Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Gui program help: you are required, but not limited, to turn in the following source files: Assignment6.java (The Assignment6 class extends JApplet) Project.java (A modified
Gui program help:
you are required, but not limited, to turn in the following source files:
Assignment6.java (The Assignment6 class extends JApplet)
Project.java (A modified version from the assignment 4)
CreatePanel.java - to be completed (it extends JPanel and contains ButtonListener nested class)
SelectPanel.java - to be completed (it extends JPanel and contains ButtonListener nested class)
Swing/AWT, Vector (very similar to ArrayList class)
Classes may be needed:
JApplet, JButton, JTextField, JTextArea, JLabel, Container, JPanel, JTabbedPane, JList, and ActionListener. You may use other classes.
JApplet defined in javax.swing Project JPanel defined in javax.swin Assignment6 tPane:JTabbedPane createPanel:CreatePanel -selectPanel:SelectPanel projectList.Vector CreatePanel SelectPanel projectList:Vector +selectPanel(Vector) +updateProjectist projectList:Vector -sPanel.selectPanel tinitOvoid CreatePanel(Vector,SelectPanel) ButtonListener ButtonListener tactionPerformed(ActionEvent):void actionPerformed(ActionEvent):void
---------------------------------------------
provided code in Assignment6.java
import javax.swing.*;
import java.util.*;
public class Assignment6 extends JApplet
{
private int APPLET_WIDTH = 800, APPLET_HEIGHT = 300;
private JTabbedPane tPane;
private CreatePanel createPanel;
private SelectPanel selectPanel;
private Vector courseList;
//The method init initializes the Applet with a Pane with two tabs
public void init()
{
//list of courses to be used in every panel
courseList = new Vector();
//register panel uses the list of courses
selectPanel = new SelectPanel(courseList);
createPanel = new CreatePanel(courseList, selectPanel);
//create a tabbed pane with two tabs
tPane = new JTabbedPane();
tPane.addTab("Project creation", createPanel);
tPane.addTab("Project selection", selectPanel);
getContentPane().add(tPane);
setSize (APPLET_WIDTH, APPLET_HEIGHT); //set Applet size
}
}
-------------------------------------------
provided code in CreatePanel.java
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
import java.util.*;
public class CreatePanel extends JPanel
{
private Vector projectList;
private JTextField titleT, projNumT, projLocationT;
private JLabel titleL, projNumL, projLocationL;
private JPanel inputPanel;
private JButton button1;
private JPanel labels, buttonP;
private JLabel message;
private JTextArea area;
private JScrollPane scrollPane;
private SelectPanel sPanel;
//Constructor initializes components and organize them using certain layouts
public CreatePanel(Vector projectList, SelectPanel sPanel)
{
this.projectList = projectList;
this.sPanel = sPanel;
// orgranize components here
// here is an example
button1 = new JButton("Create a project");
setLayout(new GridLayout(1,1));
add(button1);
}
//ButtonListener is a listener class that listens to
//see if the buttont "Create a project" is pushed.
//When the event occurs, it add the project information
//in the text fields to the text area
//and the list of project information,
//and it also does error checking.
private class ButtonListener implements ActionListener
{
public void actionPerformed(ActionEvent event)
{
// if there is no error, add a project to project list
// otherwise, show an error message
} //end of actionPerformed method
} //end of ButtonListener class
} //end of CreatePanel class
--------------------------------------------------------------------
provided code in SelectPanel.java
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
import javax.swing.event.*;
import java.util.*;
public class SelectPanel extends JPanel
{
private Vector projectList, selectedList;
//Constructor initialize each component and arrange them using
//certain layouts
public SelectPanel(Vector projectList)
{
this.projectList = projectList;
// organize components for the select panel
}
//This method updates refresh the JList of projects with
//updated vector information
public void updateProjectList()
{
// call updateUI() for the JList
}
//ButtonListener class listens to see if any of
//buttons is pushed, and perform their corresponding action.
private class ButtonListener implements ActionListener
{
public void actionPerformed(ActionEvent event)
{
//TO BE COMPLETED
}
} //end of ButtonListener class
} //end of SelectPanel class
-----------------------------------------------
provided code in Project.java
import java.util.*;
import java.text.*;
import java.io.*;
public class Project {
//instantiate variable
private String projTitle;
private int projNumber;
private String projLocation;
Manager projManager = new Manager();
public Project()
{
projTitle = "?";
projNumber = 0;
projLocation = "?";
}
// Getter methods for Project class
public String getProjTitle()
{
return projTitle;
}
public int getProjNumber()
{
return projNumber;
}
public String getProjLocation()
{
return projLocation;
}
public Manager getProjManager()
{
return projManager;
}
// Setter methods for Project class
public void setProjTitle(String title)
{
projTitle = title;
}
public void setProjNumber(int number)
{
projNumber = number;
}
public void setProjLocation(String location)
{
projLocation = location;
}
public void setProjManager(String newFname, String newLname, int newDum)
{
projManager.setFirstName(newFname);
projManager.setLastName(newLname);
projManager.setDeptNum(newDum);
}
public String toString()
{
String ProString = " Project Title:\t\t" +getProjTitle()+" Project Number:\t\t" +getProjNumber()+ " Project Location:\t" +getProjLocation()+ " Project Manager:\t" + getProjManager()+" " ;
return ProString ;
}
}
------------------------------------------------------
Write a Java program that constructs an Applet. Your program should provide labels and textfields to a user to enter information regarding projects.
The Applet (JApplet) of your program should contain two tabs. The first tab is labeled "Project creation" and the second tab is labeled "Project selection".
(The size of the applet here is approximately 800 X 300).
The section under the first tab should be divided into two parts:
The left part contains labels, textfields, and a button for a user to enter a project information. The right part shows "No Project" at the beginning (it is done using JTextArea).
A user can enter the information of a project, and push "Create a project" button.
Then the project information should appear on the right hand side panel (note that the format of the project information can be using toString() method of the Project class). A message "Project added" should also appear with red color at the top of the panel.
Error handling:
1. If a user forgets to enter some field and pushes "Create a project" button, show a message "Please enter all fields" with red color, and nothing should be added to the right hand side panel.
2. If a user enters non integer in the field for Project number, and pushes "Create a project" button, show a message "Please enter an integer for the project number." with red color and nothing should be added to the right hand side panel.
After entering several projects, the applet will have the following appearance. Note that a scroll bar appearing when the content of the textarea does not fit within its size. (you will need to use JScrollPane for this.)
Under the "Project selection" tab, a user can select from those created projects. The list of created projects should be made using JList. Below the JList, there should be two buttons, one to " Add" and one to " Remove". Below that, there should be another JList that is initially empty.
The list of projects under the Project selection tab should be exactly same as the list under "Project creation" tab.
A user can choose one project from the to JList, by clicking on it.
Then a user can push the button Add. The selected project should appear in the bottom JList.
A user can select more projects to add.
There is a label below the bottom JList that keeps track of how any projects have been selected
A user can also select a project from the JList below to delete, by clicking it, then by pushing the Remove button.
The label below the bottom JList shows the reduced count of selected projects.
A user should be able to go back and forth between "Project creation" tab and "Project selection" tab, and these two panels need to have consistent list of projects.
Class description
SelectPanel
SelectPanel class extends JPanel defined in javax.swing package. It should contain at least the following instance variable:
This class should have a constructor:
public SelectPanel(Vector projectList)
where the parameter "projectList" is passed from the Assignment6 class. The constructor layouts and organizes components in this panel. You will be adding more variables (components) than what is listed here, including combo boxes, textfields, labels, and a button. The top JList should be instantiated using the "projectList" vector. Then whenever the vector is updated, the JList will be updated as well. They will utilize the toString( ) method of the Project class to display each item.
public void updateProjectList()
This method calls updateUI() method for the JList that you will be creating. The projectList will be constantly updated under the "Project creation" tab and when it is updated, this method should be called from the actionPerformed method in the ButtonListener of the CreatePanel class so that the JList under the "Project selection" will have the same update project list in them.
This class contains a nested class called ButtonListener class that implements ActionListener interface. Thus you need to define its actionPerformed method that is supposed to update the number of selected projects and display it when the "Add" or "Remove" button is pushed.
CreatePanel
CreatePanel extends JPanel defined in the javax.swing package. It should contain at least the following instance variable:
This class should have a constructor:
public CreatePanel(Vector projectList, SelectPanel selectPanel)
where the parameter "projectList" is passed from the Assignment6 class and the second parameter is an object of SelectPanel. The constructor layouts and organizes components in this panel. You will be adding more variables (components) than what is listed here, including labels, textfields, a button, and a text area.
This class contains a nested class called ButtonListener class that implements ActionListener interface. Thus the ButtonListener needs to have a definition for actionPerformed method that adds some information of a project to the list and does error handling. See the UML class diagram for the parameter and return type of this method. In the actionPerformed, you need to extract the information from the textfields. Then you can instantiate an object of the Project class using these values from the textfields. You can use the toString( ) method of the Project object to display the information on the textarea on the right hand side and also add the Project object to the "projectList".
Assignment6 class
Assignment6 class extends JApplet defined in javax.swing package. It contains at least init() method (see UML diagram for its return type) to instantiate all instance variables and adds its components to itself. It also sets its size. It contains at least following instance variables:
Step by Step Solution
There are 3 Steps involved in it
Step: 1
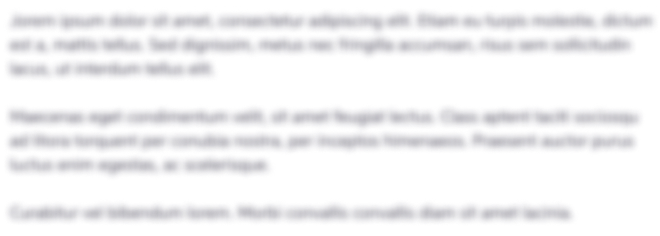
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started