Question
Have a program here that is for displaying a matrix, it is implemented using a list of lists. I believe there is a problem in
Have a program here that is for displaying a matrix, it is implemented using a list of lists. I believe there is a problem in my implementation of the set and get methods. Any help would be greatly appreciated :)
package matrix;
import java.util.List; import java.util.ArrayList;
/** * This class provides a data representation for the AbstractMatrix * implementation of the Matrix API. * It uses a list of list of integers to store matrix elements. */ public class ArrayListImplementation extends AbstractMatrix {
/** * Creates a list representation of a matrix of integers. * Elements of the list are initialized to zero. * @param numRows the number of rows in the matrix * @param numColumns the number of columns in the matrix * @throws MatrixException if dimensions are not positive */ public ArrayListImplementation(int numRows, int numColumns) { // You must provide super.setNumRows(numRows); super.setNumColumns(numColumns); elements = new ArrayList<>(numRows); for (int i=0; i < numRows; i++) { elements.add(new ArrayList
/** * Sets the element at the indicated row and column in this matrix. * * @param row the row position for the element. * @param column the column position for the element. * @param element the value to set in the matrix * @throws MatrixException if row or column is out of range */ @Override public void set(int row, int column, int element) { checkBounds(row, column); // simple helper for throwing the exception, this part is correct elements.get(row).set(column, element); this is the part I am confused on } // Private instance fields go here private List> elements; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
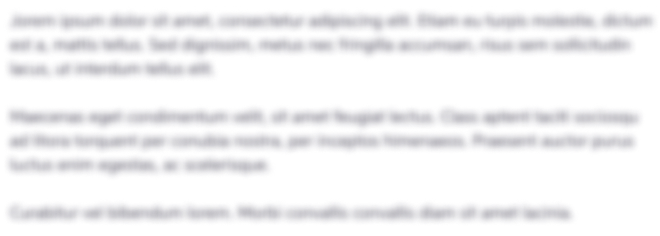
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started