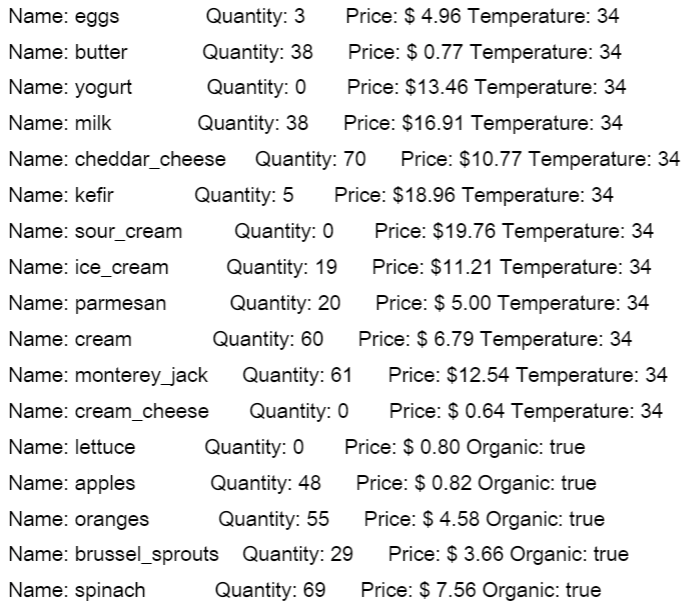
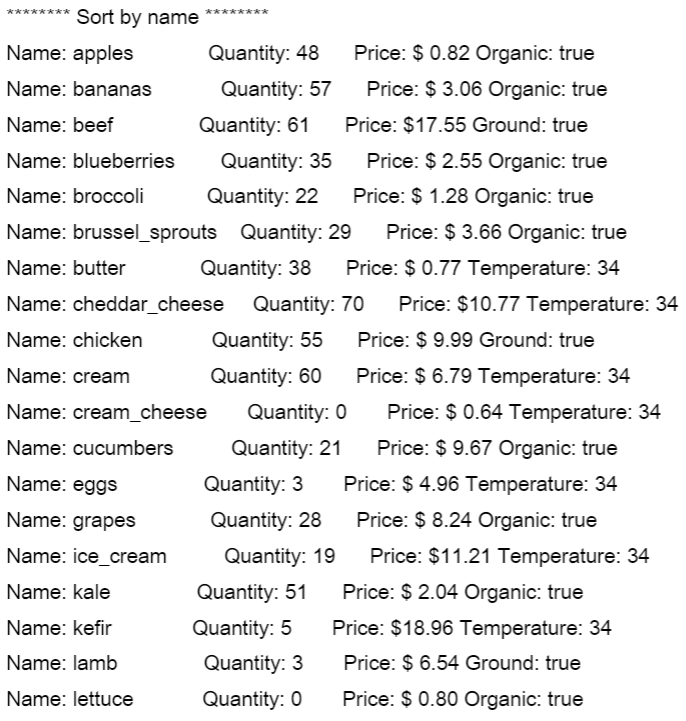
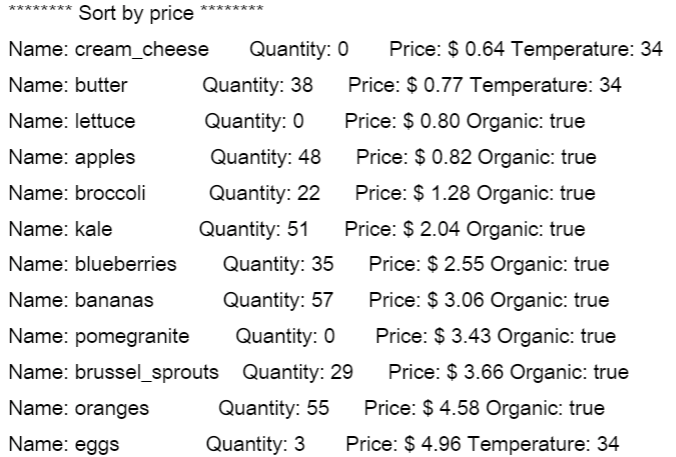
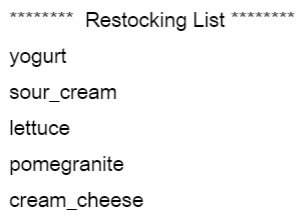
You will write a system to manage a grocery store. The initial store inventory is read from a file. Orders are read from file. The system stores the inventory, processes the orders, tracks which items are out of stock, and sorts the inventory list using two different algorithms. Classes: . . GroceryManager GroceryOrder Groceryltem Dairy - subclass of Groceryltem Produce - subclass of Groceryltem Meat-subclass of Groceryltem GroceryException GroceryDriver provided to you. You do not need to modify it. . The driver runs through the following scenario: 1. Stock the store by reading inventory from a file 2. Print inventory 3. Read the orders 4. Process orders 5. Sort by name, print inventory 6. Sort by price, print inventory 7. Print restocking list Concepts used: 1. Inheritance 2. Generics 3. Exception Handling 4. HashSet 5. Insertion Sort 6. Bubble Sort Classes in Detail Groceryltem- an abstract class that implements Comparable. Groceryltems are things like milk, lettuce, and bread. Each has a price. If they are stocked in the store, they also have a quantity. Public methods: Groceryltem (String name, int quantity, double price); Groceryltem(); void setName(String name); String getName(); void setPrice(double price); double getPrice(); void setQuantity(int n); int getQuantity(); String toString(); int compareTo(Object o); // Use name as criterion Dairy, Produce and Meat Each is a subclass of Groceryltem. Groceryltems have more specific information associated with them, depending upon their type (subclass). Note that the constructor actually takes an input line that has been read from a file. The line will have data specific to its type, which is why it is sent to the subclass. Remember that the constructor which read the line can parse it and then call the other constructor in the subclass. Below are the public interfaces Dairy: Dairy(String name,, int quantity, double price, int refrigeration Temperature); // use super Dairy(String inputline); // takes file input line, parses and sets data void setRefrigeration Temperature(int temp); int getRefrigeration Temperature(); Produce Produce(String name,, int quantity, double price, boolean isOrganic); // use super Produce (String inputline); // takes file input line, parses and sets data void isOrganic(boolean organic); boolean getisOrganic(); Meat 1 Meat(String name, int quantity, double price, boolean isGround); // use super Meat(String inputline); // takes file input line, parses and sets data void isGround(boolean ground); boolean getIsGround(); GroceryOrder - extends ArrayList
, limiting T to type Groceryltem No further methods. Use Java ArrayList Grocery Manager - pulls the whole thing together Contains two private collections: ArrayList inventory the store's current inventory HashSet restockingList - names of items that need to be reordered/restocked. Entries are unique (HashList does this for you). Public methods: . Void loadinventory(String filename) // reads input and populates inventory Calls appropriate constructor with input line. o The input file starts with 3 integers, representing the number of Dairy, Produce, and Meat items to follow. You may assume the data is properly formatted. void processOrder(GroceryOrder order) // Subtracts the items and quantities in the order from the inventory. If the order has more than is in stock, fill it with whatever quantity is available. Be careful not to go below 0. If inventory goes to 0, add to restocking list. If the quantity ordered is greater than the quantity in inventory, throw a GroceryException with message "out of xxxx, but keep on going. If an item in the order is not in inventory, also throw a GroceryException but keep going. Groceryltem finditemByName(String name); // Implement any way you want o Return null if not found void sortinventoryByName(); // Use BubbleSort, and use compareTo() void sortInventoryByPrice() // Use InsertionSort. Will compare without compareTo() void displayRestockingList() // print the list of items that need to be restocked. void displayinventory() // list inventory with all their class-specific data. Line up columns for readability. Use overloads of toString() from each class. Example: Name: yogurt Quantity: 0 Price: $7.46 temperature: 34 Name: apples Quantity: 48 Price: $0.82 organic: true Name: chicken Quantity: 9 Price: $5.29 isGround: false Data Files grocerylnventory.txt The input file starts with 3 integers, representing the number of Dairy, Produce, and Meat items to follow. Each line has the type, name, quantity, price and one type-specific datum You may assume the data is properly formatted. groceryOrders.txt Each order starts with the word Order on a line Other lines have the type, name, and quantity of an item in the order 0 You may assume the data is properly formatted. 12 13 4 Dairy eggs 13 4.96 34 Dairy butter 56 0.77 34 Dairy yogurt 2 13.46 34 Dairy milk 47 16.91 34 Dairy cheddar cheese 79 10.77 34 Dairy kefir 13 18.96 34 Dairy sour_cream 12 19.76 34 Dairy ice cream 38 11.21 34 Dairy parmesan 20 5.0 34 Dairy cream 77 6.79 34 Dairy monterey_jack 61 12.54 34 Dairy cream cheese 0 0.64 34 Produce lettuce 1 0.8 true Produce apples 52 0.82 true Produce oranges 65 4.58 true Produce brussel_sprouts 29 3.66 true Produce spinach 69 7.56 true Produce bananas 60 3.06 true Produce pears 3 9.53 true Produce cucumbers 37 9.67 true Produce pomegranite 1 3.43 true Produce blueberries 40 2.55 true Produce grapes 28 8.24 true Produce kale 62 2.04 true Produce broccoli 53 1.28 true Meat chicken 59 9.99 true Meat beef 74 17.55 true Meat lamb 19 6.54 true Meat turkey 13 21.04 true Order Dairy cream cheese 1 Produce oranges 3 Meat chicken 1 Order Dairy butter 6 Produce lettuce 8 Meat chicken 0 Order Dairy kefir 8 Produce bananas 3 Meat turkey 0 Order Dairy cream 6 Produce broccoli 9 Meat lamb 5 Order Dairy yogurt 8 Produce blueberries 11 Meat turkey 3 Order Dairy sour_cream 4 Produce pomegranite 4 Meat chicken 1 Order Dairy cream cheese 5 Produce pomegranite 9 Meat beef 2 Order Dairy cream 4 Produce blueberries 5 Meat turkey 1 Order Dairy sour_cream 5 Produce kale 11 Meat lamb 5 Order Dairy ice cream 5 Produce pomegranite 1 Meat chicken 3 Order Dairy cheddar cheese 9 Produce cucumbers 9 Meat chicken 2 Order Dairy eggs 9 Produce broccoli 9 Meat lamb 1 Order Dairy eggs 1 Produce apples 0 Meat chicken 2 Order Dairy butter 5 Produce apples 4 Meat beef 3 Order Dairy ice cream 5 Produce lettuce 2 Meat beef 0 Order Dairy cream 6 Produce lettuce 9 Meat chicken 5 Order Dairy sour_cream 8 Produce pomegranite 7 Meat turkey 1 Order Dairy cream 1 Produce broccoli 4 Meat beef 5 Order Dairy cream cheese 9 Produce grapes 0 Meat chicken 5 Order Dairy yogurt 8 Produce broccoli 7 Meat chicken 2 Order Dairy monterey_jack 0 Produce brussel_sprouts o Meat lamb 5 Order Dairy ice cream 9 Produce broccoli 9 Meat turkey 1 Order Dairy milk 9 Produce cucumbers 7 Meat beef 5 Order Dairy cream cheese 9 Produce oranges 5 Meat beef 4 Order Dairy butter 7 Produce oranges 10 Meat beef 0 Order Dairy sour_cream 9 Produce broccoli 5 Meat turkey 0 Order Dairy cream cheese 3 Produce cucumbers 7 Meat lamb 0 Order Dairy sour_cream 9 Produce lettuce 3 Meat chicken 1 Order Dairy sour_cream 0 Produce lettuce 11 Meat lamb 4 Order Dairy sour cream 1 Produce grapes 6 Meat chicken 5 Initial Inventory Name: eggs Quantity: 13 Price: $ 4.96 Temperature: 34 Name: butter Quantity: 56 Price: $ 0.77 Temperature: 34 Name: yogurt Quantity: 2 Price: $13.46 Temperature: 34 Name: milk Quantity: 47 Price: $16.91 Temperature: 34 Name: cheddar_cheese Quantity: 79 Price: $10.77 Temperature: 34 Name: kefir Quantity: 13 Price: $18.96 Temperature: 34 Name: sour_cream Quantity: 12 Price: $19.76 Temperature: 34 Name: ice_cream Quantity: 38 Price: $11.21 Temperature: 34 Name: parmesan Quantity: 20 Price: $ 5.00 Temperature: 34 Name: cream Quantity: 77 Price: $ 6.79 Temperature: 34 Name: monterey_jack Quantity: 61 Price: $12.54 Temperature: 34 Name: cream cheese Quantity: 0 Price: $ 0.64 Temperature: 34 Name: lettuce Quantity: 1 Price: $ 0.80 Organic: true Name: apples Quantity: 52 Price: $ 0.82 Organic: true Name: oranges Quantity: 65 Price: $ 4.58 Organic: true Name: brussel_sprouts Quantity: 29 Price: $ 3.66 Organic: true Name: spinach Quantity: 69 Price: $ 7.56 Organic: true Name: bananas Quantity: 60 Price: $ 3.06 Organic: true Name: pears Quantity: 3 Price: $ 9.53 Organic: true Processing Orders Out of cream cheese Out of lettuce Out of yogurt Out of pomegranite Out of cream cheese Out of pomegranite Out of lettuce Out of lettuce Out of sour_cream Out of cream cheese Out of yogurt Out of cream_cheese Out of sour_cream Name: eggs Quantity: 3 Price: $ 4.96 Temperature: 34 Name: butter Quantity: 38 Price: $ 0.77 Temperature: 34 Name: yogurt Quantity: 0 Price: $13.46 Temperature: 34 Name: milk Quantity: 38 Price: $16.91 Temperature: 34 Name: cheddar_cheese Quantity: 70 Price: $10.77 Temperature: 34 Name: kefir Quantity: 5 Price: $18.96 Temperature: 34 Name: sour_cream Quantity: 0 Price: $19.76 Temperature: 34 Name: ice_cream Quantity: 19 Price: $11.21 Temperature: 34 Name: parmesan Quantity: 20 Price: $ 5.00 Temperature: 34 Name: cream Quantity: 60 Price: $ 6.79 Temperature: 34 Name: monterey_jack Quantity: 61 Price: $12.54 Temperature: 34 Name: cream_cheese Quantity: 0 Price: $ 0.64 Temperature: 34 Name: lettuce Quantity: 0 Price: $ 0.80 Organic: true Name: apples Quantity: 48 Price: $ 0.82 Organic: true Name: oranges Quantity: 55 Price: $ 4.58 Organic: true Name: brussel_sprouts Quantity: 29 Price: $ 3.66 Organic: true Name: spinach Quantity: 69 Price: $ 7.56 Organic: true Sort by name Name: apples Quantity: 48 Price: $ 0.82 Organic: true Name: bananas Quantity: 57 Price: $ 3.06 Organic: true Name: beef Quantity: 61 Price: $17.55 Ground: true Name: blueberries Quantity: 35 Price: $ 2.55 Organic: true Name: broccoli Quantity: 22 Price: $ 1.28 Organic: true Name: brussel_sprouts Quantity: 29 Price: $ 3.66 Organic: true Name: butter Quantity: 38 Price: $ 0.77 Temperature: 34 Name: cheddar cheese Quantity: 70 Price: $10.77 Temperature: 34 Name: chicken Quantity: 55 Price: $ 9.99 Ground: true Name: cream Quantity: 60 Price: $ 6.79 Temperature: 34 Name: cream cheese Quantity: 0 Price: $ 0.64 Temperature: 34 Name: cucumbers Quantity: 21 Price: $ 9.67 Organic: true Name: eggs Quantity: 3 Price: $ 4.96 Temperature: 34 Name: grapes Quantity: 28 Price: $ 8.24 Organic: true Name: ice_cream Quantity: 19 Price: $11.21 Temperature: 34 Name: kale Quantity: 51 Price: $ 2.04 Organic: true Name: kefir Quantity: 5 Price: $18.96 Temperature: 34 Name: lamb Quantity: 3 Price: $ 6.54 Ground: true Name: lettuce Quantity: 0 Price: $ 0.80 Organic: true ***** ******** Sort by price Name: cream cheese Quantity: 0 Price: $ 0.64 Temperature: 34 Name: butter Quantity: 38 Price: $ 0.77 Temperature: 34 Name: lettuce Quantity: 0 Price: $ 0.80 Organic: true Name: apples Quantity: 48 Price: $ 0.82 Organic: true Name: broccoli Quantity: 22 Price: $ 1.28 Organic: true Name: kale Quantity: 51 Price: $ 2.04 Organic: true Name: blueberries Quantity: 35 Price: $ 2.55 Organic: true Name: bananas Quantity: 57 Price: $ 3.06 Organic: true Name: pomegranite Quantity: 0 Price: $ 3.43 Organic: true Name: brussel_sprouts Quantity: 29 Price: $ 3.66 Organic: true Name: oranges Quantity: 55 Price: $ 4.58 Organic: true Name: eggs Quantity: 3 Price: $ 4.96 Temperature: 34 ******** ******** Restocking List yogurt sour_cream lettuce pomegranite cream_cheese