Question
Guys I need help. I have a 4 class Java project that I am desperately trying to implement a menu 1-3 in my main that
Guys I need help. I have a 4 class Java project that I am desperately trying to implement a menu 1-3 in my main that looks something like this in the code below
1. Remove the Death Rate
2. Print the Queue
3. Exit
and have this menu loop until "3" is typed
It doesnt need to be 100% accurate as I can change all of that later, but I just need help figuring out how to put that menu into the code below
public class project3 {
public static void main(String[] args) throws IOException { System.out.println("What option would you like?"); System.out.println("1. Remove DR"); System.out.println("2. Print Queue"); System.out.println("3. Exit"); Scanner covid = new Scanner(System.in); System.out.println("Choose your option"); int choice = covid.nextInt(); final int sizes = 50; Priority poor = new Priority(sizes); Priority fair = new Priority(sizes); Priority good = new Priority(sizes); Priority vgood = new Priority(sizes); final int size = 50; Stack poor1 = new Stack(size); Stack fair1 = new Stack(size); Stack good1 = new Stack(size); Stack vgood1 = new Stack(size); /** * reads files in, found this path method much more consistent and easy to set up */ BufferedReader reader = new BufferedReader(new FileReader("C:\\Users\\apcg8\\eclipse-workspace\\Project2v2\\States2.csv")); String row = reader.readLine(); while ((row = reader.readLine()) != null) { String[] data = row.split(","); /** * Parses the data of the file */ String name = data[0]; String capital = data[1]; String region = data[2];
int seats = Integer.parseInt(data[3]); int population = Integer.parseInt(data[4]); int cases = Integer.parseInt(data[5]); int deaths = Integer.parseInt(data[6]); double CaseRate = ((double)cases / (double)population)* 100000; double DeathRate = ((double)deaths / (double)population)* 100000; double MHI = Double.parseDouble(data[7]); double rate = Double.parseDouble(data[8]); double cfr = (double) deaths / (double) cases; State states = new State(name, capital, region, seats, population, rate, cases, deaths, MHI, CaseRate, DeathRate, cfr); /** * Sets the limits of each group which defines where each state lands */ if(DeathRate<50) vgood.insertFront(states); else if(DeathRate<100) good.insertFront(states); else if(DeathRate<150) fair.insertFront(states); else poor.insertFront(states);
/** * Output data */ } System.out.print(" Priorty Queue Printed out"); System.out.println(" Poor: State MHI VCR CFR Case Rate Death Rate "); poor.printQueue(); System.out.println(" Fair: State MHI VCR CFR Case Rate Death Rate "); fair.printQueue(); System.out.println(" Good: State MHI VCR CFR Case Rate Death Rate "); good.printQueue(); System.out.println(" Very Good: State MHI VCR CFR Case Rate Death Rate "); vgood.printQueue(); /** * clear stacks */ Stack stack = new Stack(); while(!stack.isEmpty()) { stack.push(poor.removeFront()); } while(!fair.isEmpty()) { stack.push(fair.removeFront()); } while(!good.isEmpty()) { stack.push(good.removeFront()); } while(!vgood.isEmpty()) { stack.push(vgood.removeFront()); } System.out.print(" Stack Printed out"); System.out.println(" Poor: State MHI VCR CFR Case Rate Death Rate "); stack.printStack(); System.out.println(" Fair: State MHI VCR CFR Case Rate Death Rate "); stack.printStack(); System.out.println(" Good: State MHI VCR CFR Case Rate Death Rate "); stack.printStack(); System.out.println(" Very Good: State MHI VCR CFR Case Rate Death Rate "); stack.printStack(); reader.close(); } }//end
Step by Step Solution
There are 3 Steps involved in it
Step: 1
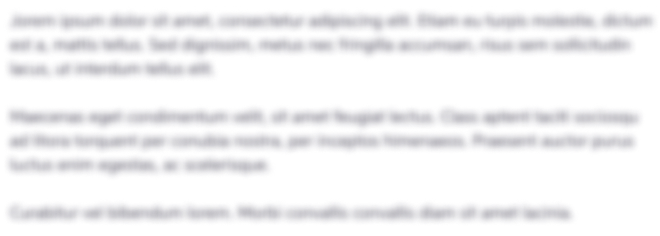
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started