Answered step by step
Verified Expert Solution
Question
1 Approved Answer
[Haskell] Complete the following Haskell function definitions. Unless stated otherwise do not use library functions that are not in the Haskell standard prelude module Project
[Haskell]
Complete the following Haskell function definitions. Unless stated otherwise do not use library functions that are not in the Haskell standard prelude
module Project where
data Tree a = Leaf a | Fork (Tree a) (Tree a) deriving (Show, Eq, Ord) data BST a = Tip | Bin (BST a) a (BST a) deriving (Show, Eq, Ord)
- height :: Tree a -> Int Find the longest path from the root to the leaf, counting only Fork nodes. That is, the height of Leaf 'x' is 0 and the height of Fork (Fork (Leaf 'a') (Leaf 'b')) (Leaf 'c') is 2. You may find the standard function max helpful. - minLeaf :: Ord a => Tree a -> a Return the minimum element in the tree; i.e., an item in a Leaf which is less than or equal to every other element. You may find the standard function min helpful. - inorder :: Tree a -> [a] Return a list containing the elements of the tree in left-to-right order. inorder (Fork (Fork (Leaf 5) (Leaf 1)) (Leaf 7)) should return [5,1,7] - contains :: Ord a => a -> BST a -> Bool Assuming that the tree is a binary search tree, determine whether the element is present in the tree. If t = Bin (Bin Tip 'a' Tip) 'b' Tip, then contains 'a' t == True and contains 'c' t == False. A BST is a binary search tree if, for every Bin l a r, all elements in l are less than or equal to a and all elements in r are greater than or equal to a. Your implementation should not examine every node in the tree. If the tree is not a true binary search tree, contains will be incorrect for some queries. So, if bad_tree = Bin (Bin Tip 'c' Tip) 'b', then contains 'c' bad_tree should be False. - insert :: Ord a => a -> BST a -> BST a Return a new BST containing the element and all the elements in the old BST. If the old BST is a binary search tree, the new BST must be as well. Note that a binary search tree never contains duplicate elements. If t = Bin (Bin Tip 'a' Tip) 'b' Tip, then insert 'c' t might be Bin (Bin Tip 'a' Tip) 'b' (Bin Tip 'c' Tip). You are not required to balance the tree. - delete :: Ord a => a -> BST a -> BST a Return a new BST containing all elements in the old BST that are not the specified element. If the old BST is a binary search tree, the new BST must be as well. If t = Bin (Bin Tip 'a' Tip) 'b' Tip, then delete 'b' t == Bin Tip 'a' Tip. You are not required to balance the tree.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
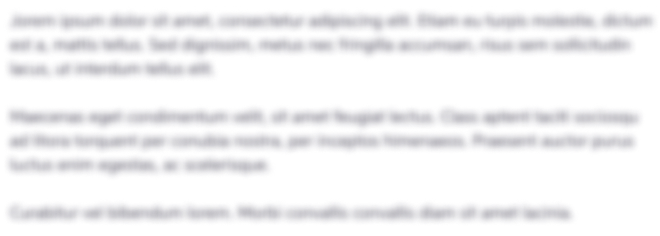
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started